Table of Contents
What is File Handling in C?
The file handling in C means storing the data of a software or program in a particular file. The file handling in C is the only method to store the data in the file.
Introduction to File Handling in C
Many applications perform operations on a large amount of data. If this data has to be entered through the standard input device, it is time-consuming and moreover, once the program execution is over, the input data, as well as the output, is lost.
To store input and output data in a permanent manner, files must be used. The input data can be read from a file and the output of a program can be stored in a file as depicted below.
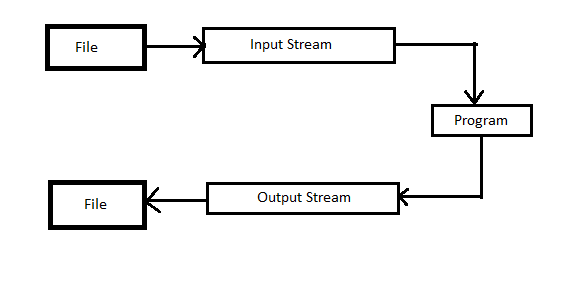
Streams
All input or output operations in ‘C’ are done using streams. A stream is a sequence of bytes of data. A sequence of bytes flowing into a program is an input stream, and the one flowing out is an output stream.
There are 5 predefined streams, which are automatically opened when a C program starts executing and are closed when the program terminates.
Stream | Meaning |
---|---|
stdin | Standard input device. |
stdout | Standard Output device. |
stderr | A standard error output device. |
stdprn* | A standard printer. |
stdaux* | The standard auxiliary device. |
Example:
The output of a function like printf( ) or puts( ) goes to the stream stdout. The scanf( ) receives its input from stream stdin. For performing an input-output operation on files, the file must be associated with a stream.
Types of Files
Files are of two types:
1. Text Files:
In a text file, all information is stored in the form of characters. Eve numeric data is stored in the form of characters. Each character occupies one byte of memory in the file.
These characters are organized in the form of lines with a special character at the end of the line.
The new-line character is converted into a Carriage Return-Line Feed combination and stored in the file. The file is terminated with a special character i.e. EOF. These files can be created, edited and read using any editor.
2. Binary Files:
In a binary file, all data is stored using its internal representation. Data is stored as a sequence of bytes. Characters are stored in one byte, integer in 2 or 4 bytes and so on.
Since data is stored in its internal binary representation, binary files are not in a human-readable form. Special software creates these files and the same software must be used to read these files.
Difference between Text and Binary Files
Text Files | Binary Files |
---|---|
Information is stored in the form of characters. | Information is stored as a sequence of bytes. |
Each character occupies one byte of memory. | Different types of data have different memory requirements. Character occupies 1 byte, integer 2 bytes and float 4 bytes. |
There is a special line terminating character. | There is no special line termination character. |
A character translation may take place in text files. | No character translation occurs. |
The number of characters read or written may not be same as those stored on the external device. | one-to-one correspondence between the bytes read and the bytes store is not done. |
A text file is in human readable form. Any editor can be used to read a text file. | The binary file can be read and edited using special software. |
File Operations in C
Various operations can be performed on files, These are:
- Open a file.
- Read data from a file.
- Write data to a file.
- Close a file.
- Detect end-of-file.
C provides various functions to perform these operations on files. All operations are performed using a file pointer.
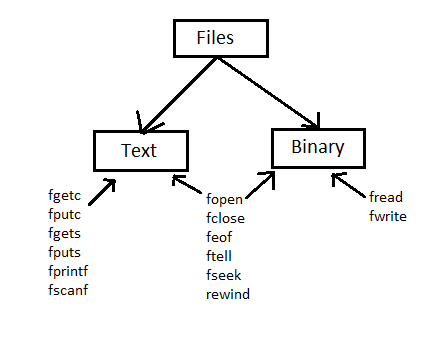
The File Pointer
All file operations are performed using a file pointer. A file pointer is a pointer variable of type FILE which is defined in stdio.h. The file pointer points to a specific file in memory. This pointer is used to perform the operations.
Syntax: FILE *file_pointer;
File Operations in C
1. Opening a File
Before performing any file Input or Output operation, the file operations in c must be opened. The process of opening a stream and linking a file to it is called opening a file.
The fopen( ) function must be used. If the open operation is successful, fopen returns a pointer to type FILE. If it fails, it returns NULL.
Prototype:
FILE *fopen(const char *filename, const char *mode);
The function fopen requires two arguments:
Mode | Meaning |
---|---|
“r” | It Opens a file for reading. If file does not exist fopen() returns NULL. |
“w” | Creates a file for writing. If specified file does not exist, a new one is created. If it exists, existing contents will be overwritten. |
“a” | It Opens a file for appending. If specified file does not exist it is created. |
“r+” | Opens the file for reading and writing. If specified file does not exist, it is created. If it exists, contents can be read and new contents can be added. |
“w+” | Opens a file for reading and writing. If the specified file does not exist, it is created. If it exists, it is overwritten by new data which can be read. |
“a+” | A file opens for reading and appending. If the specified file does not exist, it is created. If it exists, new data is appended to the end of the file. |
Examples:
//Learnprogramo FILE *fptr; fptr=fopen("in.text","r"); FILE *fptr1; fptr1=fopen("out.txt","w");
2. Closing a File
After the operations on the file have been performed and it is no longer needed, the file handling in c has to be closed using the fclose( ) function.
