Today we will learn C program to reverse a string and also how to write a c program without using string function.
Table of Contents
What is reversing a String?
Reversing a string means changing the positions of the characters in such a way that the last character comes in the first position, second last on the second position and so on.
There are so many ways to reverse a string we will see it one by one.
1. Using inbuilt function strrev()
In this program, we will use inbuilt library function called as strrev() which reverses the string and print that string on the screen.
#include <stdio.h> #include <string.h> int main() { char s[100]; printf("Enter a string to reverse\n"); gets(s); strrev(s); printf("Reverse of the string: %s\n", s); return 0; }
Output:
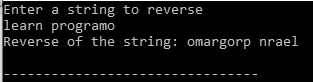
2. Reversing a string without using a function (using for loop)
The first program was very simple because we use library function for reversing a string. But how to reverse a string without using the function.
In this program, you will learn how to reverse a string without using a function.
#include <stdio.h> #include <string.h> int main() { char Str[100], RevStr[100]; int i, j, len; printf("\n Please Enter any String : "); gets(Str); j = 0; len = strlen(Str); for (i = len - 1; i >= 0; i--) { RevStr[j++] = Str[i]; } RevStr[i] = '\0'; printf("\n String after Reversing = %s", RevStr); return 0; }
Output:
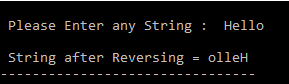
Explanation:
In the above output, you can observe that str[]=Hello and at the initial stage the value of i is 0 i.e i=0. So, len=strlen(Str)=5.Here strlen is the inbuilt function to find the length of the string i.e 5.
1st Iteration: for(i=len-1;i>=0;i–) i.e for(i=5-1;4>=0;4–).Here the condition (4>=0) is true.Therefore, RevStr[j++]=Str[i] i.e RevStr[0]=Str[4]=o.
2nd Iteration: for(i=len-1;i>=0;i–) i.e for(i=4-1;3>=0;3–).Here the condition (3>=0) is true.Therefore, RevStr[j++]=Str[i] i.e RevStr[1]=Str[3]=l.
3rd Iteration: for(i=len-1;i>=0;i–) i.e for(i=3-1;2>=0;2–).Here the condition (2>=0) is true.Therefore, RevStr[j++]=Str[i] i.e RevStr[2]=Str[2]=l.
4th Iteration: for(i=len-1;i>=0;i–) i.e for(i=2-1;1>=0;1–).Here the condition (1>=0) is true.Therefore, RevStr[j++]=Str[i] i.e RevStr[3]=Str[1]=e.
5th Iteration: for(i=len-1;i>=0;i–) i.e for(i=1-1;0>=0;0–).Here the condition (0>=0) is true.Therefore, RevStr[j++]=Str[i] i.e RevStr[4]=Str[0]=H.
6th Iteration: now the for loop will stop executing and the program will exit.
3. Without storing in a separate array
In the above program, we were storing the reverse string in a separate array. In this program, we will not store the reverse string in a separate array.
#include <stdio.h> #include <string.h> int main() { char Str[100]; int i, len; printf("\n Please Enter String : "); gets(Str); len = strlen(Str); printf("\n Reverse string is : "); for (i = len - 1; i >= 0; i--) { printf("%c", Str[i]); } return 0; }
Output:
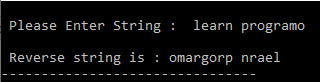
4. Reversing a string with the concept of Swapping
In this program, we will use the concept of swapping. The temporary variable temp is declared in the program.
#include <stdio.h> #include <string.h> int main() { char Str[100], temp; int i, j, len; printf("\n Please Enter any String : "); gets(Str); len = strlen(Str) - 1; for (i = 0; i < strlen(Str)/2; i++) { temp = Str[i]; Str[i] = Str[len]; Str[len--] = temp; } printf("\n String after Reversing = %s", Str); return 0; }
Output:
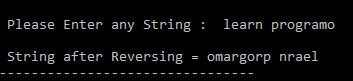
5. C program to reverse a string using the function
In this program, we will declare the user-defined function to reverse a string.
#include <stdio.h> #include <string.h> void reverse_String(char [], int, int); int main() { char Str[100], temp; int i, j, len; printf("\n Please Enter any String : "); gets(Str); len = strlen(Str); reverse_String(Str, 0, len -1); printf("\n String after Reversing = %s", Str); return 0; } void reverse_String(char Str[], int i, int len) { char temp; temp = Str[i]; Str[i] = Str[len - i]; Str[len - i] = temp; if (i == len/2) { return; } reverse_String(Str, i + 1, len); }
Output:
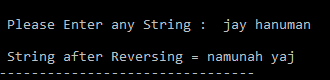
In the above program the user-defined function is reverse_String.
6. C Program to Reverse a String using Recursion
Recursion means calling a function again and again till the condition get false.
#include<stdio.h> char* reverse(char* str); // declaring recursive function void main() { int i, j, k; char str[100]; char *rev; printf("Enter the string:"); gets(str); printf("The original string is:%s ",str); rev = reverse(str); printf("\n The reversed string is: "); puts(rev); } char* reverse(char *str) { // defining the function static int i = 0; static char rev[100]; if(*str) { reverse(str+1); rev[i++] = *str; } return rev; }
Output:
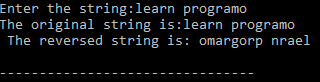
7. C program to reverse a String Using Pointers
In this program, we will reverse the string with the help of pointers.
#include <stdio.h> #include <string.h> char* reverse_String(char *Str) { static int i = 0; static char RevStr[10]; if(*Str) { reverse_String(Str + 1); RevStr[i++] = *Str; } return RevStr; } int main() { char Str[100], temp; int i, j, len; printf("\n Please Enter any String : "); gets(Str); printf("\n String after Reversing = %s", reverse_String(Str)); return 0; }
Output:
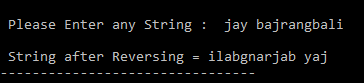
Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C