Table of Contents
Structures and Unions in C
Concept of Structure
An array allows us to store a group of elements of the same data type together. In many cases, we need to store data items of different types as a group. Structures and Unions in C allows a set of elements of different types to be stored as a group.
The structure is also called ‘records’ in some languages. The use of structures and unions in c helps organize complicated data because they permit a group of related variables to be treated as a unit rather than as separate entities.
Structure Definition
A structure is a composition of variables, possibly of different data types, grouped together under a single name. Each variable within the structure is called a ‘member’. The name given to the structure is called a ‘structure tag’.
The members of a structure can be of any data type including the basic type, array, pointer and other structures.
Declaration and Initializing Structure
A structure can be declared in the following way.
Syntax:
struct structure_name
{
1st member;
2nd member;
.
.
nth member;
};
The struct keyword is used to declare structures. The members of the structure and enclosed in { }. a structure declaration does not allocate any memory. i.e., it reserves no storage. Memory is allocated only when variables are created. A structure can contain:
- Simple variables of built-in types.
- variables of other structures.
- arrays.
- pointers.
Examples:
struct student
{
char name[20];
int rollno;
float percentage;
};
1. Creating Structure Variables
There are two ways to create variables or instances of a structure.
1st Way:
struct structure_name
{
structure_members;
} instance;
2nd Way:
struct structure_name
{
structure_members;
};
struct tag instance;
In a 1st way, the variables are declared immediately after the structure template.
In a 2nd way, the variables are declared later using the structure tag.
Example:
//Learnprogramo struct student { char name[20]; int rollno; float percentage; stud1, stud2; }
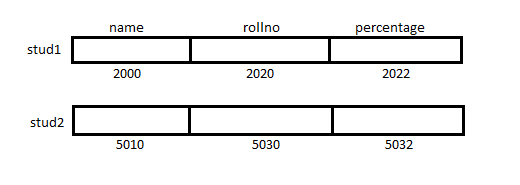
The size of a structure variable can be found using the sizeof operator.
sizeof(stud1) = sizeof(name)+sizeof(rollno)+sizeof(percentage)
= 20+2+4
= 26 bytes
2. Initializing a Structure Variable
Members of structures and unions in C variable can be assigned values during declaration.
Syntax:
struct structure_name variable={ value1, value2,…};
The initialization values are enclosed in { }.
A number and type of values should match the number and type of data members in the structure.
If there are fewer initializers than the members, the remaining members are initialized with 0.
Example:
struct student s = {“Learnprogramo”, 20, 85.2);
Use of typedef:
typedef is a unary operator in ‘C’ which creates a user-defined type. We can use typedef with a structure to give a new name to the structure.
Syntax:
typedef struct structure_name
{
1st member;
2nd member;
.
.
nth member;
}new_structure_name;
Example:
//Learnprogramo typedef struct student { char name[20]; int rollno; float percentage; }STUDENT;
Here, STUDENT becomes a new type name. To create a variable, we can directly use STUDENT as a new type name without using the struct keyword.
STUDENT s1,s2;
typedef struct
{
char name[20];
int rollno;
float percentage;
}STUDENT;
Accessing Structures and Unions in C Members
Structure members can be accessed using the structure member operator(.) also called the dot operator. This operator is used between the structure variable and the member name.
Syntax: structure_variable.member
Example:
struct student stud1;
stud1.name
stud1.rollno
stud1.percentage
Individual members of the structures can be used just like other variables. Values can also be assigned to those members.
strcpy(stud1.name,”ABCD”);
stud1.rollno=100;
stud1.percentage=85.2;
They can be read and displayed using the scanf and printf functions.
scanf(“%s%d%d”,stud1.name,&stud1.rollno,&stud1.percentage);
printf(“%s%d%d”stud1.name,stud1.rollno,stud1.percentage);
The following program accepts details for five student variables and also displays them.
//Learnprogramo #include <stdio.h> struct student { char firstName[50]; int roll; float marks; } s[10]; int main() { int i; printf("Enter information of students:\n"); for (i = 0; i < 5; ++i) { s[i].roll = i + 1; printf("\nFor roll number%d,\n", s[i].roll); printf("Enter first name: "); scanf("%s", s[i].firstName); printf("Enter marks: "); scanf("%f", &s[i].marks); } printf("Displaying Information:\n\n"); for (i = 0; i < 5; ++i) { printf("\nRoll number: %d\n", i + 1); printf("First name: "); puts(s[i].firstName); printf("Marks: %.1f", s[i].marks); printf("\n"); } return 0; }
Output:
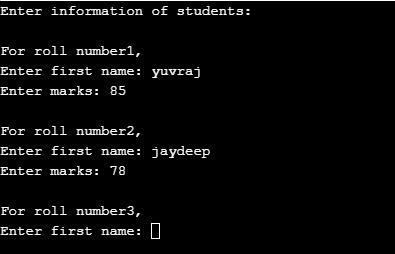
Nested Structures
The individual members of the structure can be other structures as well. A structure within another structure is called a nested structure.
Syntax:
struct outer
{
1st member;
2nd member;
struct inner
{
1st member;
…..
}inner_member;
};
Example:
//Learnprogramo struct student { char name[20]; int rollno; struct date { int day; int month; int year; }birth_date,admission_date; float percentage; }s;
Here, the student structure has the date as a nested structure. The variable of student structure is s. The variables of date structure are birth_date and admission_date. The members of variable s are:
s.name, s.rollno, s.birth_date, s.admission_date, s.percentage
Accessing Members of Nested Structure
To access members of the nested structure, the following syntax is used:
Syntax: outer_variable.inner_variable.inner_variable
Example: s.name, s.rollno
Initialization
To initialize a variable that belongs to a structure containing a nested structure, the initialization values have to the given in the same order as declared in the structure.
Array of Structures
It is possible to create an array of structures just like any other array. This array will have structures as its elements.
Syntax: struct structure_name array_name[size];
Example: struct student stud[10];
Here, the array has 10 structure variables: stud[0] to stud[9]. These are stored sequentially in memory.
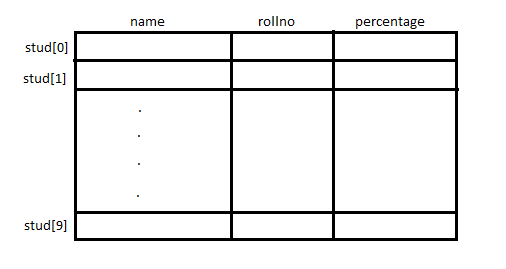
Individual elements can be accessed as
stud[i].name
stud[i].rollno
where i goes from 0 to 9.
The following program accepts data of 10 students viz. name, roll no, marks in three subjects and percentage.
//Learnprogramo #include <stdio.h> struct student { char name[50]; int roll; float marks; }s[100]; int main() { int i,n; struct student s[100]; printf("Enter total of students:\n"); scanf("%d",&n); for(i=0;i<n;i++) { printf("\n Enter information of student %d:\n",i+1); printf("Enter name: "); scanf("%s", s[i].name); printf("Enter roll number: "); scanf("%d", &s[i].roll); printf("Enter marks: "); scanf("%f", &s[i].marks); } printf("Displaying Information:\n"); for(i=0;i<n;i++) { printf("\n %d no. student info\n",i+1); printf("\tName:%s\n ",s[i].name); printf("\t Roll number: %d\n",s[i].roll); printf("\t Marks: %.1f\n\n",s[i].marks); } return 0; }
Output:
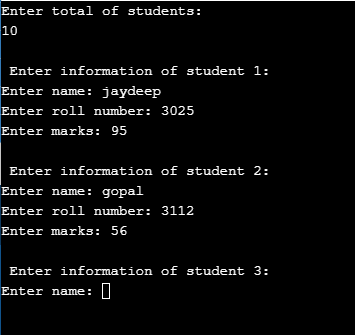
Unions
A union is very similar to a structure but only one of its members can be used at a time. Unions provide a way to manipulate different kinds of data in a single area of storage.
