Table of Contents
What is Preprocessor in C?
A Preprocessor in C is a program that processes or analyzes the source code file before it is given to the compiler. But actually what is Preprocessor in C? What is its role in C?
A Preprocessor in C plays an important role in the program execution process. In the C program development cycle, the source code (.C program) is given to the preprocessor before it is compiled.
Advantages of Preprocessor in C
- It takes action according to special instructions called Preprocessor directives which begin with #.
- Joins any lines that end with a backslash character into a single line.
- Divides the program into a stream of tokens.
- Remove comments and replaces them by a single space.
- Processes preprocessor directives and expands macros.
- Replaces escape sequences by their equivalent internal representation.
- Concatenates adjacent constant character strings.
- Replaces trigraph sequences (Non-ASCII character sets) by their equivalent.
The following flowchart shows the sequence of operations of the preprocessor:
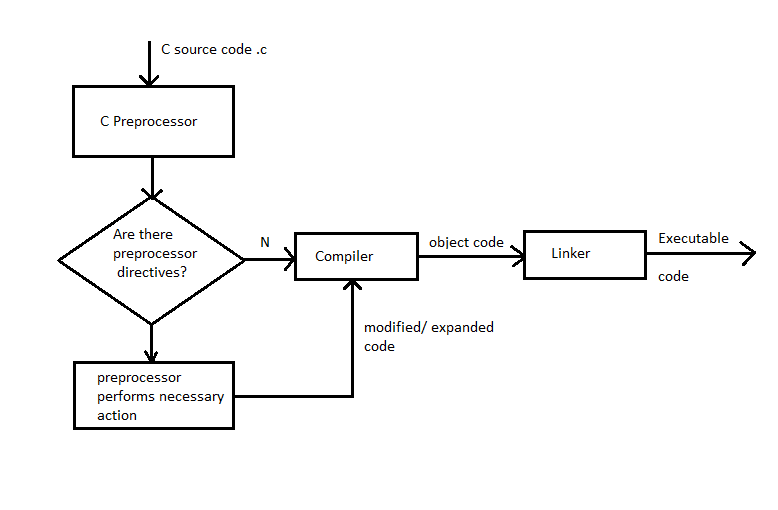
Format of Preprocessor Directives
The preprocessor in C checks the source code for special instructions called preprocessor directives. Pre-processor directives are not ‘C’ language statements but they are special instructions for the pre-processor. The format of the directive is:
A preprocessor directive begins with # and does not end with semicolon(;)
Preprocessor directives in C are of three types:
- File inclusion directive.
- Macro substitution directive.
- Conditional compilation directive.
1. File Inclusion Directive
The file inclusion directive is #include. This directive instructs the compiler to include contents of the specified file, i.e, it inserts the entire contents of the file at that position.
Including a header file is equal to copying the contents of the header file.
Syntax:
#include<filename> OR #include “filename”
In the first format, the filename is searched in standard directories only. In the second, the file is first searched in the current directory. If it is not found there, the search continues in the standard directories.
Any external file-containing user-defined functions, macro definitions etc, can be included. An included file can include other files.
Example 1:
//Learnprogramo #include<stdio.h> #include<string.h>
Example 2:
//Learnprogramo /* group.h */ #include<stdio.h> #include<math.h> #include "myfile.c" /*mainprogram.c*/ #include "group.h" void main() { ... ... }
2. Macro Substitution Directive
Macro substitution is a process where an identifier in a program is replaced by a predefined string or a value. The macro substitution directive is #define.
Different types of macros in C substitution:
- Simple macro.
- Argumented macro.
- Nested macro.
Simple Macro Substitution:
It defined a symbol which represents a value. Wherever the macro name or symbol occurs in a program the pre-processor substitutes the code of the macro at that position.
It is used to define symbolic constants which occurs frequently in a program.
Syntax:
#define macro-id value
Examples:
- #define PI 3.142
- #define TRUE 1
Program:
//Learnprogramo #include<stdio.h> #define PI 3.142 void main() { float radius,area; printf("Enter the radius"); scanf("%f", &radius); area=PI*radius*radius; printf("Area=%f",area); }
Output:

Argumented Macros:
A macro can be defined with arguments. Such a macro is called a function macro because it looks like a function. It represents a small executable code. Each time the macro name is encountered, its arguments are replaced by the actual arguments in the program.
Example:
#define HALFOF(x) x/2 #define SQR(x) x*x #define MAX(x,y) ((x)>(y)?(x):(y))
Wherever the macro occurs in the program, its argument(example:x) is replaced by the variable or value used in the program.
For Example:
result=HALFOF(10);
It is advisable to enclose the arguments in ( ). If the arguments are not enclosed in ( ), it may yield wrong results.
Example: result=HALFOF(10+2);
This will be evaluated as result=10+2/2; and will give incorrect results. The correct way to define the macro would be:
#define HALFOF(x)
Now, result=(10+2)/2; which will give the correct result.
Nested Macros:
A macro can be used within another macro. this is called nesting of macros.
Example: #define CUBE(x) SQR(x)*(x)
In this example, macro SQR is called in a macro named CUBE.
A Macro can also be passed to itself as an argument.
#define MAX(a,b)
#define MAXTHREE(a,b,c)
3. Conditional Compilation Directive
this type of preprocessors in C causes conditionally suppress the compilation portion of the source code.
There are six types of Conditional Compilation Directive:
- #if directives.
- #elif directives.
- #ifdef directives.
- #ifndef directives.
- #else directives.
- #endif directives.
Example:
//Learnprogramo #ifdef MACNAME /*tokens added if MACNAME is defined */ #if TEST <=10 /*tokens added if MACNAME is defined and TEST <= 10 */ #else /*tokens added if MACNAME is defined and TEST > 10 */ #endif #else /*tokens added if MACNAME is not defined */ #endif
If you want to learn preprocessor in c in Hindi then visit here.
Conclusion:
So in this lesson, we have learned about what is a preprocessor in C. So in this way we have completed all the lessons of C language. If you have any doubt then feel free to ask in the comment section below. Please to understand better do more and more practise of C programs.
Also Read:
- Strings and Points in C.
- An array of String in C.
- String in C.
- Static and Dynamic Memory Allocation in C.
- How to declare Function pointer in C.
- Structures and Unions in C.
- File Handling in C.