Today we will learn how to construct different number pattern programs in C. So before start learning pattern program in C, we will make a quick overview of the number pattern definition.
Table of Contents
What is Number Pattern Programs in C?
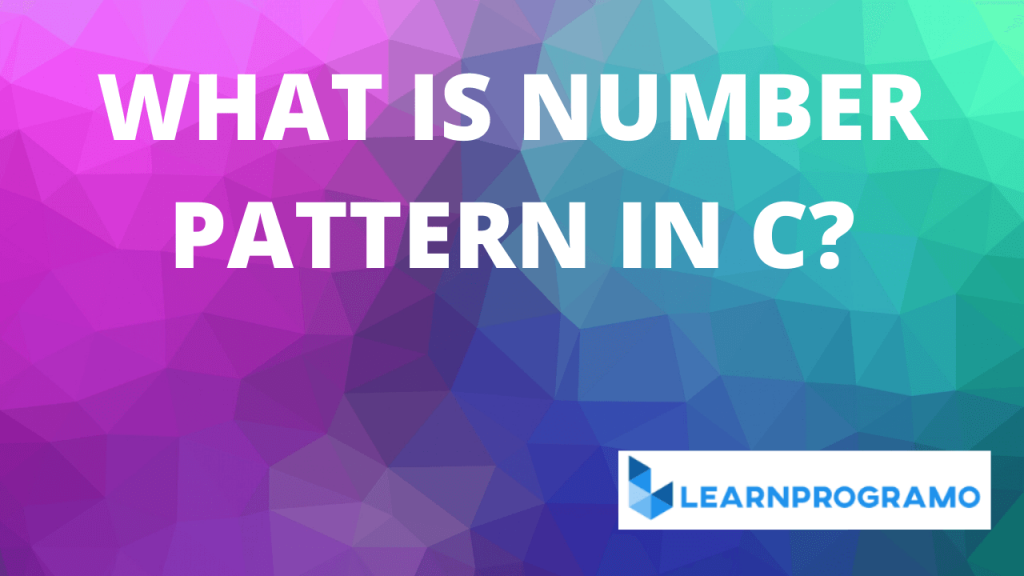
Basically the Number Pattern Programs in C is the series of numbers which are arranged in a particular order. These patterns are created by arranging the numbers which are similar to the star patterns in C.
For Example:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Every interview starts with pattern programs. So it is mandatory to learn the logic behind this number patterns. These patterns are best suited to increase your logical thinking ability.
We have structured these pattern programs in such a way that the beginners and the professionals can easily understand and practice with their own.
So after learning these programs, your duty is to understand the logic behind each pattern. Don’t go fast spend time to understand each program which will be beneficial for you.
So without wasting time, we will start learning number patterns one by one. If you have any doubt then please feel free to express your doubt in the comment section below.
Number Pattern Programs in C
Example #1
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for(i = 1; i <= n; i++) { for(j = 1; j <= i; j++) { printf("%d",j); } printf("\n"); } return 0; }
Example #2
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
6 6 6 6 6 6
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for(i = 1; i <= n; i++) { for(j = 1; j <= i; j++) { printf("%d",i); } printf("\n"); } return 0; }
Example #3
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for(i = n; i >= 1; i--) { for(j = 1; j <= i; j++) { printf("%d",j); } printf("\n"); } return 0; }
Example #4
1 1
1 2 2 1
1 2 3 3 2 1
1 2 3 4 4 3 2 1
1 2 3 4 5 5 4 3 2 1
1 2 3 4 5 6 6 5 4 3 2 1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j, k; printf("Enter the number of rows: "); scanf("%d",&n); for(i =1; i <= n; i++) { for(j =1; j <= n; j++) { if(j <= i) printf("%d",j); else printf(" "); } for(j = n; j >= 1;j--) { if(j <= i) printf("%d",j); else printf(" "); } printf("\n"); } return 0; }
Example #5
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
16 17 18 19 20 21
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j, c = 1; printf("Enter the number of rows: "); scanf("%d",&n); for(i = 1; i <= n; i++) { for(j = 1; j <= i; ++j) { printf("%d ", c); ++c; } printf("\n"); } return 0; }
Example #6
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for(int i = 1; i < n; i++) { for(int j = 1; j <= i; j++) printf("%d",j); printf("\n"); } for(int i = n; i >= 0; i--) { for(int j = 1; j <= i; j++) printf("%d",j); printf("\n"); } return 0; }
Example #7
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for (int i = n; i >= 0; i--) { for (int j = 1; j <= i; j++) printf("%d",j); printf("\n"); } for(int i = 1; i <= n; i++) { for(int j = 1; j <= i; j++) printf("%d",j); printf("\n"); } return 0; }
Example #8
1
0 1
1 0 1
0 1 0 1
1 0 1 0 1
0 1 0 1 0 1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j, x, y; printf("Enter the number of rows: "); scanf("%d",&n); for (i = 1; i <= n; i++) { if (i % 2 == 0) { x = 1; y = 0; } else { x = 0; y = 1; } for (j = 1; j <= i; j++) if (j % 2 == 0) printf("%d",x); else printf("%d",y); printf("\n"); } return 0; }
Example #9
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { int n, i, j; printf("Enter the number of rows: "); scanf("%d",&n); for(i = n; i >= 1; i--) { for(j = i; j >= 1; j--) { printf("%d", i); } printf("\n"); } return 0; }
Example #10
1 2 3 4 5
2 3 4 5
3 4 5
4 5
5
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 1;a < 6;a++) { for(i = a;i < 6;i++) { printf("%d", i); } printf("\n"); } return 0; }
Example #11
5 4 3 2 1
4 3 2 1
3 2 1
2 1
1
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 5;a >= 1;a--) { for(i = a;i >= 1;i--) { printf("%d", i); } printf("\n"); } return 0; }
