Table of Contents
Static and Dynamic Memory Allocation in C
When variables are declared in a program or static and dynamic memory allocation in c, the compiler calculates the size of the variable and allocates memory to the variable. This method is called static memory allocation.
The amount of memory required is calculated during compile-time. So, In the case of arrays, the size of the array has to be declared at the beginning. In many cases, a user does not know how many elements are to be put.
In such a case, memory is either wasted if the size specified is very large or enough memory is allocated if the size specified is smaller than required.
So, In the Dynamic Memory Allocation method, we can allocate and de-allocate memory whenever required. Dynamic memory allocation means allocating memory at runtime.
C provides four memory allocation and de-allocation functions. They are declared in stdlib.h
Function | Use |
---|---|
malloc | Allocates requested number of bytes and returns a pointer to the first byte. |
calloc | This also allocates memory for a group of objects, initializes them to zero and returns a pointer to the first byte. |
realloc | It changes the size of a previously allocated block of memory and returns pointer to the block. |
free | Releases or frees previously allocated space. |
Allocation
Two functions are used to allocate a block of memory dynamically. These are:
1. malloc:
The malloc() function is used to allocate memory a single block of memory of the specified size. The malloc() function allocates size number of bytes of storage and returns a pointer to the first byte. It returns NULL if the allocation is unsuccessful or if the size is 0.
The prototype of malloc is void * malloc(int size);
Usage:
pointer=(type*)malloc(number_of_bytes);
Explicit type casting is required because by default malloc( ) returns a void pointer.
Example:
To allocate memory to store n integers
int * ptr;
ptr=(int *) malloc(n * sizeof(int));
In the example below, the pointer ptr stores the address of the first byte of the allocated memory.
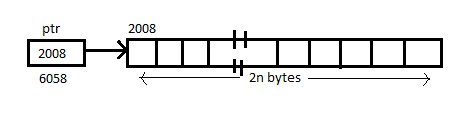
Program:
//Learnprogramo #include<stdio.h> #include<stdlib.h> int main(){ int n,i,*ptr,sum=0; printf("Enter number of elements: "); scanf("%d",&n); ptr=(int*)malloc(n*sizeof(int)); if(ptr==NULL) { printf("Sorry! unable to allocate memory"); exit(0); } printf("Enter elements of array: "); for(i=0;i<n;++i) { scanf("%d",ptr+i); sum+=*(ptr+i); } printf("Sum=%d",sum); free(ptr); return 0; }
Output:
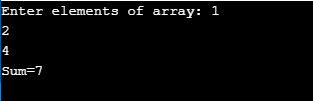
2. calloc:
The calloc( ) function is similar to malloc. It allocates a single block of memory of the specified size and also initializes the bytes to 0.
Its prototype is: void * calloc(size_t_num, size_t_size);
Here, num is the number of objects to allocate and size is the size(in bytes) of each object.
Example:
To allocate memory for n integers,
int * ptr;
ptr=(int *) calloc(n,sizeof(int));
Program:
//Learnprogramo #include<stdio.h> #include<stdlib.h> int main(){ int n,i,*ptr,sum=0; printf("Enter number of elements: "); scanf("%d",&n); ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc if(ptr==NULL) { printf("Sorry! unable to allocate memory"); exit(0); } printf("Enter elements of array: "); for(i=0;i<n;++i) { scanf("%d",ptr+i); sum+=*(ptr+i); } printf("Sum=%d",sum); free(ptr); return 0; }
Output:
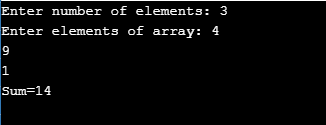
Difference Between malloc( ) and calloc( )
The malloc( ) requires one argument i.e. the total number of bytes to be allocated. calloc( ) requires two arguments – the number of objects and size of each object.
Memory allocated by malloc( ) contains garbage values i.e malloc( ) does not initialize the memory whereas calloc( ) initializes the memory to 0.
3. realloc:
Reallocation means changing the size of an allocated block of memory. The realloc( ) function is used to reallocate memory for a block which was dynamically allocated memory using malloc( ) or calloc( ).
Prototype: void * realloc (void *ptr, size_t size);
ptr points to the original block, size is the requires new size in bytes.
If ptr is NULL, realloc acts like malloc and returns a pointer to it and if argument size is 0, the memory that ptr points to is freed and the function returns NULL.
If sufficient space exists to expand the memory block, additional memory is allocated and the function returns ptr. And if sufficient space does not exist to expand the current block, a new block of the same size is allocated, existing data copied into it, old block is freed and a pointer to the new block is returned.
So, if the memory is insufficient for reallocation(either expanding or allocating new one), the function returns NULL and the old block remains unchanged.
Example:
int n=10, n1=20;
//allocates block for n integers
ptr=(int) malloc(n sizeof(int));
//change the block size
ptr=(int) realloc (ptr,(n+n1)sizeof(int));
4. free:
The dynamically allocated memory is taken from the dynamic memory pool(heap) that is available to the program. After the program finishes using that memory block, it should be freed to make memory available for future use.
The free( ) function is used to release the memory that was allocated by malloc( ) or realloc( ).
Prototype: void free(void *ptr);
Example:
int *ptr;
ptr=(int *) malloc(n * sizeof(int));
….
….
free(ptr);
The free( ) function releases the memory pointed to by ptr.
Difference Between Static and Dynamic Memory Allocation in C
Static Memory Allocation | Dynamic Memory Allocation |
---|---|
Static memory allocation refers to the allocation of memory during the compilation of program. | Dynamic memory allocation refers to the allocation of memory during the execution of program. |
Allocated memory size remains fixed till the program is running. | Memory can be allocated, reallocated and released dynamically. |
Variables remain permanently allocated. | Allocated only when program unit is active. |
Faster execution than dynamic. | Slower execution than static. |
More memory space required. | Optimum usage of memory space. |
Data is stored in data segment of memory. | Data is stored in heap memory. |
Conclusion:
So in this lesson, we have learned about static and dynamic memory allocation in c. Now, in the next lesson, we will learn string in c.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.
- Multidimensional Array in C.
- Pointer Arithmetic in C.
- How to declare Function Pointer in C.