All programs read data. process data and give some output. The C language does not define any keywords that perform input and output functions in c. Instead, the input and output function in c is accomplished through library functions.
These library functions enable the transfer of data between the C program and standard input or output devices.
These functions can be classified into the following three broad categories:
Console I/O Functions: Functions to receive input from the keyboard and write output to the screen.
File I/O Functions: Functions to perform I/O operations on a file stored on floppy disk or hard disk.
Port I/O Functions: Functions to perform I/O operations on various ports like a printer.
C language always treats all Input or output functions in c data, regardless of where they originate or where they go, as a stream of characters.
A stream of characters or text stream is a sequence of Characters divided into lines. Each line consists of various characters followed by a newline character (\n).
A sequence of bytes flowing into the program is called input stream. A sequence Of bytes flowing out of the program is called output stream.
The Standard input-output devices or the associated files or text streams are referred to as:
stdin: standard input file, normally connected to the keyboard.
stdout: Standard output file, normally connected to the screen or console.
stderr: Standard error display device file, normally connected to the screen.
In order to be able to use input and output functions in C program, you must include pre-processor directive <stdio.h> to include these standard library functions. This can be done with a #include<stdio.h>.
The screen and keyboard together are called a console. Console I/O functions can be further classified into two categories namely, formatted and unformatted console I/O functions.
Table of Contents
Character Input and Output Functions in C
C language provides getchar( ), getch( ) for reading single character and putchar( ), putch( ) for displaying single character on screen. Let us see syntax and use of these functions in C program.
getchar() and putchar() Functions
1. getchar( ):
It reads a single character from input device i.e. stdin. This function is defined in <stdio.h> header file.
Syntax: int getchar( );
Usage: var_name=getchar( );
Where var_name is of type int or char.
The characters accepted by getchar( ) are buffered until RETURN is hit. It is a buffered function. The buffered function gets the input from the keyboard and store it in the memory buffer temporally until user press the Enter key.
It means getchar( ) requires Enter key to be pressed following the character that you typed. It echoes typed character. If an end-of-file(EOF) condition error occurs, getchar() returns EOF.
The EOF macro is defined in <stdio.h> and is often equal to -1.
2. putchar():
It displays or writes a single character to the standard output device or screen at the current cursor position. This function is defined in <stdio.h> header file.
Syntax: int putchar(int c);
Usage: putchar( var_name);
Where var_name is of type int or char.
The putchar( ) function returns the character written or EOF if an error occurs.
Example:
//Learnprogramo #include<stdio.h> int main() { char x; x=getchar(); putchar(x); return 0; }
Output:
If we input any character say j then output is j. So the output is: y /* the keypress is echoed on the screen */ y
getch(), getche() and putch() Functions
1. getch():
This function is used to read a character from the console but does not echo to the screen. This function is included in header file <conio.h>
Syntax: int getch( );
Usage: var_name=getch( );
where var_name is of type int or char.
getch() function is a non-buffered function. It does not use any buffer, so the entered character is immediately returned without waiting for the enter key.
The character data read by this function is directly assigned to a variable rather it goes to the memory buffer.
Another use of this function is to maintain the output on the screen until you have not to press the Enter key. getch() works only on dos like TC compiler. It does not work on a Linux platform.
2. getche():
getche( ) function is used to read a character from the console and echoes that character to the screen. This function is included in header file <conio.h>.
Syntax: int getche( );
Usage: var_name=getche( );
It does not use any buffer, so the entered character is immediately returned without waiting for the enter key. getche() works only on DOS-like TC compiler. It does not work on a Linux platform.
The main difference between getch() and getche() is getch() does not echo character after reading, while getche() echoes character after reading.
3. putch():
putch() function displays or writes single character to the standard output device(i.e. stdout). This function is defined in <conio.h> header file.
Syntax: int putch(int c);
Usage: putch(var_name);
Where, var_name is of type int or char.
putch() does not translate linefeed characters (\n) into carriage-return/linefeed pairs. The putch() function returns the character written or EOF if an error occurs.
