Today we will learn the Armstrong Number Program in C and also how to find Armstrong number in C program. But, before start learning, you should have a little bit knowledge about Armstrong number.So,
Table of Contents
What is an Armstrong Number?
A positive number is called as Armstrong of order n if abc=a^n+b^n+c^n. The number is called an Armstrong number if the sum of the cubes of each digit is equal to the number itself.
For Example:
153 is an Armstrong number because of 1*1*1+5*5*5+3*3*3=153 which is equal to the number itself. Now we will take another example of 1253 =1*1*1+2*2*2+5*5*5+3*3*3=1253 which is equal to number i.e 1253.
Armstrong Number Algorithm:
1st Step: Take an Integer value from the user.
2nd Step: Now assign that value to the variable.
3rd Step: Now split all the digits.
4th Step: Find the cube value of each digit.
5th Step: Add all the value of the cubes.
6th Step: now store that value in a variable.
7th Step: if the value of addition is equal to input number then print Armstrong.
8th Step: else print Not an Armstrong number.
There are so many methods to check whether the number is Armstrong or not we will see the methods one by one.
1. Armstrong Number Program in C Using While loop
In this program, we will ask the user to enter the number which the user wants to check whether the number is Armstrong or not. The program will check using while loop.
#include <stdio.h> #include <math.h> int main() { int Number, Temp, Reminder, Times =0, Sum = 0; printf("\nPlease Enter number to Check for Armstrong \n"); scanf("%d", &Number); //Helps to prevent altering the original value Temp = Number; while (Temp != 0) { Times = Times + 1; Temp = Temp / 10; } Temp = Number; while( Temp > 0) { Reminder = Temp %10; Sum = Sum + pow(Reminder, Times); Temp = Temp /10; } printf("\n Sum of entered number is = %d\n", Sum); if ( Number == Sum ) printf("\n %d is Armstrong Number.\n", Number); else printf("\n %d is not a Armstrong Number.\n", Number); return 0; }
Output:
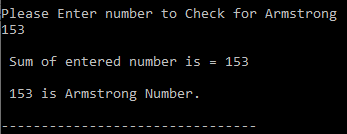
Explanation:
If you didn’t understand the logic behind the program then read this explanation carefully.
To understand this program we will take another example of 1634.At first the value of sum=0,Temp=Number i.e Temp=1634.
1st Iteration: Remainder=Temp%10 i.e remainder=1634%10=4.Now, Sum=Sum+(Remainder*Remainder*Remainder*Remainder) i.e Sum=0+(4*4*4*4)=0+256=256 and Temp=Temp/10 i.e 1634/10=163.
2nd Iteration: From the first iteration the value of Temp and Sum is replaced i.e Temp=163 and Sum=256. Remainder=temp%10 i.e remainder=163%10=3 and Sum=256+(3*3*3*3) i.e Sum=256+81=337.So,Temp=Temp/10 i.e 163/10=16.
3rd Iteration: From the second iteration the value of Temp and Sum is replaced i.e Temp=16 and Sum=337. Remainder=temp%10 i.e remainder=16%10=6 and Sum=337+(6*6*6*6) i.e Sum=337+1296=1633.So,Temp=Temp/10 i.e 16/10=1.
4th Iteration: From the third iteration the value of Temp and Sum is replaced i.e Temp=1 and Sum=1633. Remainder=temp%10 i.e remainder=1%10=0 and Sum=1633+(1*1*1*1) i.e Sum=1633+1=1634.So,Temp=Temp/10 i.e 1/10=0.
5th Iteration: Now the value of Number=0 so the while loop will fail.if(Number==Sum) i.e if(1634==1634) is TRUE then the number is Armstrong else, not Armstrong.
2. Check Armstrong of three-digit Number
#include <stdio.h> int main() { int num, originalNum, remainder, result = 0; printf("Enter a three-digit integer: "); scanf("%d", &num); originalNum = num; while (originalNum != 0) { // remainder contains the last digit remainder = originalNum % 10; result += remainder * remainder * remainder; // removing last digit from the orignal number originalNum /= 10; } if (result == num) printf("%d is an Armstrong number.", num); else printf("%d is not an Armstrong number.", num); return 0; }
Output:

3. Armstrong Number Program in C Using for Loop
In this program, we will use for loop instead of while loop but remember the logic behind the program is same.
