Today we will learn How to write a Menu Driven Program Using Switch case in C.Before starting we will learn about switch case statement.
Table of Contents
What is Switch Case?
The Switch statement is the type of statement which allows user to execute one code block among many alternatives.
We use a switch statement when we want to write 2 or more than 2 programs in a single program.
Inside the switch statement, cases are used we can number these cases using number or alphabets like case ‘a’: or case ‘1’:.After the case ends break statement should compulsorily be added.
To access a particular case we have to enter the case number After executing the program and the program written in that case starts executing.
when the user enters case number other than declared then default statement is used.
Flowchart of Switch Case:
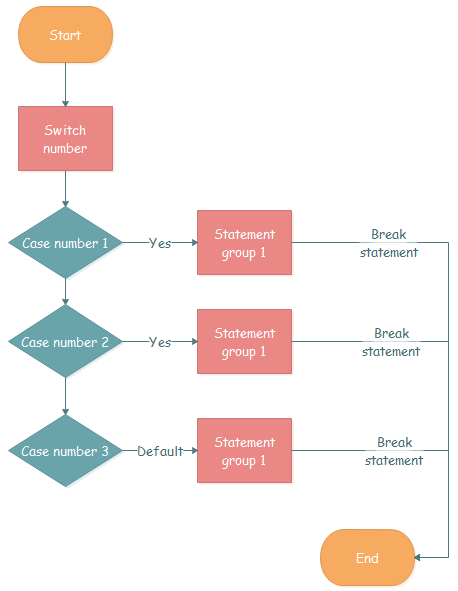
Now we will learn how to write c program using switch statement using different types of examples.
1. Menu-driven Program Example 1
Write a menu-driven program to calculate 1. The Area of Circle 2. Area of Square 3. Area of Sphere
#include <stdio.h> int input(); void output(float); int main() { float result; int choice, num; printf("Press 1 to calculate area of circle\n"); printf("Press 2 to calculate area of square\n"); printf("Press 3 to calculate area of sphere\n"); printf("Enter your choice:\n"); choice = input(); switch (choice) { case 1: { printf("Enter radius:\n"); num = input(); result = 3.14 * num * num; printf("Area of sphere="); output(result); break; } case 2: { printf("Enter side of square:\n"); num = input(); result = num * num; printf("Area of square="); output(result); break; } case 3: { printf("Enter radius:\n"); num = input(); result = 4 * (3.14 * num * num); printf("Area of sphere="); output(result); break; } default: printf("wrong Input\n"); } return 0; } int input() { int number; scanf("%d", &number); return (number); } void output(float number) { printf("%f", number); }
Output:
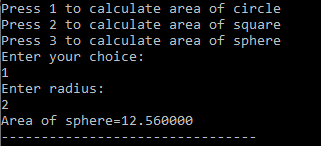
2. Menu Driven Program in C Example 2
Write a Menu Driven Program to find 1.Factorial of a number 2.Prime Number 3. Find Number is odd or even
#include<stdio.h> int main() { int choice, num, i; unsigned long int fact; while(1) { printf("1. Factorial \n"); printf("2. Prime\n"); printf("3. Odd\\Even\n"); printf("4. Exit\n\n\n"); printf("Enter your choice : "); scanf("%d",&choice); switch(choice) { case 1: printf("Enter number:\n"); scanf("%d", &num); fact = 1; for(i = 1; i <= num; i++) { fact = fact*i; } printf("\n\nFactorial value of %d is = %lu\n\n\n",num,fact); break; case 2: printf("Enter number:\n"); scanf("%d", &num); if(num == 1) printf("\n1 is neither prime nor composite\n\n"); for(i = 2; i < num; i++) { if(num%i == 0) { printf("\n%d is not a prime number\n\n", num); break; } } /* Not divisible by any number other than 1 and itself */ if(i == num) { printf("\n\n%d is a Prime number\n\n", num); break; } case 3: printf("Enter number:\n"); scanf("%d", &num); if(num%2 == 0) // 0 is considered to be an even number printf("\n\n%d is an Even number\n\n",num); else printf("\n\n%d is an Odd number\n\n",num); break; case 4: exit(0); // terminates the complete program execution } } return 0; }
Output:
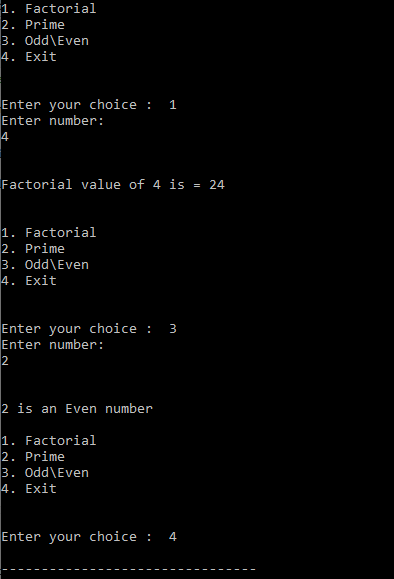
In the above program when the case execution is complete the compiler again ask the user to enter the number to perform the operation.Because we have used while(1) in the starting of the program. And when we press 0 then the program is exited.
3. Menu Driven Program Using Function Example 3
Write a menu-driven program using a function which can do the following operations: 1. Addition of two numbers 2. Subtraction of two numbers 3. multiplication of two numbers 4. division of two numbers.
#include <iostream> using namespace std; void showChoices(); float add(float, float); float subtract(float, float); float multiply(float, float); float divide(float, float); int main() { float x, y; int choice; do { showChoices(); cin >> choice; switch (choice) { case 1: cout << "Enter two numbers: "; cin >> x >> y; cout << "Sum " << add(x,y) <<endl; break; case 2: cout << "Enter two numbers: "; cin >> x >> y; cout << "Difference " << subtract(x,y) <<endl; break; case 3: cout << "Enter two numbers: "; cin >> x >> y; cout << "Product " << multiply(x,y) <<endl; break; case 4: cout << "Enter two numbers: "; cin >> x >> y; cout << "Quotient " << divide(x,y) <<endl; break; case 5: break; default: cout << "Invalid input" << endl; } }while (choice != 5); return 0; } void showChoices() { cout << "MENU" << endl; cout << "1: Add " << endl; cout << "2: Subtract" << endl; cout << "3: Multiply " << endl; cout << "4: Divide " << endl; cout << "5: Exit " << endl; cout << "Enter your choice :"; } float add(float a, float b) { return a + b; } float subtract(float a, float b) { return a - b; } float multiply(float a, float b) { return a * b; } float divide(float a, float b) { return a / b; }
Output:
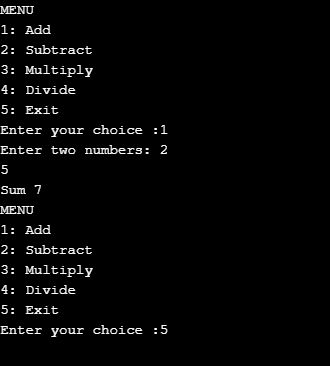
In above program we have used function add() for addition,subtract() for subtraction,multiply() for multiplication and divide() for division.
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Addition of Two Numbers in C
- Matrix Multiplication Program in C.
- Playfair Cipher Program in C.
- GCD Program in C.
- Tower of Hanoi in C.
- First Fit Program in C
- Swapping of two numbers in C
- Odd or Even Number Program in C