//Learnprogramo #include<stdio.h> #include<conio.h> int main() { char x; x=getch(); putch(x); return 0; }
Output:
If we input any character say y then output is y. So the output is:
_ /* the keypress is not echoed on the screen */ y
String Input and Output Functions in C
The functions gets() and puts() are used for string reading(input) and string writing(output) purpose from standard library functions.
1. gets():
gets() function accepts single or multiple characters of string including spaces from the standard input device until ENTER key is pressed. This function in <stdio.h> header file.
Syntax: gets(var_name);
Where var_name is string or array of characters.
gets() is also buffered function. It will read a string from the keyboard until the user presses the Enter key from the keyboard. It will mark the null character (‘\0’) in the memory at the string when you press the enter key.
gets stops when either the newline character is read or when the end-of-file(EOF) is reached, whichever comes first.
2. puts():
puts() function displays or writes a string to the standard output device. It appends a newline character to string. This function is defined in <stdio.h> header file.
Syntax: puts(var_name);
Where var_name is string or array of characters.
//Learnprogramo #include<stdio.h> int main() { char name[20]; puts("Enter the name"); gets(name); puts("Name is:"); puts(name); return 0; }
Output:
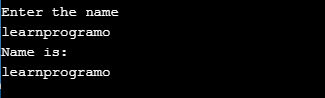
The following table summarizes basic console I/O functions:
Function | Operation |
---|---|
getchar() | Reads character from keyboard and waits for return. |
getche() | Reads a character with echo and do not wait for return. |
getch() | Reads a character without echo and do not wait for return. |
putchar() | Writes a character to the screen. |
putch() | Writes a character to the screen. |
gets() | Reads a string from the keyboard. |
puts() | Writes a string to the screen. |
Formatted Input and Output
Two functions printf() and scanf() are used to perform formatted output and input. printf() function writes data on the screen, while scanf() function reads data from the keyboard.
1. printf() Function:
It is a formatted output function. The printf() function is used to print the data in specified format from the computer’s memory onto a standard output device.
This function can operate on any of the built-in data types. It can be used to output any combination of numerical values, single character and strings.
You can specify the format in which data must be displayed on the screen. It returns the number of characters actually displayed.
The function is included in <stdio.h> file.
Syntax of printf(): int printf(“control string”, arg1, arg2,….., argn);
Format Specifier:
Syntax: %[flags][width][.precesion][length] data-type-specifier
Specifier | Output |
---|---|
c | character |
d | integer |
f | floating point |
Program:
//Learnprogramo #include<stdio.h> int main() { char item[]="ABC"; int part no=12345; float cost=0.50; printf("%s%d%f",item,partno,cost); return 0; }
Output:
ABC 12345 0.050000
The printf() function interprets s-type conversion for strings differently than the scanf function. In the printf() function, the s-type conversion is used to output a string that is terminated by the null character(\0). Whitespace characters may be included within the string.
The f-type conversion is used to output floating-point value.
2. scanf() Function:
The scanf() function reads data from the input device and stores them at the given memory location.
It can handle any built-in type and strings. The function is included in <stdio.h> file.
Syntax: scanf(“control string”, &var1, &var2..);
The variables must precede by address operator which specifies memory address or location where the corresponding input should be stored.
//Learnprogramo #include<stdio.h> int main() { char a; printf("Enter your name="); scanf("%c",&a) printf("Your name is=",a); }
Output:
Enter your name=learnprogramo
Your name is=learnprogramo
sprintf() and sscanf() Functions
1. sprintf() Function:
The sprintf() function works similar to the printf() function except for one small difference.
Instead of sending the output to the screen as printf() does, this function writes the output to an array of characters.
It means this function writes the formatted text to a character array. The return value is equal to the number of characters actually placed into the array.
Syntax: sprintf (char Array[], “Control string” , var1, var2,…..varn);
2. sscanf() Function:
The sscanf() function is identical to scanf() except that data is read from the character array rather than stdin.
The sscanf() function reads the values from a character array and stores each value into variables of matching data type by specifying the matching format specifier.
Syntax: sscanf(char Array[], “Control String”, &var1, &var2…&varn);
Read More: