Table of Contents
Strings and Pointers in C
Strings and pointers in C are very closely related. Since a string is an array, the name of the string is a constant pointer to the string. This pointer can be used to perform operations on the string.
Declaration: char *pointer;
Example: char *ptr;
Initialization:
Before use, every pointer must be initialized. To initialize a pointer, we assign it the address of a string.
Syntax: pointer = stringname;
Example:
char str[20] = “Hello”;
char *ptr;
ptr=str;
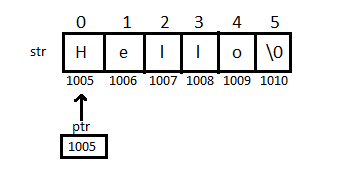
Using the pointer, string characters can be accessed and modified. Each character can be accessed using *ptr. For example, to display all characters using pointer, the following loop is used:
char str[20] = “Hello”, *ptr;
for(ptr=str; *ptr!=’\0′; ptr++)
putchar(*ptr);
In this section, we will see some user-defined string functions using pointers.
1. Find the length of the string
int length(char s) { int count=0; while(s!=’\0′)
{
count++;
s++;
}
return count;
}
2. Copy one string to another and return the copied string.
char *copystring(char *t, char *s)
{
char *temp=t;
while(*s!=’\0′)
{
*t=*s;
t++,s++;
}
*t=’\0′;
return temp;
}
3. Convert a string to uppercase and return the converted string.
char *uppercase(char *s)
{
char temp=s; while(s!=’\0′)
{
if(islower(s)) toupper(s);
s++;
}
return temp;
}
4. Count the occurrences of a character in a string
int charcount(char s, char ch) { int count=0; while(s!=’\0′)
{
if(*s==ch)
count++;
s++;
}
return count;
}
The above user-defined functions can be called in main() as follows:
//Learnprogramo int main() { char s1[20]="ABCDEF", s2[20]="HelloWorld"; printf("%d",length(s1)); printf("%d",charcount(s2,'1')); printf("%d",uppercase(s2)); printf("%d",copystring(s2,s1)); return 0; }
Command Line Arguments
So far we have written main( ) with an empty pair of brackets. But like any other function, it is possible to pass arguments or parameters to main when it starts executing. i.e., at runtime.
These arguments are called command-line arguments because they are passed from the command line during run time.
Declaration of main
To pass command-line arguments to the main function, it has to be declared as follows:
main(int argc, char *argv[])
{
…..
…..
}
main is called with two arguments.
1. int argc:
Argument count which is the number of command-line arguments the program was called or invoked with.
2. char *argv[ ]:
Argument vector. It is an array of pointers each pointing to a command-line argument.
The subscript for argv[ ] are 0 to argc-1.
argv[0] is the name of the executable file of the program (./a.out)
It is not necessary to use the words argc and argv. Any other identifiers can be used. However, they are used by convention. While running the program, the arguments have to be separated by spaces.
How to run the program with command line arguments
A program, which uses command line arguments, can be executed as follows in the Linux environment. In Linux, a.out is the executable file. To execute a program with command-line arguments, use the syntax:
$ ./a.out arg1 arg2…..argn
Example:
$ ./a.out Hello 25 a
It is important to note that all arguments are stored as strings. Even numbers are stored in the form of a string. So, if we need to perform operations on numbers, suitable conversions must be done.
The following program accepts command line arguments and displays them on the screen.
//Learnprogramo #include<stdio.h> void main(int argc, char *argv[]) { int i; printf("Number of arguments = %d\n",argc); printf("The arguments are:\n"); for(i=0;i<argc;i++) printf("%s\n",argv[i]); }
Output:
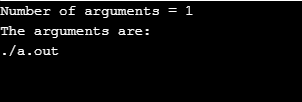
Advantages of Command Line Arguments
Arguments can be supplied during runtime. Therefore, the program can accept different arguments at different times.
There is no need to change the source code to work with different inputs to the program.
Conclusion:
In this lesson we have learned about strings and pointers in C. Now we will start new lesson structures and unions in c.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.
- Multidimensional Array in C.
- Pointer Arithmetic in C.
- How to declare Function Pointer in C.
- String in C.
- An array of Strings in C.