Table of Contents
Array of Strings in C
A string is an array of characters. When we want to store many strings, we need an array of strings. An array of strings in c is a two-dimensional array of characters. This is often required in applications with a list of names, etc.
Declaraction
An array of strings in c can be declared as:
char arrayname[m][n];
where m=number of strings and n=length of each string.
Example:
char str[10][20];
str is an array of 10 strings; each storing 20 characters. The 10 strings are str[0]…str[9].
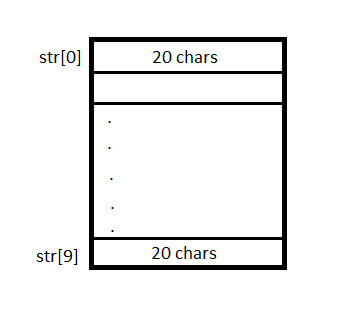
Initialization
A single string can be initialized by giving the characters enclosed in ” “. Since an array of strings in c contains multiple strings, an array of strings can be initialized by giving the string initializers in { } and separated by a comma.
Example:
char cities[4][10]={“Pune”, “Mumbai”,”Delhi”,”Nagpur”};
They are stored as:
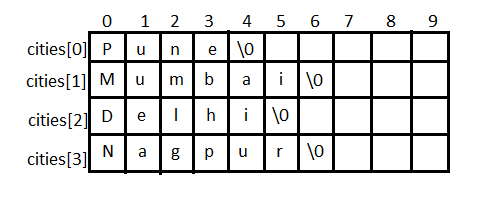
All strings are stored in memory consecutively as shown below:
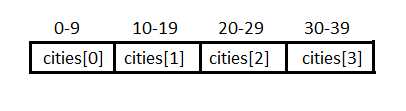
The following illustrates how we accept a list of ‘n’ strings and store them in an array of strings in c and sort alphabetically.
Program 1:
//Learnprogramo #include <stdio.h> #include <string.h> void main() { char name[10][8], tname[10][8], temp[8]; int i, j, n; printf("Enter the value of n \n"); scanf("%d", &n); printf("Enter %d names n \n", n); for (i = 0; i < n; i++) { scanf("%s", name[i]); strcpy(tname[i], name[i]); } for (i = 0; i < n - 1 ; i++) { for (j = i + 1; j < n; j++) { if (strcmp(name[i], name[j]) > 0) { strcpy(temp, name[i]); strcpy(name[i], name[j]); strcpy(name[j], temp); } } } printf("\n----------------------------------------\n"); printf("Input NamestSorted names\n"); printf("------------------------------------------\n"); for (i = 0; i < n; i++) { printf("%s\t\t%s\n", tname[i], name[i]); } printf("------------------------------------------\n"); }
Output:
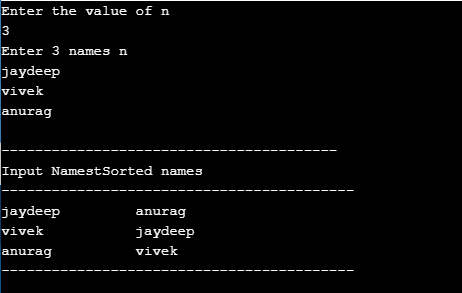
Program 2:
To search for a specific name, we must compare the name in the list using the function strcmp. If the function returns 0, the string is found.
//Learnprogramo #include<stdio.h> #include<string.h> int main() { char str[20][50], s1[50]; int n,i,found=0; printf("Enter how many string (names): "); scanf("%d",&n); printf("Enter %d strings:\n",n); for(i=0;i<n;i++) { scanf("%s",str[i]); } printf("Enter string to search: "); scanf("%s",s1); for(i=0;i<n;i++) { if(strcmp(s1,str[i])==0) { found=1; printf("Found in row-%d\n",i+1); } } if(found==0) printf("Not found"); return 0; }
Output:
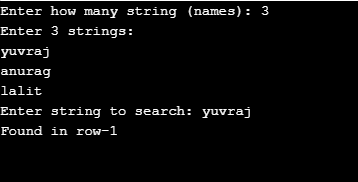
Program 3:
To find the longest name, we must calculate the length of each name using the strlen( ) function. The length of each string is compared with max (which initially 0). Whenever the string length is>max, max is updated and the position of the string is stored.
//Learnprogramo #include<stdio.h> #include<string.h> #define size 100 #define wsize 20 void Longest_Word(char str[][20],int n); void main() { char str[size][wsize]; int i,count=0,n; printf("\n How many words to accept:- "); scanf("%d",&n); printf("\n Enter %d words:- \n \n",n); for(i=0;i<n;i++) scanf("%s",str[i]); Longest_Word(str,n); } // Longest_Word Function void Longest_Word(char str[][20],int n) { int i,Max,len1,c; Max=strlen(str[0]); for(i=1;i<n;i++) { len1=strlen(str[i]); if(len1>Max) { c=i; Max=len1; } } printf("\n The longest string among all is \"%s\" \n \n",str[c]); }
Output:
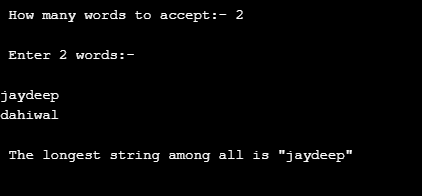
Program 4:
To sort strings alphabetically, we use a sorting method like Bubble sort. This method has passed and in each pass, it compares consecutive pairs of strings using strcmp. If the strings are not in the correct order, they are swapped by using a temporary string.
//Learnprogramo #include <stdio.h> #include <string.h> int main () { char string[100]; printf("\n\t Enter the string : "); scanf("%s",string); char temp; int i, j; int n = strlen(string); for (i = 0; i < n-1; i++) { for (j = i+1; j < n; j++) { if (string[i] > string[j]) { temp = string[i]; string[i] = string[j]; string[j] = temp; } } } printf("The sorted string is : %s", string); return 0; }
Output:
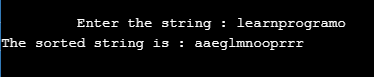
Conclusion:
So in this lesson, we have learnt about the array of strings in C. Now in the next lesson we will learn about strings and pointers in C.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.
- Multidimensional Array in C.
- Pointer Arithmetic in C.
- How to declare Function Pointer in C.
- String in C.