Example #12
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 1;a < 6;a++) { for(i = 5;i >= a;i--) { printf("%d", i); } printf("\n"); } return 0; }
Example #13
5
4 5
3 4 5
2 3 4 5
1 2 3 4 5
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 5;a >= 1;a--) { for(i = a;i <= 5;i++) { printf("%d", i); } printf("\n"); } return 0; }
Example #14
5 5 5 5 5
4 5 5 5 5
3 4 5 5 5
2 3 4 5 5
1 2 3 4 5
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i, j; for(a = 5;a >= 1;a--) { for(i = a;i <= 5;i++) { printf("%d", i); } for(j = a-1;j >= 1;j--) { printf("5"); } printf("\n"); } return 0; }
Example #15
5
4 4
3 3 3
2 2 2 2
1 1 1 1 1
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 5; a >= 1; a--) { for(i = a;i <= 5;i++) { printf("%d", a); } printf("\n"); } return 0; }
Example #16
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i; for(a = 5;a >= 1;a--) { for(i = 1;i <= a;i++) { printf("%d", a); } printf("\n"); } return 0; }
Example #17
1
2 3
4 5 6
7 8 9 10
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i, k = 1; for(a = 1;a < 5;a++) { for(i = 1;i <= a;i++) { printf("%d ", k++); } printf("\n"); } return 0; }
Example #18
1
1 0
1 0 1
1 0 1 0
1 0 1 0 1
Program:
//Learnprogramo #include <stdio.h> int main() { int a, i, k; for(a = 1;a <= 5;a++) { for(i = 1;i <= a;i++) { printf("%d ", i%2); } printf("\n"); } return 0; }
Example #19
1
1 2 3
1 2 3 4 5
1 2 3 4 5 6 7
1 2 3 4 5
1 2 3
1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> void main() { int i, j; for(i=1;i<=7;i+=2) { for(j=1;j<=i;j++) printf("%d",j); printf("\n"); } for(i=5;i>=1;i-=2) { for(j=1;j<=i;j++) printf("%d",j); printf("\n"); } }
Example #20
1
0 0
1 1 1
0 0 0 0
1 1 1 1 1
Program:
//Learnprogramo #include<stdio.h> #include<conio.h> void main() { int i,j,n; printf("Enter no. of lines:\n"); scanf("%d",&n); for(i=1;i<=n;i++) { for(j=1;j<=i;j++) { if(i%2==1) { printf("1 "); } else { printf("0 "); } } printf("\n"); } }
Half Pyramid Of Numbers
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Program:
//Learnprogramo #include <stdio.h> int main() { int n; scanf("%d", &n); for (int i = 1; i <= n; ++i) { for (int j = 1; j <= i; ++j) { printf("%d ", j); } printf("\n"); } return 0; }
Inverted Half Pyramid Of Numbers
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Program:
//Learnprogramo #include <stdio.h> int main() { int n; scanf("%d", &n); for (int i = n; i >= 1; --i) { for (int j = 1; j <= i; ++j) { printf("%d ", j); } printf("\n"); } return 0; }
Full Pyramid Of Numbers
1
2 3 2
3 4 5 4 3
4 5 6 7 6 5 4
5 6 7 8 9 8 7 6 5
Program:
//Learnprogramo #include<stdio.h> int main() { int n; scanf("%d",&n); for (int i = 1; i <= n; i++) { for (int j = 1; j <= n - i; j++) { printf(" "); } for (int j = i; j < 2 * i; j++) { printf("%d ", j); } int ele = 2 * (i - 1); for (int j = 1; j <= i - 1; j++) { printf("%d ", ele--); } printf("\n"); } return 0; }
Pascals Triangle
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Program:
#include<stdio.h> int main() { int n; scanf("%d",&n); for (int i = 1; i <= n; i++) { for(int j = 1; j <= n-i; j++) { printf(" "); } int C = 1; for (int j = 1; j <= i; j++) { printf("%d ", C); C = C * (i - j) / j; } printf("\n"); } return 0; }
Floyd’s Triangle
1
2 3
4 5 6
7 8 9 10
Program:
//Learnprogramo #include <stdio.h> int main() { int n; scanf("%d", &n); int num = 1; for (int i = 1; i <= n; i++) { for (int j = 1; j <= i; ++j) { printf("%d ", num); ++num; } printf("\n"); } return 0; }
Conclusion:
So this is the pattern programs in C which are widely used in C and interviews. If you learn all the above pattern then your base of programming will become perfect. Hope you understand all the programs have a nice day…
If you have any problems or doubt then please mention in the below comment box.
Also Read:
- 20 Different Star Pattern Programs in C.
- 20 Different Number Pattern programs in C.
- Anagram Program in Java.
- How to add Tomcat Server in Eclipse.