It ensures that all information associated with the file is flushed out of buffers.
Prevents accidental misuse of the file.
It is necessary to close a file before it can be reopened in a different mode.
Prototype: int fclose(FILE *fp);
Example: fclose(fptr);
We can also close all open streams(except the predefined ones) by using the fcloseall( ) function.
Prototype: int fcloseall(void);
3. Check End of File
In-text files, a special character EOF denotes the end of the file. We can use this character to identify that the file has ended. Another method to check if the file has ended is by using the library function feof( ) which returns TRUE if the end of file is reached.
Prototype: int feof(FILE *fp);
Example:
//Learnprogramo if(feof(fptr)==1) printf("File has ended");
4. Reading and Writing Characters
1. fgetc():
fgetc is a function to read a single character from the specified stream.
Prototype: int fgetc(FILE *fp);
It returns a single character from the file pointed by the pointer.
Example:
ch=fgetc(fptr);
2. fputc( ):
fputc is used to write a single character to the specified stream (and its associated file).
Prototype: int fputc(int ch, FILE *fp);
Example:
fptr=fopen(“out.txt”,”w”);
char ch=’A’;
fputc(ch,fptr);
3. fgets( ):
It reads a string of characters from a file.
Prototype: char *fgets(char*str, int n, FILE *fp)
str points to the string where the read string has to be stored.
n is the maximum number of characters to be read. Characters are read until a new line is encountered of(n-1) characters have been read, whichever occurs first. File handling in C.
Example:
char name[80];
fgets (name,80,fptr);
4. fputs( ):
This function writes a line of characters to a stream. It does not add a new-line to the end of the string. If it is required, then the new line has to be explicitly added.
Prototype: char fputs(char *str, FILE *fp);
Example:
char city[]=”Pune”;
fputs(city, fptr);
5. Formatted File Input/Output Functions
In order to deal with multiple data types, formatted file I/O functions are used. fprintf( ) is used for output and fscanf( ) is used for input.
1. fprintf( ):
this is similar to printf. It is used to read formatted data from a file. Along with the format specifier and arguments, a file pointer is given in the function. Data is written to the file associated with the pointer.
Prototype: int fprintf(FILE *fp), char *format, argument list);
The format string is the same as used for printf. The argument list is the names of variables whose values are written to the file.
Example:
//Learnprogramo char name[ ]="Learnprogramo", int age=20; float amount=1005.50; fprintf(fptr, "%s%d%f", name, age, amount);
2. fscanf( ):
This is similar to scanf except that input comes from a specified file stream instead of stdin.
Prototype: int fscanf(FILE *fp, char*format, address list);
The format string is the same as used for scanf. The address list contains the addresses of the variables where fscanf( ) is to assign the values.
Example: fscanf(fptr, “%s%d%f”, name, &age, &amount);
6. Other Functions
1. fflush:
This causes the buffer associated with an open output stream to be written to the specified file. If it is an input stream, its buffer contents are cleared.
Syntax: fflush(FILE *fp);
Example: fflush(stdin);
2. remove:
This deletes the file specified. If it is open, be sure to close it before removing it.
Syntax: int remove(const char *filename);
Example: remove(“myfile.txt”);
3. rename:
This function changes the name of an existing disk file.
Syntax: int rename(const char *oldname, const char *newname);
Both files must be in the same disk drive.
Example: rename(“c:\myfile.txt”,”c:\mynewfile.txt”);
Program:
//Learnprogramo // C program to Open a File, // Read from it, And Close the File # include <stdio.h> # include <string.h> int main( ) { // Declare the file pointer FILE *filePointer ; // Declare the variable for the data to be read from file char dataToBeRead[50]; // Open the existing file lprogramo.c using fopen() // in read mode using "r" attribute filePointer = fopen("lprogramo.c", "r") ; // Check if this filePointer is null // which maybe if the file does not exist if ( filePointer == NULL ) { printf( "lprogramo.c file failed to open." ) ; } else { printf("The file is now opened.\n") ; // Read the dataToBeRead from the file // using fgets() method while( fgets ( dataToBeRead, 50, filePointer ) != NULL ) { // Print the dataToBeRead printf( "%s" , dataToBeRead ) ; } // Closing the file using fclose() fclose(filePointer) ; printf("Data successfully read from file lprogramo.c\n"); printf("The file is now closed.") ; } return 0; }
Conclusion:
In this lesson, we have learned about file handling in c. Now we will start the next lesson Preprocessor in C.
Also Read:
- Strings and Points in C.
- An array of String in C.
- String in C.
- Static and Dynamic Memory Allocation in C.
- How to declare Function pointer in C.
- Structures and Unions in C.