This is one of the Simplest Methods for Memory Allocation. There, the main motive is to divide the memory into several fixed Sizes. Each partition of First Fit Program in C contains exactly one process.
There are different Memory Management Schemes in the operating System named as First Fit, Worst Fit, Best Fit. But today we will learn First Fit Program in C so before start learning we should have knowledge about First-Fit.
Table of Contents
What is First Fit?
The First Fit memory allocation checks the empty memory blocks in a sequential manner. It means that the memory Block which found empty in the first attempt is checked for size.
But if the size is not less than the required size then it is allocated.
Advantage:
It is the fastest searching Algorithm as we not to search only first block, we do not need to search a lot.
Disadvantage:
The main disadvantage of First Fit is that the extra space cannot be used by any other processes.If the memory is allocated it creates large amount of chunks of memory space.
First Fit Memory Management Scheme:
In the First Fit Memory Management Scheme, we check the block in the sequential manner i.e we take the first process and compare its size with the first block.
If the size is less than the size of the first block then only it is allocated. Otherwise, we move to the second block and this process is continuously going on until all processes are allocated.
Algorithm of First Fit:
1 Step: START.
2 Step: At first get the no of processes and blocks.
3 Step: Allocate the process by if(size of block>=size of the process) then allocate the process else move to the next block.
4 Step: Now Display the processes with blocks and allocate to respective process.
5 Step: STOP.
We have discussed the working and the Algorithm. Now, we will see the program for First Fit in C.
1. First Fit Program in C
#include<stdio.h> void main() { int bsize[10], psize[10], bno, pno, flags[10], allocation[10], i, j; for(i = 0; i < 10; i++) { flags[i] = 0; allocation[i] = -1; } printf("Enter no. of blocks: "); scanf("%d", &bno); printf("\nEnter size of each block: "); for(i = 0; i < bno; i++) scanf("%d", &bsize[i]); printf("\nEnter no. of processes: "); scanf("%d", &pno); printf("\nEnter size of each process: "); for(i = 0; i < pno; i++) scanf("%d", &psize[i]); for(i = 0; i < pno; i++) //allocation as per first fit for(j = 0; j < bno; j++) if(flags[j] == 0 && bsize[j] >= psize[i]) { allocation[j] = i; flags[j] = 1; break; } //display allocation details printf("\nBlock no.\tsize\t\tprocess no.\t\tsize"); for(i = 0; i < bno; i++) { printf("\n%d\t\t%d\t\t", i+1, bsize[i]); if(flags[i] == 1) printf("%d\t\t\t%d",allocation[i]+1,psize[allocation[i]]); else printf("Not allocated"); } }
Output:
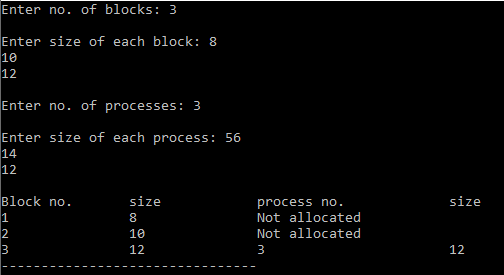
2. First Fit Program in C++
#include<iostream> using namespace std; int main() { int bsize[10], psize[10], bno, pno, flags[10], allocation[10], i, j; for(i = 0; i < 10; i++) { flags[i] = 0; allocation[i] = -1; } cout<<"Enter no. of blocks: "; cin>>bno; cout<<"\nEnter size of each block: "; for(i = 0; i < bno; i++) cin>>bsize[i]; cout<<"\nEnter no. of processes: "; cin>>pno; cout<<"\nEnter size of each process: "; for(i = 0; i < pno; i++) cin>>psize[i]; for(i = 0; i < pno; i++) //allocation as per first fit for(j = 0; j < bno; j++) if(flags[j] == 0 && bsize[j] >= psize[i]) { allocation[j] = i; flags[j] = 1; break; } //display allocation details cout<<"\nBlock no.\tsize\t\tprocess no.\t\tsize"; for(i = 0; i < bno; i++) { cout<<"\n"<< i+1<<"\t\t"<<bsize[i]<<"\t\t"; if(flags[i] == 1) cout<<allocation[i]+1<<"\t\t\t"<<psize[allocation[i]]; else cout<<"Not allocated"; } return 0; }
Output:
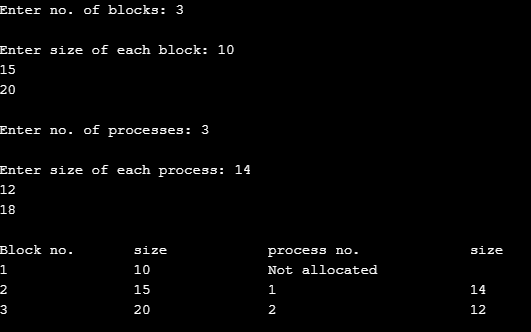
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Addition of Two Numbers in C
- Matrix Multiplication Program in C.
- Playfair Cipher Program in C.
- GCD Program in C.
- Tower of Hanoi in C.
- Swapping of two numbers in C