Table of Contents
What are Storage Classes in C?
Storage classes in C are used to describe the features of a variable or function. These features basically include the scope, visibility and life-time which help us to trace the existence of a particular variable during the runtime of a program.
There are four storage classes in C language as follows namely, Auto Storage Class, Static Storage Class, Extern Storage Class and Register Storage Class.
The syntax for storage Class are given below:
storage_specifier data_type variable_name;
Now we will see all the four types of storage classes in detail.
1. Automatic (Auto) Storage Class
The Auto storage class is a default storage class in which variables are of type by default auto. So, the auto keyword is less used while constructing a program in C language.
In order to explicit declaration of variable use ‘auto’ keyword is as follows:
auto int num1;
Variables which are defined within a function or a block by default belong to the auto storage class.
These variables are also called as local variables because these are local to the function and are by default assigned some garbage value.
Since these variables are declared inside a function, therefore these can only be accessed inside that function.
Features of auto Storage Class variable:
Storage: Main Memory.
Scope: Local or block scope.
Default Initial Value: Garbage.
Life Time: Exists as long as control remains in the block.
Program for auto storage class:
//Learnprogramo #include<stdio.h> int main() { auto num=50; { auto num=100; printf("\nNum=%d",num); } printf("\nNum=%d",num); }
Output:
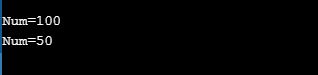
2. Static Storage Class
The Static Storage class is declared with the keyword static as follows:
(static int cnt;)
These variables are automatically initialized to zero. Static variables continue to exist even after the block in which they are defined terminates.
Thus, the value of a static variable in a function is retained or persists between repeated function calls to the same function. Thus, static variables have a property of preserving their value even after they are out of their scope.
Instead of creating and destroying a variable every time it comes into and goes out of scope. A static variable is initialized only once and remains into existence until the end of the program.
Features of Static Storage Class:
Storage: Memory.
Default Initial Value: Zero.
Scope: Local or file scope.
Life Time: Value of the variable persist between different function calls.
Use: In recursive functions and counting the function calls.
Program:
//Learnprogramo #include<stdio.h> int main() { void display(); display(); display(); display(); return 0; } void display() { auto int a=0; static int c=0; c++; a++; printf("%d%d",c,a); }
Output:

In the above program, variable c persists value between function calls while variable a does not.
Difference between Static and Auto Variable:
Static Variable | Auto Variable |
---|---|
We have to specify the storage class to make a variable static. | This is the default storage class. |
Default value is 0. | Default value is garbage. |
Static Variable is having local as well as file scope. | Auto Variable is having block or local scope. |
Retains its value between different function calls. It holds its last value. | Retains its value till the control remains in the block in which the variable is declared. |
This variable should be compiled by compiler first. | Auto variable will compile by the compiler after the static variable. |
Example: static int i; | Example: int i; |
3. External (Extern) Storage Class:
Variables of this storage class are Global variables.
We write extern keyword before a variable to tell the compiler that this variable is declared somewhere else.
Basically, by writing the extern keyword before any variable tells us that this variable is a global variable declared in some other program file.
In order to explicit declaration of variable use
‘extern’ keyword.
When you have multiple files and you define a global variable or function, which will also be used in other files.
Then extern will be used in another file to provide the reference of defined variable or function.
Just for understanding, extern is used to declaring a global variable or function in another file.
Features of External Storage Class:
Storage: Memory.
Default Initial Value: 0.
Scope: Global.
Lifetime: As long as the program’s execution does not come to an end.
The external variable has two cases namely a global variable without extern keyword and A global variable with an extern keyword.
Program: First File main.c
//Learnprogramo #include<stdio.h> int count; extern void write_extern(); int main() { count=5; write_extern(); return 0; }
Program: Second File support.c
//Learnprogramo #include<stdio.h> extern int count; void write_extern(void) { printf("count is%d",count); }
Output:
count is 5.
4. Register Storage Classes in C
The Register Storage Classes in C announces register factors which have similar uses as that of the auto factors.
The main contrast is that the compiler attempts to store these factors in the register of the microprocessor if a free register is accessible.
This utilizes register factors to be a lot quicker than that of the factors put away in the memory during the runtime of the program.
In the event that a free register isn’t accessible, these are then put away in the memory as it were. Generally, scarcely any factors which are to be gotten to every now and again in a program are announced with the register catchphrase which improves the running time of the program.
A significant and fascinating point to be noted here is that we can’t get the location of a register variable utilizing pointers.
We cannot declare register as float and double because float requires 32-bits and double requires 64-bits but the normal capacity of CPU register is usually 16-bits.
Thus, the register storage class is unable to store double and float variables. Whenever register float and register double are declared they are converted into an auto variable.
Register keyword is used to define the local variable.
Syntax: register int count;
One application of register storage class can be in using loops, where the variable gets used a number of times in the program, in a very short span of time.
Features of Register Storage Class:
Storage: CPU registers.
Scope: Local or block scope.
Default Initial Value: Garbage.
Life Time: Exists as long as control remains in the block.
Example:
//Learnprogramo #include<stdio.h> main() { register int i; for(i=1;i<=10;i++) printf("%d",i); }
Output:

Conclusion:
In this lesson, we have learned about four different storage classes in C. So, we have completed the topic of the function. Now from the next lesson, we will start the new topic Arrays in C.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input Output Functions in C.