Today we will learn C Program to Add Two Numbers in C and also learn Program to Add Two Numbers in C.
The Addition is the Arithmetic Operation in which the sum of two numbers is performed.
For Example, The addition of 5 and 6 is 11.
Table of Contents
Algorithm of Adding two Numbers
1 Step: START.
2 Step: Initialize integers A, B and C.
3 Step: Accept two integers A and B from User.
3 Step: Now do the operation using formula C=A+B.
4 Step: Now the addition is stored in C Variable.
5 Step: Print C.
6 Step: STOP.
Now we will do this arithmetic operation in C.There are different methods to make Addition in C we will see it one by one.
1. Addition Program in C
In this program, we will ask the user to enter the two numbers and do addition using formula. This addition will store in the third Variable and at the end, the value stored in the third variable is printed.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//c program to add two numbers using third variable C #include <stdio.h> int main() { int A,B,C; printf("Enter two integers for addition: "); scanf("%%d %%d", &A, &B); // calculating sum C = A + B; printf("The addition of %%d and %%d is=%%d",A,B,C); return 0; }</pre> </div>
Output:
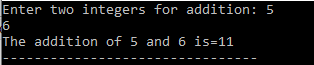
2. Addition of Two Numbers Without Using Third Variable
In the first program after doing the addition of A and B we store that value in third variable C but, in this program, we will not use the third variable we will store value in same variable A.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//c program to add two numbers without using third variable #include <stdio.h> int main() { int A=5,B=7; // calculating sum A = A + B; printf("The addition of 5 and 7 is=%%d",A); return 0; }</pre> </div>
Output:

This program is not recommended because we have initialized the variables A and B with 5 and 7 we didn’t take numbers from the user and the original value of A is lost.
3. C program to Add Two Numbers Repeatedly
The process of addition is same as program one only change is that first program end’s after doing one operation but in this program, we will use while loop which continues the addition until user press n for the exit.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>#include <stdio.h> int main() { int A, B, C; char ch; while (1) { printf("Input two integers\n"); scanf("%%d%%d", &A, &B); getchar(); C = A + B; printf("(%%d) + (%%d) = (%%d)\n", A, B, C); printf("Do you wish to add more numbers (y/n)\n"); scanf("%%c", &ch); if (ch == 'y' || ch == 'Y') continue; else break; } return 0; }</pre> </div>
Output:
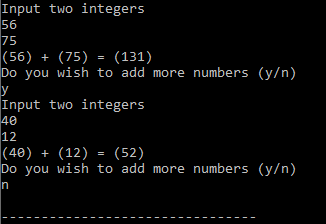
4. Program to Add Two Numbers Using Function
Till now we do not use user-defined functions to calculate the sum of two numbers But, in this program, we will use a user-defined function to do the addition of two numbers.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//addition using function #include<stdio.h> long addition(long, long); int main() { long first, second, sum; printf("Enter two numbers for addition \n"); scanf("\n%%ld\n%%ld", &first, &second); sum = addition(first, second); printf("The addition is=%%ld\n", sum); return 0; } long addition(long a, long b) { long result; result = a + b; return result; }</pre> </div>
Output:
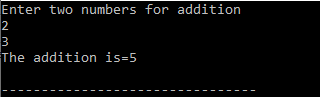
In the above program, we have used a user-defined function addition. When the compiler reaches to sum=addition(long a, long b) then compiler directly jumps to the function addition and perform the operation.
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Multiplication of Matrix in C