In this program, you will learn how to print Hello World program in C. The Beginning of Every language starts with the Hello World Program.
We will learn the different ways to print Hello world program in C.
Table of Contents
1. Hello World program in C
In this program we will print the “Hello World” on the screen.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>// Simple C program to display "Hello World" // Header file for input output functions #include <stdio.h> // main function - // where the execution of program begins int main() { // prints hello world printf("\n Hello World"); return 0; } </pre> </div>
Output:

Explanation:
#include<stdio.h>:
On this particular line of code, we now include a file called stdio.h. This document lets us use specific commands for output or input signal which we can utilize in our program. (Look at its lines of code commands) which were written for us by somebody else).
For example, it’s commanded for input like reading from the keyboard and output commands such as printing items on the monitor.
int main():
The int is what’s called the return value (in this instance of this type integer). Where it used will be explained further down. Every program has to have a main(). It’s the beginning point of each program.
The round brackets are there to get a Motive, in a subsequent tutorial it’ll be clarified, however, for now, it’s sufficient to understand they need to be there.
{ }:
Both curly brackets (one in the beginning and one at the end) are utilized to set all of the commands together. In this scenario, all the commands between the two curly brackets belong to main(). The curly brackets are frequently utilized from the C language to group commands together.
printf(“\n Hello World”);:
The printf can be used for printing items on the display, in this instance the words: Hello World. Since you may see the information that will be printed is placed inside mounts.
The word Hello World is interior inverted commas since they are what’s called a series. (Just one letter is called a character along with a succession of characters is called a series ).
Strings should always be placed between inverted commas. In this instance, it signifies a newline character. After printing something to the display you usually need to publish something on another line.
When there’s absolutely no \n then another printf command will print the series on precisely the exact same line. After the final round mount there has to be a semi-colon. The semi-colon proves that it’s the conclusion of the command. (So later on, do not forget to place a semi-colon in case a control stopped ).
return 0:
Once we wrote the first line”int main()”, we announced that main should return an integer int main(). (int is short for integer that is just another word for amount ).
Together with the control return 0; we could return the value to the working system. If you return with a zero you inform the operating system there were no errors while running this program. (Have a peek at the C tutorial — factors and constants to learn more on integer variables).
Compile:
In case you’ve typed everything right, there should be no difficulty to compile your program. After compilation, you should have a hello.exe (Windows) or hello binary (UNIX/Linux).
Now you can run this program by typing hello.exe (Windows) or. If that which was done right, you should see the words”Hello World” printed onto the monitor.
Comments:
The Hello World program is a little program that’s simple to comprehend. However, a program can contain tens of thousands of lines of code and may be so complicated it is difficult for us to comprehend.
To make our lives simpler it’s possible to compose an explanation or comment in a program. This makes it significantly easier to know the code.
comments can be defined by using ‘//’ or ‘*/ ….*/’.
2. Hello World Program Using Functions
In this program, we will use function printMessage to print the Hello World on the screen.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> // function to print Hello World! void printMessage(void) { printf("Hello World!"); } int main() { //calling function printMessage(); return 0; }</pre> </div>
Output:

In the above program, the function printMessage is called in main and we get the output Hello World on the screen.
3. Using Character variables
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { char a = 'H', b = 'e', c = 'l', d = 'o'; char e = 'w', f = 'r', g = 'd'; printf("%%c%%c%%c%%c%%c %%c%%c%%c%%c%%c", a, b, c, c, d, e, d, f, c, g); return 0; }</pre> </div>
Output:

In the above program, we have used seven-character variables a,b,c,d,e,f,g and %c is used to print the characters.
4. Print Hello world number of Times
In this program, we ask the user how many times he wants to print the Hello World. According to input, the program prints Hello World on the screen.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { int c, n; puts("How many times?"); scanf("%%d", &n); for (c = 1; c <= n; c++) puts("Hello world!"); return 0; }</pre> </div>
Output:
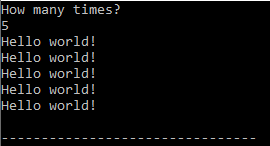
Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C