Today we will learn C Program to Swap two numbers and also learn C Program to Swap Two Numbers without using third variable using functions, pointers, X-OR operator and so on. So,
Table of Contents
What is Swapping?
Generally, the meaning of swapping is to interchange something or change the position of the variables.
For Example: Consider two variables A and B.The value of A is 5 and the value of B is 10 so after swapping the value of a will become 10 and the value of B will become 5.
Algorithm:
1 Step: START.
2 Step: Declare the Variables A, B with values.
3 Step: Declare the temp variable.
4 Step: Logic: temp=A; A=B; and B=temp;
5 Step: Print the values.
6 Step: STOP.
There are different methods to do swapping of two numbers we will see it one by one.
1. Swapping of two numbers Using third Variable
In this program, we will use a third variable temp to store the values of A and B temporarily.
Logic: The idea of using the third variable is very simple.First store the value of A in a temp variable and store the value of B in A.After storing the value of B in A then store the value of temp variable in B.
Thus the two numbers are swapped.
#include<stdio.h> int main() { float A, B, temp; printf("Enter first number: "); scanf("%f", &A); printf("Enter second number: "); scanf("%f", &B); // Value of A is assigned to temp temp = A; // Value of B is assigned to A A = B; // Value of temp (initial value of A) is assigned to B B = temp; printf("\nAfter swapping, A = %.2f\n", A); printf("After swapping, B = %.2f", B); return 0; }
Output:
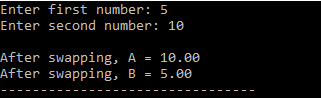
2. Swapping of two numbers Without Using Third Variable
In the above program, we used the third variable temp to store the values of A and B temporarily. But, in this program, we will not use a temporary variable.
#include <stdio.h> int main() { int var1, var2, temp; printf("Enter two integers\n"); scanf("%d%d", &var1, &var2); printf("Before SwappingnFirst variable = %d\nSecond variable = %d\n", var1, var2); var1 = var1 + var2; var2 = var1 - var2; var1 = var1 - var2; printf("After SwappingnFirst variable = %d\nSecond variable = %d\n", var1, var2); return 0; }
Output:
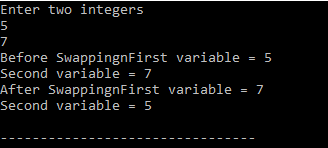
Logic:
In the first variable, we are storing the sum of both the variables. Then in the next step, we are extracting the value of first variable by subtracting the value of second variable from the sum and storing it in the second variable.
At last we extract the original value of second variable and store it in first variable.
i.e var1=var1+var2; var2=var1-var2; and var1=var1-var2;
3. Swapping of two numbers using a function
In this program, we will use user-defined function Swap() to swap two numbers. The compiler asks the user to enter two numbers that user wants to swap.
When the compiler visit the line Swap(A, B) then compiler directly jumps on the function Swap(int A, int B)
#include <stdio.h> void Swap(int, int); int main() { int A, B; printf("\nPlease Enter the value of A and B\n"); scanf("%d %d", &A, &B); printf("\nBefore Swap: A = %d and A = %d\n", A, B); Swap(A, B); return 0; } void Swap(int A, int B) { int Temp; Temp = A; A = B; B = Temp; printf("\nAfter Swapping: A = %d and B = %d\n", A, B); }
Output:
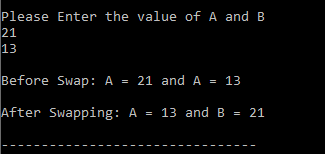
4. Swapping of two numbers using pointer
In this program, we will pass the address of variables to two different variables.
#include <stdio.h> int main() { int a, b, *i, *j, Temp; printf("\nPlease Enter the value of a and b\n"); scanf("%d %d", &a, &b); printf("\nBefore Swap: a = %d b = %d\n", a, b); i = &a; j = &b; Temp = *i; *i = *j; *j = Temp; printf("\nAfter Swapping: a = %d b = %d\n", a, b); printf("\nAfter Swapping: i = %d j = %d\n", *i, *j); return 0; }
Output:
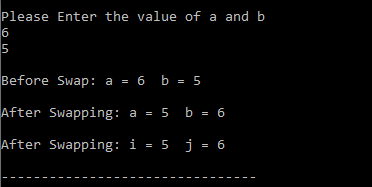
we have assigned the address of variables a and b to the i and j.
5. Swapping Using Call by Reference
#include <stdio.h> void swap(int*, int*); //Swap function declaration int main() { int x, y; printf("Enter the value of x and y\n"); scanf("%d%d",&x,&y); printf("Before Swapping\nx = %d\ny = %d\n", x, y); swap(&x, &y); printf("After Swapping\nx = %d\ny = %d\n", x, y); return 0; } //Swap function definition void swap(int *a, int *b) { int t; t = *b; *b = *a; *a = t; }
Output:
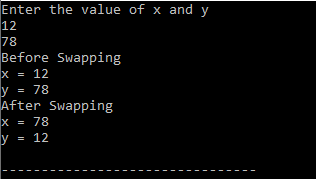
6. Swapping of two numbers Using Bitwise X-OR operator
In this program, we will use bitwise X-OR Operator for swapping of two numbers.
#include <stdio.h> int main() { int x, y; printf("Enter two integers\n"); scanf("%d%d", &x, &y); printf("x = %d\ny = %d\n", x, y); x = x ^ y; y = x ^ y; x = x ^ y; printf("x = %d\ny = %d\n", x, y); return 0; }
Output:
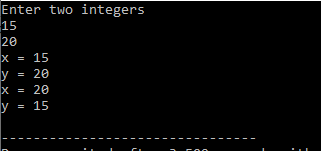
Explanation:
Let us consider the user enters the value of x and y as 8 and 4.The Binary values of 8 and 4 are 1000 and 0100.
The code is x=x^y i.e x=1000^0100, therefore, the value of x becomes 0011.
After y=x^y i.e y=0011^0100, therefore, the value of y becomes 1000.
Now x=x^y i.e x=0011^1000,therefore, the value of x becomes 0100.Hence both numbers are swapped.1000=8 and 0100=4.
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Addition of Two Numbers in C
- Matrix Multiplication Program in C.
- Playfair Cipher Program in C.
- GCD Program in C.
- Tower of Hanoi in C.
- First Fit Program in C
- Menu-driven program in C.