Table of Contents
What is an Array in C?
An array in C can be defined as “a collection of data items of the same data type which are stored in consecutive memory locations and referred by the common name”.
The Variable allows us to store a single value at a time, what if we want to store roll no. of 100 students? For this task, we have to declare 100 variables, and then assign values to each of them.
What if there are 10000 students or more? As you can see declaring that many variables for a single entity (i.e. student) are not a good idea.
In this chapter, we will discuss a structured data type called an array in C. The structured data type has common characteristics that it is a single variable and it can represent multiple data items. Each data item can be accessed separately.
The Concept Of an Array in C
Suppose, you want to store a salary of 100 employees. You can store salaries Of all employees by creating 100 variable of float data type individually like employeeSalary1, employeeSalary2, employeeSalary3 employeeSalary100.
This is very tedious, time-consuming and difficult to maintain a method of storing 100 salaries.
In this type of situation, C language provides support for array data structure, which solves the problem of storing N similar data. We can declare a float array of length 100 to store salaries of 100 employees.
It is a linear and homogeneous data structure. Linear data structure stores its individual data elements in sequential order in the memory. Homogenous means all the individual data elements are of same data type.
The data type of the elements may be any valid data type like char, int or float.
The elements of an array in C share the same variable name but each element has a different index number known as a subscript. i.e. we can access the individual elements of an array using index or subscript.
So an array in C is also called a subscripted variable. Each subscript must be expressed as a non-negative integer.
The value of each subscript can be expressed as an integer constant. an integer variable or more complex integer expression.
Array declaration tells to Compiler about Type of the array, Name of the Array and Size of array.
The general syntax for declaring an array is as follows:
<data_type> array_name[size1][size2]…..[sizen].
where data_type can be basic data type or structure.
[size1][size2]…..[sizen] specify dimension of an array.
For Example:
int age[100]; double employee[100]; float volume[80][20][40];
For the above problem, we can take an array variable age[100] of type int. The size of this array variable is 100 so it is capable of storing 100 integer values.
The individual elements of this array are age[0], age[1], age[2],age[3], age[4], age[98], age[99].
In C the subscripts start from zero, so age[0] is the first element, age [1] is the second element of an array and so on.
Types of Array in C
The number of subscripts determines the dimension of the array. The maximum dimensions a Program can have to depend on which compiler is being used.
In C, there are three types of array namely, One Dimension Array, Two Dimensional Array and Multi-Dimensional Array.
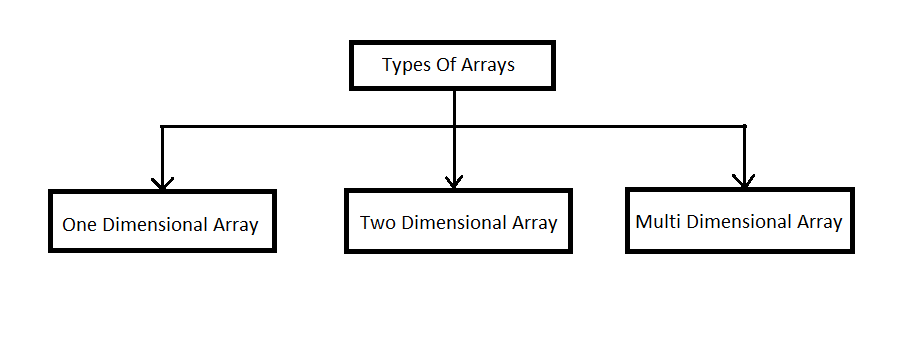
1. One Dimensional Array
A one-dimensional array has one subscript. One Dimensional (1D) array is an array which is represented either in one row or in one column.
The one-dimensional arrays are known as vectors.
In other words, it can be represented as in a single dimension-width or height as shown in the below figure:
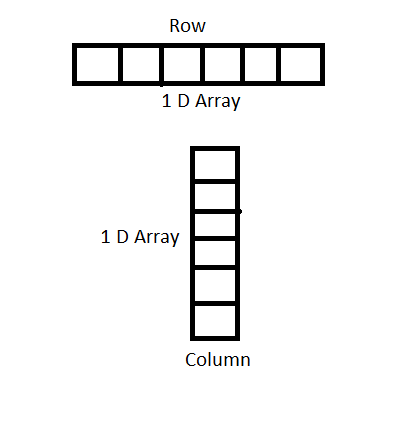
2. Two Dimensional Array
Two Dimensional (2D) array is a fixed-length, homogeneous data type, row and column-based data structure which is used to store similar data type element in a row and column-based structure.
A two-dimensional array is referred to as a matrix or a table. A matrix has two subscripts, one denotes the row and another denotes the column.
In other words, a two-dimensional array is an array of a one-dimensional array.
The general syntax for declaration of a 2D array is given below:
data_type array_name[row] [column];
where data_type specifies the data type of the array. array_name specifies the name of the array, row specifies the number of rows in the array and column specifies the number of columns in the array.
The total number of elements that can be stored in a two array can be calculated by multiplying the size of all the dimensions.
For example The array int x[10][20] can store total (10*20) = 200 elements.
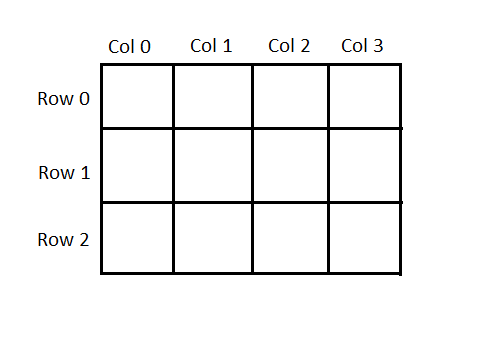
3. Multi-Dimensional Array
An array having more than one dimension is called multidimensional array in C language. A two-dimensional array is the simplest form of a multidimensional array.
By placing n number Of brackets [ ] we can declare n-dimensional array where n is dimension number.
In general, an n-dimensional array declaration is as shown below:
<data_type> array_name[d1][d2]…..[dn]
For example,
int a[5][5][5] Three Dimensional Array.
int a[2][5][4][4] Four-Dimensional Array.
A total number of elements that can be stored in a multidimensional array can be calculated by multiplying the size of all the dimensions.
The three-dimensional array is an array or collection of 2D arrays. Below figure shows visualization of a 3D array having 3 tables and each table having 4rows and 2 columns.
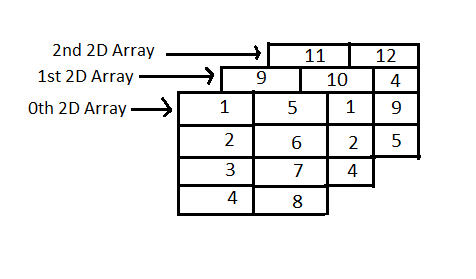
Conclusion:
In this lesson we have learned about array in C and their types. Now in next lesson we will learn One Dimensional Array in C with Detailed Explanation.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.