#include <stdio.h> #include <math.h> int main() { int Number, Temp, Reminder, Times =0, Sum = 0; printf("\nPlease Enter any number to Check for Armstrong \n"); scanf("%d", &Number); Temp = Number; while (Temp != 0) { Times = Times + 1; Temp = Temp / 10; } for(Temp = Number; Temp > 0; Temp = Temp /10 ) { Reminder = Temp % 10; Sum = Sum + pow(Reminder, Times); } printf("\nSum of entered number is = %d\n", Sum); if ( Number == Sum ) printf("\n%d is Armstrong Number.\n", Number); else printf("%d is not the Armstrong Number.\n", Number); return 0; }
Output:
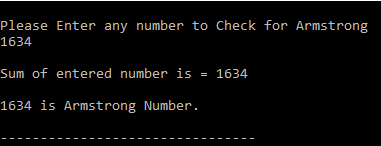
4. Armstrong Number Using Function
In this program, we will use a user-defined function to check whether the number is Armstrong or not.
#include <stdio.h> #include <math.h> int armstrongNumberFinder(int n); int main() { int n, flag; printf("Enter a positive integer: "); scanf("%d", &n); flag = armstrongNumberFinder(n); if (flag == 1) printf("%d is an Armstrong number.", n); else printf("%d is not an Armstrong number.",n); return 0; } int armstrongNumberFinder(int num) { int original, rem, sum = 0, n = 0, flag; original = num; while(original != 0) { original /= 10; ++n; } original = num; while(original != 0) { rem = original%10; sum += pow(rem, n); original /= 10; } if(sum == num) flag = 1; else flag = 0; return flag; }
Output:


In the above program, we have used function armstrongNumberFinder to check number is Armstrong or not. When the compiler reaches armstrongNumberfinder(n) then compiler directly jumps to the function to do further operations.
Armstrong Number in C Program Using Recursion
Recursion is the process which occurs when a function calls a copy of itself to work on the smaller problems.
#include <stdio.h> #include <math.h> int Check_Armstrong (int, int); int main() { int Number, Sum = 0, Times =0,Temp; printf("\nPlease Enter number to Check for Armstrong \n"); scanf("%d", &Number); Temp = Number; while (Temp != 0) { Times = Times + 1; Temp = Temp / 10; } Sum = Check_Armstrong (Number, Times); printf("Sum of entered number is = %d\n", Sum); if ( Number == Sum ) printf("\n%d is Armstrong Number.\n", Number); else printf("%d is not the Armstrong Number.\n", Number); return 0; } int Check_Armstrong (int Number, int Times) { static int Reminder, Sum = 0; if (Number > 0) { Reminder = Number %10; Sum = Sum + pow(Reminder, Times); Check_Armstrong (Number /10, Times); return Sum; } else return 0; }
Output:
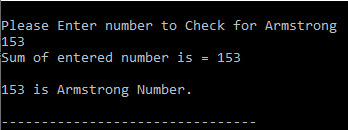
Armstrong_Check(Number/10); this statement is used to call the function Recursively. If you miss the statement the program will terminate after executing the first line.
Armstrong Number Program to Print Between 1 to n
After compiling this program, the compiler will ask to enter a minimum and the maximum number and then the compiler will start executing and prints the Armstrong number between minimum and maximum.
#include<stdio.h> #include <math.h> int Check_Armstrong (int); int main() { int Number,Reminder,Reverse,Temp, Sum; int Minimum,Maximum; printf("\nPlease Enter the Minimum & Maximum Values\n"); scanf("%d %d",&Minimum, &Maximum); printf("Armstrong Numbers Between %d and %d are:\n",Minimum, Maximum); for(Number = Minimum; Number <= Maximum; Number++) { Sum = Check_Armstrong (Number); if(Number == Sum) printf("%d ",Number); } return 0; } int Check_Armstrong (int Number) { int Temp, Reminder, Times =0, Sum = 0; Temp = Number; while (Temp != 0) { Times = Times + 1; Temp = Temp / 10; } for(Temp = Number; Temp > 0; Temp = Temp /10 ) { Reminder = Temp %10; Sum = Sum + pow(Reminder, Times); } return Sum; }
Output:
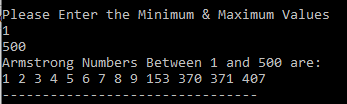
in the above program, we have entered the minimum value is 1 and the maximum value is 500. So, the program prints Armstrong numbers between 1 and 500.
Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C