Today we will learn a C program to Reverse a Number and Program to reverse a Number in C using different loops and methods.
First of all, what is Reverse of a Number?. Reversing a number means changing all the positions of the digit of a number.
For Example 12345 and it’s reverse is 54321.
There are many methods to reverse a number in c we will see it one by one.
Table of Contents
1. Reversing a Number Using While loop
In this program, we will ask the user to enter the number which user want to reverse. The program will reverse the number using While loop.
#include <stdio.h> int main() { int n, rev = 0, remainder; printf("Enter an integer: "); scanf("%d", &n); while (n != 0) { remainder = n % 10; rev = rev * 10 + remainder; n /= 10; } printf("Reversed number = %d", rev); return 0; }
Output:

Logic:
Let us consider user enter the number 1456. First the value of rev is 0.
1st Iteration: remainder=n%10 i.e remainder=1456%10=6 and rev=rev*10+remainder i.e rev=0*10+6=0+6=6.So, n=n/10 i.e 1456/10=145.
2nd Iteration: from the first iteration the value of n=145 and rev=6. remainder=n%10 i.e remainder=145%10=5 and rev=rev*10+remainder i.e rev=6*10+5=60+5=65. So, n=n/10 i.e 145/10=14.
3rd Iteration: from the second iteration the value of n=14 and reverse=65. remainder=n%10 i.e remainder=14%10=4 and the rev=rev*10+remainder i.e rev=65*10+4=650+4=654. So, n=n/10=14/10=1.
4th Iteration: from the third iteration the value of n=1 and rev=654. remainder=n%10 i.e remainder=1%10=1 and rev=rev*10+remainder i.e rev=654*10+1=6540+1=6541. So,n=n/10 i.e n=1/10=0
Now the value of n is 0 so the while loop will exit.
2. Using for Loop
#include<stdio.h> void main() { int no,rev=0,r,a; printf("Enter any num: "); scanf("%d",&no); a=no; for(;no>0;) { r=no%10; rev=rev*10+r; no=no/10; } printf("Reverse of %d is %d",a,rev); }
Output:

In the above program, we have a user for loop instead of while loop so doesn’t be hesitated the logic behind the program is same.
3. C program to reverse a number using function
In this program, we will use the function in the main method so that the user will get the reverse of the input number after executing.
#include <stdio.h> int Reverse_Integer (int); int main() { int Number, Reverse = 0; printf("\nPlease Enter any number to Reverse\n"); scanf("%d", &Number); Reverse = Reverse_Integer (Number); printf("Reverse of entered number is = %d\n", Reverse); return 0; } int Reverse_Integer (int Number) { int Reminder, Reverse = 0; while (Number > 0) { Reminder = Number %10; Reverse = Reverse *10+ Reminder; Number = Number /10; } return Reverse; }
When the compiler reaches to Reverse_Integer(Number) then the compiler immediately jump to the Reverse_Integer function to perform its operation.
The logic behind the program is already discussed in the while loop program.
4. Using Recursion
Recursion means the function calls itself again and again till the condition becomes false.
#include<stdio.h> int main(){ int num,reverse_number; //User would input the number printf("\nEnter any number:"); scanf("%d",&num); //Calling user defined function to perform reverse reverse_number=reverse_function(num); printf("\nAfter reverse the no is :%d",reverse_number); return 0; } int sum=0,rem; reverse_function(int num){ if(num){ rem=num%10; sum=sum*10+rem; reverse_function(num/10); } else return sum; return sum; }
Output:
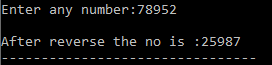
In the above program, we have defined the user-defined function reverse_function which is called recursively.
Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C