Today we will learn the Anagram program in c and also learn the Anagram program in C using String. But before starting you should have a little bit of knowledge about Anagram. So,
Table of Contents
What is Anagram?
The two strings are called as Anagram of each other if they contain the same characters either arranged in sequence or not it is not necessary.
How to check two Strings are Anagram or not?
To check whether the given two strings are Anagram of each other or not the compiler will ask the user to enter the two strings to check. After the input given by the user, the program will start executing are check whether the strings are Anagram or not.
After executing the compiler will display the output. The two strings are Anagram if and only if characters in that strings are the same sequence is not mandatory.
For Example abcd and dcba, creative and reactive, course and source are Anagram of each other.
Anagram Algorithm:
1 Step: Declare two Strings.
2 Step: Find out the length of two Strings (Strings are not anagram if the length of strings is not the same).
3 Step: Even if the lengths are equal the two strings should be in lowercase because it makes easy to check.
4 Step: Now sort the characters in the strings.For, sorting convert the strings into a character array.
5 Step: After converting character array must be sorted.
6 Step: In the last step, the Anagram is checked.
There are different ways to check the Anagram program in C we will see it one by one.
1. Anagram Program in C Using Sorting
In this program, we have declared two strings abcd and cabd. Now, the program will check whether the two Strings are Anagram or not.
#include <stdio.h> #include <string.h> //Declare the function names that are defined in the program later void converttoLowercase(char[]); void Arraysort(char[]); int main () { char string1[] = "abcd", string2[] = "cabd"; int a1, b = 0; //length of strings is compared if(strlen(string1) != strlen(string2)) { printf("Both the strings are not anagram"); return 0; } else { //the strings are converted to lowercase converttoLowercase(string1); converttoLowercase(string2); //The arrays are sorted by calling the function Arraysort() Arraysort(string1); Arraysort(string2); for(a1 = 0; a1 < strlen(string1); a1++) { if(string1[a1] != string2[a1]) { printf("Both the strings are not anagram"); return 0; } } printf("Both the strings are anagram"); } return 0; } void converttoLowercase(char a[]) { int c; for(c = 0; c < strlen(a)-1; c++) { a[c] = a[c]+32; } } void Arraysort(char a[]) { int temperory = 0,k,l; for(k = 0; k < strlen(a)-1; k++) { for (l = k+1; l < strlen(a); l++) { if(a[k] > a[l]) { temperory = a[k]; a[k] = a[l]; a[l] = temperory; } } } }
Output:

2. Anagram program in C Using Nested For Loop
In this program, we will not declare strings instead we will ask the user to enter a first and second string. If the length of strings is not the same then the program will exit so length should be the same.
#include<stdio.h> #include<conio.h> #include<string.h> int main() { char string1[20], string2[20]; int leng, leng1, leng2, a, b, found1=0, not_found1=0; printf("Enter First String: "); gets(string1); printf("Enter Second String: "); gets(string2); //length of the first string is calculated leng1 = strlen(string1); //length of the first string is calculated leng2 = strlen(string2); //compare the length of the two strings to find out if the strings are anagram or not if(leng1 == leng2) { leng = leng1; for(a=0; a<leng; a++) { found1 = 0; for(b=0; b<leng; b++) { if(string1[a] == string2[b]) { found1 = 1; break; } } if(found1 == 0) { not_found1 = 1; break; } } if(not_found1 == 1) printf("\nThe two entered strings are not Anagram"); else printf("\nThe two entered strings are Anagram"); } else printf("\nsame number of characters must be present in both the strings to be an Anagram"); getch(); return 0; }
Output:
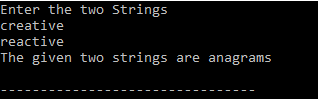
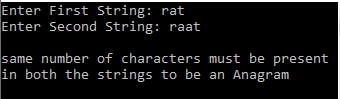
3. By Calculating Frequency Of Characters
In this program, the frequency of each character is calculated and this frequency is compared with the frequency of other string. If the frequency is same then the two strings are Anagram else Not Anagram.
#include <stdio.h> int anagram(char [], char []); int main() { char i[100], j[100]; printf("Enter the two Strings\n"); gets(i); gets(j); //checking anagrams if (anagram(i, j) == 1) printf("The given two strings are anagrams\n"); else printf("The given two strings are not anagrams\n"); return 0; } int anagram(char i[], char j[]) { int first1[26] = {0}, second1[26] = {0}, d=0; // the frequency of characters in the first string is calculated while (i[d] != '\0') { first1[i[d]-'a']++; d++; } d = 0; while (j[d] != '\0') { second1[j[d]-'a']++; d++; } // the frequency of characters in the second string is calculated for (d = 0; d < 26; d++) { if (first1[d] != second1[d]) return 0; } return 1; }
Output:
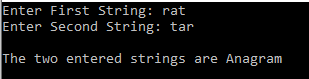
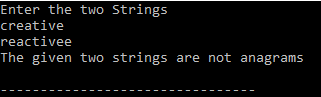
4. By comparing ASCII values of two strings
In this program, two Strings course and source are declared, then the compiler compares the ASCII values of two strings. If ASCII values of two strings are same the Strings are Anagram else Not Anagram.
#include<stdio.h> #include<stdbool.h> #include<string.h> # define NO_OF_CHARACTERS 26 // Checking if the given strings are anagrams using functions bool Anagram(char *Test1, char *Test2) { // two count arrays are created and initialized to 0 int Count1[NO_OF_CHARACTERS] = {0}; int Count2[NO_OF_CHARACTERS] = {0}; int r=0; if (strlen(Test1) != strlen(Test2)) return false; // count is incremented in count array for each character in the given input strings //the ascii value of 'a' is 97 for (r = 0; Test1[r] && Test2[r]; r++) { Count1[Test1[r]-97]++; Count2[Test2[r]-97]++; } // count arrays are compared by using the assigned value to NO_OF_CHARACTERS for (r = 0; r < NO_OF_CHARACTERS; r++) if (Count1[r] != Count2[r]) return false; return true; } int main() { char Test1[] = "grab"; char Test2[] = "brag"; if (Anagram(Test1, Test2)) printf("The two strings are anagram of each other"); else printf("The two strings are not anagram of each other"); return 0; }
Output:

Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- LCM program in C