There are many applications where we would want to store different data terms at different times and all are not needed at the same time. A union provides a facility to do this.
Definition
A union is a collection of data items of different data types grouped together under a single name. All members share the same memory area and only one member can be used at a time.
Declaration of Union
A union is declared in the same way as a structure except that the keyword ‘union’ is used instead of ‘struct’.
Syntax:
union union_name
{
union_members;
}instance;
Example:
//Learnprogramo union u { char s[5]; int num; }u1;
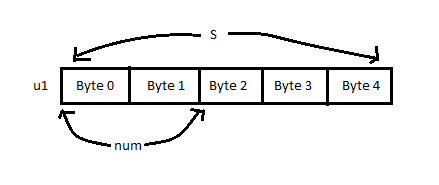
The variable u1 will be allocated memory to accommodate the largest member of the union. In this example, it will be allocated 5 bytes.
Only one member can be stored at a time. We can store either array s or num. Both do not exist simultaneously. To calculate the size of the union, the sizeof operator is used. For the example above, sizeof(u1) will be 5.
Accessing Members of the Union
Union members can be accessed using the dot operator i.e.,
union_variable.member
If we have a pointer to the union (similar to the pointer to a structure), the members are accessed using the ->operator.
union_pointer->member
Example:
union u *ptr=&u1;
Accessing members:
u1.s ptr->s
u1.num ptr->num
Initializing a Union
Since only one member of the union can be used at a time, only the first member can be initialized during declaration.
Example:
union u
{
char s[5];
int num;
};
union u var={“ABC”};
This will be stored as follows:
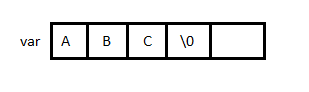
Union within a Structure
A union can be nested within another union or a structure.
Consider the example: Suppose we wish to store information about an employee-id, name, and type. The type can be “F” for a full-time employee or “P” for a part-time employee.
If the type is ‘F’, store the monthly_salary. If the type is ‘P’, store the hours_worked.
The structure and union declarations will be:
//Learnprogramo struct employee { char name[20]; int id; char type; union info { int monthly_salary; int hours_worked; }details; }e1,e2;
The following assignments are valid
strcpy(e1.name,”ABC”);
e1.id=456;
e1.type=’F’;
e1.details.monthly_salary=1500;
strcpy(e2.name,”LMN”);
e2.id=458;
e2.type=’P’;
e2.details.hours_worked=15;
Operations on a Union
A union variable can be assigned to another union variable.
The address of the union variable can be obtained using the address-of operator.
Only the first member of the union can be initialized.
A function can accept and return a union or a pointer to a union.
The sizeof operator can be used with a union.
typedef keyword can be used to create a synonym for a union. Instances can then be created using this synonym.
What is the difference between Structure and Union
Points | Structure | Union |
---|---|---|
Memory Allocation | Each member of a structure is allocated separate memory space. | All Members share the same space in memory. |
Size of structure | Structure variable occupies the sum of sizes of all members in the variables. | The amount of memory required is the same as its largest number. |
Member access | Structure members can be accessed at a time. | Only one member can be accessed at a time. |
Initialization | Members of the structure can be initialized. | Only the first member of the union can be initialized. |
An Altering | Altering the value of one member will not affect other members. | Altering the value of one member will change the value of other members. |
Conclusion:
So in this lesson, we have learned about the Structures and unions in C. Now, in the next lesson, we will learn about file handling in C.
Also Read:
- Strings and Points in C.
- An array of String in C.
- String in C.
- Static and Dynamic Memory Allocation in C.
- How to declare Function pointer in C.