Today we will learn how to check odd or even program in C.we will also learn Even and Odd program in c using different methods. Before start learning, we will make you know what is even number and odd number.
Table of Contents
What is Even Number?
The number is said to be even if and only if the number is divisible by 2.
For Example 2,4,6,8 and so on.
What is Odd Number?
The number is said to be Odd if and only if the number is not divisible by 2.
For Example 1,3,5,7 and so on.
Odd and even Number Algorithm
1 Step: START.
2 Step: Enter the Number to Check.
3 Step: if Number is divisible by 2 then the Number is Even.
4 Step: Else Number is Odd.
5 Step: Print the Output.
6 Step: STOP.
Odd and Even Program Flowchart:
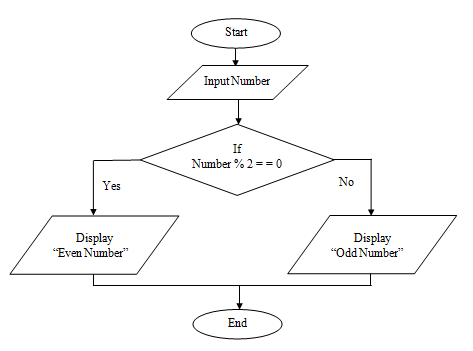
1. Odd or Even Program in C Using Modulus Operator
In this program, we will ask the user to enter the number which the user wants to check whether the number is odd or even. After that Compiler will check the number and display the output on the Screen.
#include <stdio.h> int main() { int n; printf("Enter a Number to Check Even or Odd\n"); scanf("%d", &n); if (n%2 == 0) printf("The Number is Even\n"); else printf("The Number is Odd\n"); return 0; }
Output:
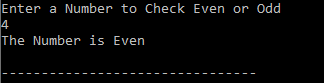
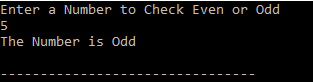
2. Odd or Even Program in C Using Conditional Operator
In this program we will use Conditional Operator to Check whether the given number is Odd or even.
Syntax of Conditional Statement:
Condition? 1st printf statement : 2nd printf statement.
The Condition statement returns TRUE if the condition is Correct and return FALSE if the condition is not Correct.
#include <stdio.h> int main() { int num; printf("Enter an integer: "); scanf("%d", &num); // conditional operator (num % 2 == 0) ? printf("%d is even.", num) : printf("%d is odd.", num); return 0; }
Output:


3. Using Bitwise Operator
In this program we will use bitwise operator to check whether the number is even or odd.
#include <stdio.h> int main() { int n; printf("Enter the number to check even or odd\n"); scanf("%d", &n); if (n & 1 == 1) printf("The number is Odd\n"); else printf("The number is Even\n"); return 0; }
Output:
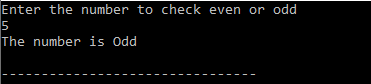
4. Even or Odd Program without Using Bitwise & Modulus Operator
Till now we use Bitwise and Modulus Operators.But in this program, we will not use this operators.
#include <stdio.h> int main() { int n; printf("Enter the number to check even or odd\n"); scanf("%d", &n); if ((n/2)*2 == n) printf("the number is Even\n"); else printf("the number is Odd\n"); return 0; }
Output:
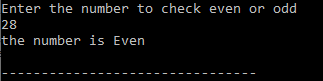
In C Language when we divide the two integers we get result as integer only.So we can use it to find whether the number is even or odd.
Even numbers are in the form 2*n and odd numbers are in the form (2*n+1) where n is an integer value.If we divide number by 2 and multiply it by 2 if the number is same then the number is even else the number is odd.
5. Program to print Even Numbers upto 100
In this program, we will print the even numbers upto 100.
#include<stdio.h> #include<conio.h> void main() { int n=2; while(n<=100) { printf(" %d",n); n=n+2; } }
Output:

Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Addition of Two Numbers in C
- Matrix Multiplication Program in C.
- Playfair Cipher Program in C.
- GCD Program in C.
- Tower of Hanoi in C.
- First Fit Program in C
- Swapping of two numbers in C
- Menu Driven Program in C.
- Round Robin Program in C