Today we will LCM Program in C and also learn GCD and LCM program in C.So before starting you should have knowledge about LCM and GCD.So,
Table of Contents
What is LCM?
LCM stands for Least Common Multiple. The LCM of two numbers a and b is the smallest positive integer which is perfectly divisible by both a and b (without remainder).
For Example, LCM of 2 and 3 is 6.LCM of 72 and 120 is 360.
What is GCD?
GCD stands for Greatest Common Divisor. The GCD of two or more integers(other than zero), which is the largest positive integer that divides both the integers.
For Example, GCD of 8 and 12 is 4.
Algorithm for LCM:
1 Step: Initialize the two integers A and B with +ve Integers.
2 Step: then Store Maximum of A and B in variable max.
3 Step: Now check whether max is divisible by A and B.
4 Step: If it is divisible then, Display max as LCM.
5 Step: If not divisible then, change multiple of the max and repeat step 3.
6 Step: STOP.
1. LCM Program in C
In this program, the compiler will ask user to enter two positive integers other than zero. When the two numbers are accepted then the program will start doing its operation and finally print the LCM of two numbers.
#include <stdio.h> int main() { int i, num1, num2, max, lcm=1; /* Input two numbers from user */ printf("Enter any two numbers to find LCM: "); scanf("%d%d", &num1, &num2); /* Find maximum between num1 and num2 */ max = (num1 > num2) ? num1 : num2; /* First multiple to be checked */ i = max; /* Run loop indefinitely till LCM is found */ while(1) { if(i%num1==0 && i%num2==0) { /* * If 'i' divides both 'num1' and 'num2' * then 'i' is the LCM. */ lcm = i; /* Terminate the loop after LCM is found */ break; } /* * If LCM is not found then generate next * multiple of max between both numbers */ i += max; } printf("LCM of %d and %d = %d", num1, num2, lcm); return 0; }
Output:
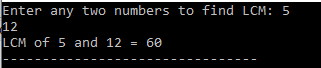
2. GCD program in C
#include <stdio.h> int main() { int n1, n2, i, gcd; printf("Enter two integers: "); scanf("%d %d", &n1, &n2); for(i=1; i <= n1 && i <= n2; ++i) { // Checks if i is factor of both integers if(n1%i==0 && n2%i==0) gcd = i; } printf("G.C.D of %d and %d is %d", n1, n2, gcd); return 0; }
Output:
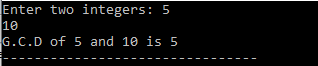
3. Calculate LCM Using GCD
In this program, we have calculated the LCM using the concept of GCD.
#include <stdio.h> int main() { int n1, n2, i, gcd, lcm; printf("Enter two positive integers: "); scanf("%d %d", &n1, &n2); for (i = 1; i <= n1 && i <= n2; ++i) { // check if i is a factor of both integers if (n1 % i == 0 && n2 % i == 0) gcd = i; } lcm = (n1 * n2) / gcd; printf("The LCM of two numbers %d and %d is %d.", n1, n2, lcm); return 0; }
Output:
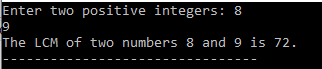
4. LCM Program in C Using Recursion
The Recursion means the user-defined function which calls itself directly or indirectly. In this program, the compiler will ask you to enter two values and the print its LCM Using the recursive function.
#include <stdio.h> long gcd(long x, long y); int main() { int Num1, Num2, GCD, LCM; printf("Please Enter two integer Values \n"); scanf("%d %d", &Num1, &Num2); GCD = gcd(Num1, Num2); LCM = (Num1 * Num2) / GCD; printf("LCM of %d and %d is = %d", Num1, Num2, LCM); return 0; } long gcd(long x, long y) { if (y == 0) { return x; } else { return gcd(y, x % y); } }
Output:
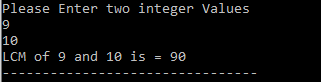
In the above program we have used the traditional LCM formula LCM=(Num1*Num2) / GCD;.
When the Compiler reaches to the line in the main gcd(long x, long y) the compiler will directly move to the function long gcd(long x, long y)
Let’s check above program condition’s if(y==0) will check whether the given number is 0 or not. In recursion, it’s very important to give the condition else that the program will execute infinitely.
Hope you understand the program with logic.
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Program to Add Two Numbers in C.