Table of Contents
What is Algorithm in C?
An algorithm is just an outline or idea behind a program. In other words, an algorithm in C can be defined as the stepwise procedure to represent the solution to the problem. The first step in the program development is to devise and describe a precise plan of what you want the computer to do.
This plan, expressed as a sequence of operations is called an algorithm.
An algorithm is expressed in pseudo code – something resembling C language or Pascal, but with some statements in English rather than within the programming language. It is expected that one could translate each pseudocode statement to a small number of lines of actual code, easily and mechanically.
Definition
An Algorithm is defined as, “a set of explicit and unambiguous finite steps which, when carried out for a given set of initial conditions, produce the corresponding output and terminate in a finite time”.
Characteristics of an Algorithm
Every algorithm should follow the following characteristics or criteria:
1. Input:
There should be zero or more input values which are to be supplied to algorithm.
2. Output:
At least one result should be produced by the algorithm.
3. Definiteness:
Each step must be clear and unambiguous.
4. Finiteness:
If we trace the steps of an algorithm, then for all cases, the algorithm must terminate after a finite number of steps.
5. Effectiveness:
Each step must be sufficiently basic that a person using only paper and pencil can in principle carry it out. In addition, not only each step is definite, but it must also be feasible.
How to Write algorithms?
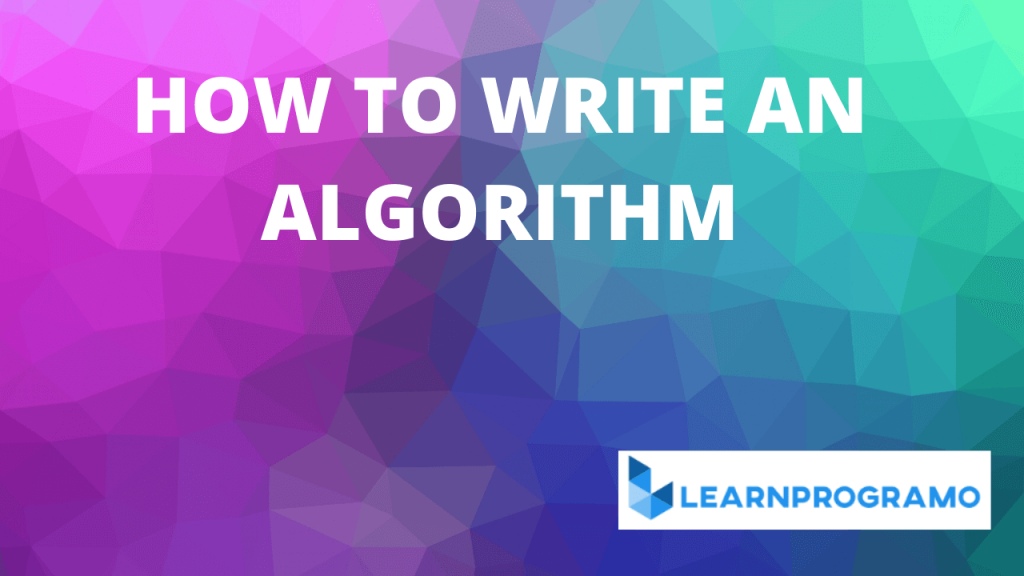
The process of writing algorithms consists of the following steps:
Step 1: Define your Algorithms Input:
Many algorithms take in data to be processed, to calculate the area of rectangle input may be the rectangle height and rectangle width.
Step 2: Define the Variables:
Algorithm’s variables allow you to use it for more than one place. We can define two variables for rectangle height and rectangle width as HEIGHT and WIDTH(or h and w).
We should use the meaningful variable name, for instance, instead of using H and W use HEIGHT and WIDTH as a variable name.
Step 3: Outline the Algorithm’s Operations:
Use the input variable for computation purpose. For example, to find the area of rectangle multiply the HEIGHT and WIDTH variable and store the value in a new variable say AREA.
An Algorithm’s operations can take the form of multiple steps and even branch, depending on the value of the input variables.
Step 4: Output the Results of your Algorithm’s Operations:
In the case of the area of the rectangle, the output will be the value stored in variable AREA. If the input variables described a rectangle with a HEIGHT of 2 and a WIDTH of 3, the algorithm would output the value of 6.
