Table of Contents
String in C
String in C is a sequence of characters with the null character(\0) at the end.
A string literal or string constant is a sequence of zero or more characters enclosed by ” “. A string is an array of the character called NULL character(‘\0).
“Welcome to C” is a string literal and it is stored in memory as:
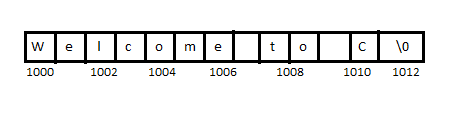
The characters in a string can be any character from the ‘C’ character set which includes alphabets, digits, underscore, special characters and escape sequences. Each character is stored in 1 byte as its ASCII code.
Examples of string literals:
- “Pune”
- “Hello” “Welcome”
- “This is a literal”
String variables
A string variable is an array of characters which stores a string literal.
Declaration
The variable name is declared in the same way as a one-dimensional array with data type char.
Syntax is as follows:
char string_name[length];
A variable name is an identifier. The length determines the maximum number of characters in the string. The length includes the string termination character ‘\0’.
Examples:
char city[10]; char message[50];
Initialization
There are several ways to initialize a string variable.
char message[10]={‘H’,’e’,’l’,’l’,’o’,’\0′};
char message[10]=”Hello”;
In the above declarations, the size of the string variable is 10 characters out of which 6 characters are occupied.
String Input or Output
String input and output can be performed using four functions: gets, scanf, puts, printf.
Input functions: gets( ) and scanf( ). The scanf function does not allow a string which contains spaces. gets( ) allows a string with spaces.
Output functions: puts( ) and printf( ). puts( ) displays a newline character after displaying the string. printf( ) does not display every string on a new line.
Example: Accept the name of a person and display a greeting.
char name[80];
printf(“Enter your name”);
gets(name);
printf(“Good morning %s”,name);
sprintf and sscanf
1. sprintf:
sprintf is the same as printf except that the output is written into a string rather than displayed on the output device. The return value is equal to the number of characters actually placed into the array.
The string is terminated with ‘\0’ and it must be long enough to hold the result.
Prototype:
int sprintf(char * buf, char * format, arg_list)
Example:
char s[80];
sprintf(str, “%s%d%f”, “Hello”,2,5.0);
This will result into data Hello 2 5.0 to be put into the string str.
2. sscanf:
sscanf is identical to scanf except that data is read from the string pointed to by buf rather than stdin. The return value is equal to the number of fields that were actually assigned values.
Prototype:
int sscanf(char *buf, char *format, arg_list)
Example:
char s[20];
int n1, n2;
sscanf(“hello 10 20” “%s%d%d”,s, &n1, &n2);
This assigns hello to string s and 10 and 20 to n1 and n2 respectively.
Program to accept and display a string.
//Learnprogramo #include<stdio.h> void main() { char name[20]; printf("%s",name); printf("Hello %s",name); }
3. Predefined String Functions
C provides a large number of string handling functions in the header file string.h. Each function accepts one or more strings as an argument.
1. strlen – Length of a string
The strlen function takes one string as an argument and returns the number of characters in the string excluding the NULL character ‘\0’.
Syntax: size_t strlen (char * s)
Example:
char message[20]=”Hello”;
printf(“%d”, strlen(message));
2. strlwr – Convert a string to lowercase
The strlwr function takes one string as an argument and converts the string to lowercase. It returns the converted string.
Syntax: char * strlwr(char * s)
Example:
char message[20]=”Hello”;
printf(“%d”, strlwr(message));
3. strupr – Convert a string to uppercase
The strupr function takes one string as an argument and converts the string to uppercase. It returns the converted string.
Syntax: char * strupr(char * s)
Example:
char message[20]=”Hello”;
printf(“%d”, strupr(message));
4. strrev – Reverse a string
The strrev function takes one string as an argument and reverses the string. It returns the reversed string.
Syntax: char * strrev(char * s)
Example:
char message[20]=”Hello”;
printf(“%d”, strrev(message));
5. strcpy – Copy one string to another
The strcpy function takes two strings as an argument and copies the second string into the first. The copied string is returned.
Syntax: char * strcpy(char *target, char *source)
Example:
char src[20]=”Hello”, target[20]=”Hello”;
strcpy(target, src);
printf(“%s%s”, src, target);
6. strcat – Concatenate one string to another
The strcat function takes two string arguments and appends the second string at the end of the first. The NULL character is automatically added at the end.
It returns the concatenated string. The size of the first string must be large enough to store the concatenated string.
Syntax: char * strcat{char *first, char *second}
Example:
char str[20]=”Learn”, str2[20]=”Programo”;
strcat(str1, str2);
printf(“%s%s, str1, str2);
7. strcmp – Compare two strings
The strcmp function takes two string arguments and compares them lexicographically. It compares the two strings character by character. The function returns an integer which is:
0 if both strings are equal. Greater than 0 if the first string is greater than the second string. And less than 0 if the first string is less than the second string.
Syntax: int strcmp(char *first, char *second)
Example:
ans= strcmp(“Hello”, “Hello”);
User Defined String Functions
In the above program, the string operations were performed using standard library functions. It is also possible to perform operations on strings without using these functions.
To perform the operations, we can access individual characters from the string and manipulate them. This can be done using a loop which will continue until we reach the NULL character.
for(i=0; s[i]!=’\0′; i++)
The above code can be written in a user-defined function. The string or strings should be passed to the function as arguments.
A general structure of the user-defined function is:
return_type function_name(char s1[80], char s2[80]…)
{
//performs operations on strings s1,s2
}
Conclusion:
In this lesson, we have learned about string in c. Now, in the next lesson, we will learn about the array of strings in c.