Today we will learn the Fibonacci Series Program in C and also Fibonacci Series in C Program using different loops and user-defined functions. But, before starting you must have knowledge of Fibonacci Series. So, we will learn it first.
Table of Contents
What is Fibonacci Series?
The Fibonacci Series is a never-ending Sequence where the next upcoming term is the addition of the previous two terms. The Fibonacci Series starts with 0,1…
Fibonacci Series: 0,1,1,2,3,5,8,13,21 and so on.
In the above example, you will observe that the series is starting with 0,1 and the third number is 1 because of the addition of the first two numbers.
Fibonacci Series Algorithm:
1 Step: Take an integer variable X, Y and Z
2 Step: Set X=0 and Y=0.
3 Step: Display X and Y.
4 Step: Z=X+Y.
5 Step: Display Z.
6 Step: Start X=Y, Y=Z.
7 Step: Repeat from 4-6, for n times.
There are different methods to print Fibonacci Series we will see that methods one by one.
1. Fibonacci Sequence up to Certain Number
In this program, we will ask the user to enter the number up to which the user wants to print the series.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { int Number, i = 0, Next, First_Value = 0, Second_Value = 1; printf("\n Please Enter the Range Number: "); scanf("%%d",&Number); while(i < Number) { if(i <= 1) { Next = i; } else { Next = First_Value + Second_Value; First_Value = Second_Value; Second_Value = Next; } printf("%%d \t", Next); i++; } return 0; }</pre> </div>
Output:
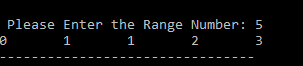
In the above program, we have declared four integer variables Number, i, First_Value, Second_Value.
Explanation:
1st Iteration: the while condition(0<5) returns TRUE so the while statement will start executing. We have used the if-else statement and condition if(0<=1) returns TRUE. So, Next=0 and then if statement block will exit. At last, i will increment.
2nd Iteration: the while condition(1<5) returns TRUE so the while statement will start executing. The condition if(1<=1) returns TRUE. So, Next=1 and then if statement block will exit. At last, i will increment to 1.
3rd Iteration: the while condition(2<5) returns TRUE and if(2<=1) returns FALSE so else statement will start executing. Next=First_Value+Second_Value=0+1=1 and the First_Value=Second_Value=1. So, Second_Value=Next=1 and i is incremented by 1.
4th Iteration: the while condition(3<5) returns TRUE and if(3<=1) returns FALSE so else statement will start executing. Next=First_Value+Second_Value=1+1=2 and the First_Value=Second_Value=1. So, Second_Value=Next=2 and i is incremented to 4.
5th Iteration: the while condition(4<5) returns TRUE and if(4<=1) returns FALSE so else statement will start executing. Next=First_Value+Second_Value=1+2=3 and the First_Value=Second_Value=2. So, Second_Value=Next=3 and i is incremented to 5.
6th Iteration: the while condition(5<5) returns FALSE and the program is exited. And the final output is 0 1 1 2 3.
2. Fibonacci Series up to n Times
This program will print Fibonacci terms up to n times.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { int i, n, t1 = 0, t2 = 1, nextTerm; printf("Enter the number of terms: "); scanf("%%d", &n); printf("Fibonacci Series: "); for (i = 1; i <= n; ++i) { printf("%%d, ", t1); nextTerm = t1 + t2; t1 = t2; t2 = nextTerm; } return 0; }</pre> </div>
Output:

3. Fibonacci Series in C without Recursion
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include<stdio.h> int main() { int n1=0,n2=1,n3,i,number; printf("Enter the number of elements:"); scanf("%%d",&number); printf("\n%%d %%d",n1,n2);//printing 0 and 1 for(i=2;i<number;++i) //loop starts from 2 because 0 and 1 are already printed { n3=n1+n2; printf(" %%d",n3); n1=n2; n2=n3; } return 0; } </pre> </div>
Output:
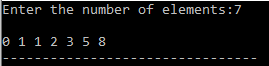
4. Fibonacci Series program Using Recursion
Recursion means calling the function again and again until the condition becomes false.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre> #include<stdio.h> void printFibonacci(int n){ static int n1=0,n2=1,n3; if(n>0){ n3 = n1 + n2; n1 = n2; n2 = n3; printf("%%d ",n3); printFibonacci(n-1); } } int main(){ int n; printf("Enter the number of elements: "); scanf("%%d",&n); printf("Fibonacci Series: "); printf("%%d %%d ",0,1); printFibonacci(n-2);//n-2 because 2 numbers are already printed return 0; } </pre> </div>
Output:

Time Complexity: T(n)=T(n-1)+T(n-2) i.e exponential.
In the above program, we can observe that the implementation does lots of repetition so this is very bad implementation for nth Fibonacci Series.
5. Using for Loop
In this program, we will use for loop instead of while loop doesn’t be afraid the logic is the same.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { int Number, Next, i, First_Value = 0, Second_Value = 1; printf("\n Please Enter the Range Number: "); scanf("%%d",&Number); /* Find & Displaying Fibonacci series */ for(i = 0; i <= Number; i++) { if(i <= 1) { Next = i; } else { Next = First_Value + Second_Value; First_Value = Second_Value; Second_Value = Next; } printf("%%d \t", Next); } return 0; }</pre> </div>
Output:
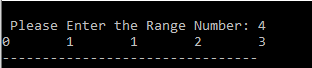
6. Using dynamic Programming
we can store the Fibonacci number to avoid repetition.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int fib(int n) { /* Declare an array to store Fibonacci numbers. */ int f[n + 1]; int i; /* 0th and 1st number of the series are 0 and 1*/ f[0] = 0; f[1] = 1; for (i = 2; i <= n; i++) { /* Add the previous 2 numbers in the series and store it */ f[i] = f[i - 1] + f[i - 2]; } return f[n]; } int main() { int n = 9; printf("%%d", fib(n)); getchar(); return 0; } </pre> </div>
Output:

7. Fibonacci Series in C program Using Functions
In this program, we will use a user-defined function Fibonacci_Series to print a Fibonacci Series Up to n number.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include<stdio.h> int Fibonacci_Series(int); int main() { int Number, i = 0, j; printf("\n Please Enter Number upto which you want too: "); scanf("%%d", &Number); printf("Fibonacci series\n"); for ( j = 0 ; j <= Number ; j++ ) { printf("%%d\t", Fibonacci_Series(j)); } return 0; } int Fibonacci_Series(int Number) { if ( Number == 0 ) return 0; else if ( Number == 1 ) return 1; else return ( Fibonacci_Series(Number - 1) + Fibonacci_Series(Number - 2) ); }</pre> </div>
Output:

Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Fibonacci Series in Python