Today we will learn the Round-robin program in C and we will also learn Round Robin Algorithm. So before Start learning, we will make you know about Round Robin Program in C.
Table of Contents
What is Round Robin?
The Round-robin (RR) scheduling algorithm is designed especially for timesharing systems.
It is similar to FCFS scheduling except that is a purely preemptive algorithm.
A small unit of time, called a time quantum or time slice, is defined. A time quantum is generally from 10 to 100 milliseconds
The ready queue is treated as a circular queue. The ready queue is treated like a FIFO queue of processes. New processes are added to the tail of the ready queue. The CPU picks the first process from the ready queue, sets a timer to interrupt after 1-time quantum, and dispatches the process.
Round Robin Algorithm:
The CPU scheduler travels the ready queue, allocates the CPU to each process for a time interval of up to 1-time quantum, the process is preempted and the next process in the queue is allocated the CPU.
If the process has a CPU burst of less than 1-time quantum then the process releases the CPU and the scheduler selects the next process in the ready queue.
If the CPU burst of the currently running process is longer than 1-time quantum, the timer will go off and will cause an interrupt to the operating system. A context switch will be executed, and the process will be put at the tail of the ready queue. The CPU scheduler will then select the next process in the ready queue.
Advantages:
Algorithm logic is simple.
System supports multiprogramming effectively.
Gives a better response than SJF.
Disadvantages:
The average waiting time under RR is often long.
If the duration of time quantum is very large, the system acts as FIFO.
Average turnaround time is higher than SJF.
If the duration of time quantum is very small, then Every time a dispatcher is called. More context switching and a large amount of time is wasted in transferring PCB contents to internal registers to PCB.
For Example:
consider the following set of processes and their burst time. Let the time is 20 units.
Process | Burst Time |
---|---|
P1 | 53 |
P2 | 17 |
P3 | 68 |
P4 | 24 |
Working of Round Robin:
The Process P1 will be allocated for 20-time units and then pre-empted, process P2 is allocated time units.
The Process continues till all processes terminates.
The Gantt chart Shows the details:
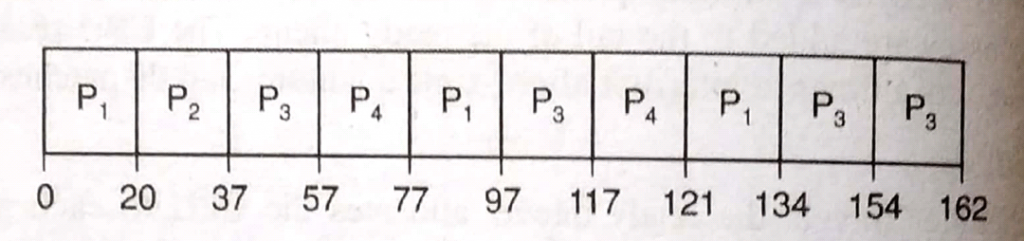
To calculate waiting time of the processes
Waiting time for a process = (Start time – arrival time) + (New start time – Old finish time)
1. Waiting time for P1 = (0-0)+(77-20)+(121-97) = 81.
2. Waiting time for P2 = (20-0) = 20.
3. Waiting time for P3 = (37-0)+(97-57)+(134-117) = 94.
4. Waiting time for P4 = (57-0)+(1177-77) = 57+40 = 97.
5. Average Waiting time = (292)/4 = 73.
Waiting Time: Waiting time is the sum of periods spent waiting in ready queue as the CPU scheduling algorithm does not affect the amount of time that a process spends waiting in the ready queue.
Turn-Around Time: One major factor from the process’s point of view is how long it takes to execute that process. Turnaround time is the interval from the time of submission of a process to the time of completion. i.e
Turn around time=(time spent in waiting to get into memory)+(time spent waiting in ready queue)+(time spent executing in CPU)+(time spent in doing I/O).
1. Round Robin Program in C
#include<stdio.h> int main() { int i, limit, total = 0, x, counter = 0, time_quantum; int wait_time = 0, turnaround_time = 0, arrival_time[10], burst_time[10], temp[10]; float average_wait_time, average_turnaround_time; printf("\nEnter Total Number of Processes:\t"); scanf("%d", &limit); x = limit; for(i = 0; i < limit; i++) { printf("\nEnter Details of Process[%d]\n", i + 1); printf("Arrival Time:\t"); scanf("%d", &arrival_time[i]); printf("Burst Time:\t"); scanf("%d", &burst_time[i]); temp[i] = burst_time[i]; } printf("\nEnter Time Quantum:\t"); scanf("%d", &time_quantum); printf("\nProcess ID\t\tBurst Time\t Turnaround Time\t Waiting Time\n"); for(total = 0, i = 0; x != 0;) { if(temp[i] <= time_quantum && temp[i] > 0) { total = total + temp[i]; temp[i] = 0; counter = 1; } else if(temp[i] > 0) { temp[i] = temp[i] - time_quantum; total = total + time_quantum; } if(temp[i] == 0 && counter == 1) { x--; printf("\nProcess[%d]\t\t%d\t\t %d\t\t\t %d", i + 1, burst_time[i], total - arrival_time[i], total - arrival_time[i] - burst_time[i]); wait_time = wait_time + total - arrival_time[i] - burst_time[i]; turnaround_time = turnaround_time + total - arrival_time[i]; counter = 0; } if(i == limit - 1) { i = 0; } else if(arrival_time[i + 1] <= total) { i++; } else { i = 0; } } average_wait_time = wait_time * 1.0 / limit; average_turnaround_time = turnaround_time * 1.0 / limit; printf("\n\nAverage Waiting Time:\t%f", average_wait_time); printf("\nAvg Turnaround Time:\t%f\n", average_turnaround_time); return 0; }
Output:
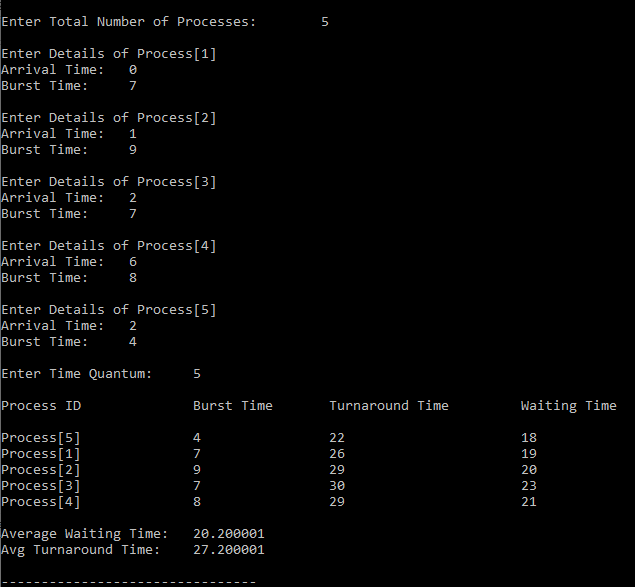
2. Round Robin Program in C++
// C++ program for implementation of RR scheduling #include<iostream> using namespace std; // Function to find the waiting time for all // processes void findWaitingTime(int processes[], int n, int bt[], int wt[], int quantum) { // Make a copy of burst times bt[] to store remaining // burst times. int rem_bt[n]; for (int i = 0 ; i < n ; i++) rem_bt[i] = bt[i]; int t = 0; // Current time // Keep traversing processes in round robin manner // until all of them are not done. while (1) { bool done = true; // Traverse all processes one by one repeatedly for (int i = 0 ; i < n; i++) { // If burst time of a process is greater than 0 // then only need to process further if (rem_bt[i] > 0) { done = false; // There is a pending process if (rem_bt[i] > quantum) { // Increase the value of t i.e. shows // how much time a process has been processed t += quantum; // Decrease the burst_time of current process // by quantum rem_bt[i] -= quantum; } // If burst time is smaller than or equal to // quantum. Last cycle for this process else { // Increase the value of t i.e. shows // how much time a process has been processed t = t + rem_bt[i]; // Waiting time is current time minus time // used by this process wt[i] = t - bt[i]; // As the process gets fully executed // make its remaining burst time = 0 rem_bt[i] = 0; } } } // If all processes are done if (done == true) break; } } // Function to calculate turn around time void findTurnAroundTime(int processes[], int n, int bt[], int wt[], int tat[]) { // calculating turnaround time by adding // bt[i] + wt[i] for (int i = 0; i < n ; i++) tat[i] = bt[i] + wt[i]; } // Function to calculate average time void findavgTime(int processes[], int n, int bt[], int quantum) { int wt[n], tat[n], total_wt = 0, total_tat = 0; // Function to find waiting time of all processes findWaitingTime(processes, n, bt, wt, quantum); // Function to find turn around time for all processes findTurnAroundTime(processes, n, bt, wt, tat); // Display processes along with all details cout << "Processes "<< " Burst time " << " Waiting time " << " Turn around time\n"; // Calculate total waiting time and total turn // around time for (int i=0; i<n; i++) { total_wt = total_wt + wt[i]; total_tat = total_tat + tat[i]; cout << " " << i+1 << "\t\t" << bt[i] <<"\t " << wt[i] <<"\t\t " << tat[i] <<endl; } cout << "Average waiting time = " << (float)total_wt / (float)n; cout << "\nAverage turn around time = " << (float)total_tat / (float)n; } int main() { // process id's int processes[] = { 1, 2, 3}; int n = sizeof processes / sizeof processes[0]; // Burst time of all processes int burst_time[] = {10, 5, 8}; // Time quantum int quantum = 2; findavgTime(processes, n, burst_time, quantum); return 0; }
Output:
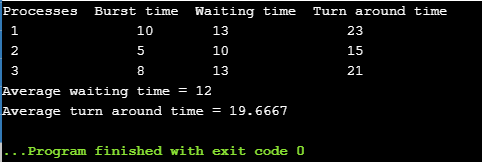
Also Read:
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Decimal to Binary Conversion Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C
- Program to print prime numbers from 1 to n
- Anagram program in C.
- Calculate LCM Program in C.
- Addition of Two Numbers in C
- Matrix Multiplication Program in C.
- Playfair Cipher Program in C.
- GCD Program in C.
- Tower of Hanoi in C.
- First Fit Program in C
- Swapping of two numbers in C
- Menu Driven Program in C.
- Odd or Even number program in C
- Simple Interest Program in C