Examples of what is algorithm in C:
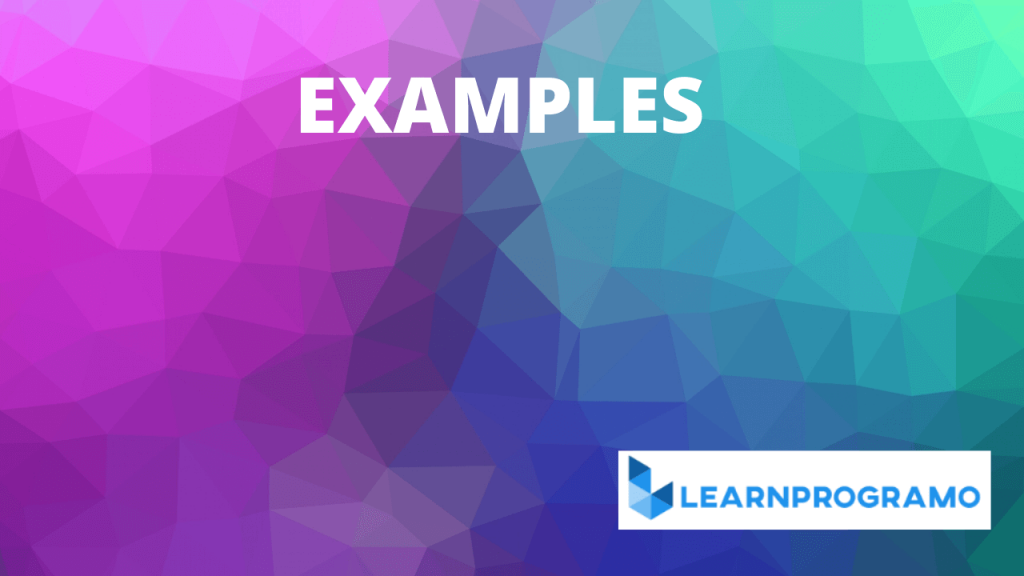
Let us try to develop an algorithm to compute and display the sum of two numbers.
Example 1: To find the sum of two numbers
1st Step: START.
2nd Step: Read two numbers a and b.
3rd Step: Calculate the sum of a and b and store it in new variable sum.
4th Step: Display the value of the sum.
5th Step: STOP.
Example 2: To find area and perimeter of Rectangle
1st Step: START.
2nd Step: Input side length and breadth say L, B.
3rd Step: AREA=LxB.
4th Step: PERIMETER=2x(L+B).
5th Step: Display AREA, PERIMETER.
6th Step: STOP.
Example 3: To convert Temperature from Fahrenheit to Celsius
1st Step: START.
2nd Step: Input Temperature in Fahrenheit say as F.
3rd Step: C=5.0/9.0(F-32).
4th Step: Display Temperature in Celcius C.
5th Step: STOP.
Example 4: To find the smallest of two numbers
1st Step: START.
2nd Step: Input two numbers say as num1, num2.
3rd Step: If num1<num2 then print smallest is num1 else print smallest is num2 and end if.
4th Step: STOP.
Example 5: To find the sum of first n natural numbers(1+2+…+n)
1st Step: START.
2nd Step: Accept the n term.
3rd Step: Let i=1, sum=0.
4th Step: Let sum=sum+i.
5th Step: Let i=i+1( or increment i by 1).
6th Step: if(i<=n) then goto step 4.
7th Step: Display sum.
8th Step: STOP.
Advantages and Disadvantages of Algorithms
Advantages of Algorithm:
It is the step-wise representation of a solution to a given problem, which makes it easy and simple to understand.
An algorithm uses a definite procedure. It facilitates program development by acting as a design document or a blueprint of a given problem solution.
It is not dependent on any programming language, so it is easy to understand for anyone even without programming knowledge.
Every step in an algorithm has its own logical sequence so it is easy to debug.
By using the algorithm, the problem is broken down into smaller pieces or steps hence, it is easier for a programmer to convert it into an actual program.
Disadvantages/Limitations of Algorithm:
In large algorithms, the flow of program control becomes difficult to track.
It is difficult to show branching and looping.
Algorithms lack visual representation of programming constructs like flowcharts, thus understanding the logic becomes relatively difficult in a complex problem.
Also Read: