Table of Contents
What is Multidimensional Array in C?
An array having more than one dimension is called multidimensional array in C language. A two-dimensional array is the simplest form of a multidimensional array.
By placing n number of brackets [ ] we can declare n-dimensional array where n is dimension number.
In general, an n-dimensional array declaration is as shown below:
<data_type> array_name[d1][d2]…..[dn]
For example:
int a[5][5][5] Three Dimensional Array.
int a[2][5][4][4] Four-Dimensional Array.
A total number of elements that can be stored in a multidimensional array can be calculated by multiplying the size of all the dimensions.
The three-dimensional array is an array or collection of 2D arrays. Below figure shows a visualization of a 3D array having 3 tables and each table having 4rows and 2 columns.
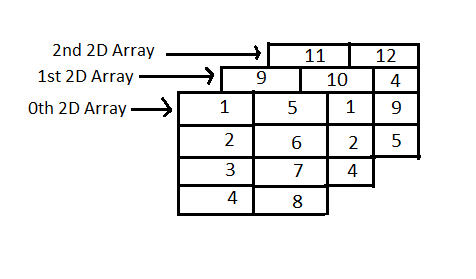
Initialization of Multidimensional Array in C
Let us see an example of a three-dimensional array. We can initialize a three-dimensional array in a similar way like a two-dimensional array. Here is an example,
int test[2][3][4]={{{3,4,2,3},{1,2,3,4},{5,6,7,8}},{{13,4,56,3},{5,9,1,3},{8,9,10,45}}};
Accessing Multidimensional Array Elements
We can access elements using three subscripts. The following code shows how to initialize and access the 3D array.
//Learnprogramo #include<stdio.h> int main() { int tables, rows, columns; int Employees[2][2][3] = { { {9, 99, 999}, {8, 88, 888} }, { {225, 445, 665}, {333, 555, 777} } }; for (tables = 0; tables < 2; tables++) { for (rows = 0; rows < 2; rows++) { for (columns =0; columns < 3; columns++) { printf("Employees[%d][%d][%d] = %d\n", tables, rows, columns, Employees[tables][rows][columns]); } } } return 0; }
Output:
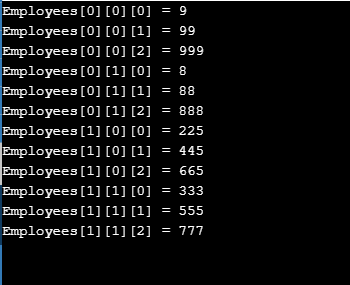
Explanation:
First Iteration: First the value of the tables will 0 and the given condition(tables<2) will be true. So, the compiler will enter in the second for loop i.e row iteration.
Row First Iteration: Initially the value of row will be 0 and condition(rows<2) will be True. So the compiler will enter into third for loop i.e column iteration.
Column First Iteration: At first the value of the column will be 0 and condition (columns<2) will be true. So, the compiler will start executing the given statements inside the loop until the given condition gets failed.
This process goes on continuously and the elements get printed on the screen until the condition gets failed.
Sample Program
Write a C program to do matrix multiplication.
In this program first, we will ask the user how many rows and columns you want for matrix A and B then the program will ask the user to enter the elements and then the compiler will print the multiplication of Matrix A and B in Matrix C.
//Learnprogramo #include <stdio.h> void main() { int A[50][50],B[50][50],C[50][50],i,j,k,r,s; int m,n; printf("How many rows and columns for first matrix\n"); scanf("%d%d",&m,&n); printf("How many rows and columns for second matrix\n"); scanf("%d%d",&r,&s); if(m!=r) printf("\n The matrix cannot multiplied"); else { printf("\n Enter the elements of first matrix "); for(i= 0;i<m;i++) { for(j=0;j<n;j++) scanf("\t%d",&A[i][j]); } printf("\n Enetr the elements of second matrix "); for(i=0;i<m;i++) { for(j=0;j<n;j++) scanf("\t%d",&B[i][j]); } printf("\n The element of first matrix is"); for(i=0;i<m;i++) { printf("\n"); for(j=0;j<n;j++) printf("\t%d",A[i][j]); } printf("\n The element of second matrix is"); for(i=0;i<m;i++) { printf("\n"); for(j=0;j<n;j++) printf("\t%d",B[i][j]); } for(i=0;i<m;i++) { printf("\n"); for(j=0;j<n;j++) { C[i][j]=0; for(k=0;k<m;k++) C[i][j]=C[i][j]+A[i][k]*B[k][j]; } } } printf("\n Multiplication of two matrix is"); for(i=0;i<m;i++) { printf("\n"); for(j=0;j<n;j++) printf("\t%d",C[i][j]); } }
Output:
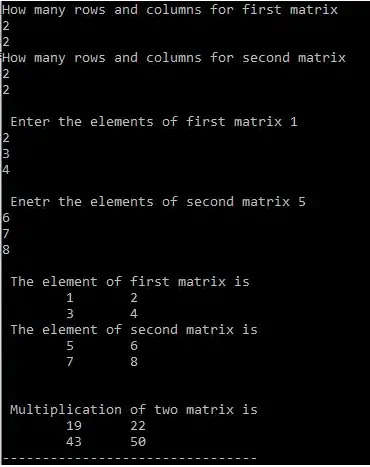
At first, we initialize the variables and array in main and then ask the user for rows and columns for Matrix A and Matrix B.
When the user enters the rows and columns then compiler asks to enter the elements for Matrix A and Matrix B.Then matrix addition is done and the addition is stored in third matrix C.
Finally the matrix is printed on the screen.
Conclusion:
In this lesson, we have learned about the multidimensional array in C. Now, in the next lesson, we will learn passing an array to a function in C.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.