Table of Contents
Passing an Array to a Function in C
There are two methods for passing an array to a function in c namely, Passing array element by element and passing an entire array to function.
1. Passing Array Element By Element
In this method, array elements are passed one by one to function. The function gets access only one element at a time and cannot modify this value. This is calling by value method of parameter passing.
Example:
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//Learnprogramo #include<stdio.h> main() { int arr[5],i; void fun(int num); printf("\nEnter the 5 elements:"); for(i=0;i<5;i++) scanf("%%d",&arr[i]); printf("\n Passing array element by element.."); for(i=0;i<5;i++) fun(arr[i]); } void fun(int num) { printf("\nElement:%%d",num); }</pre> </div>
Output:
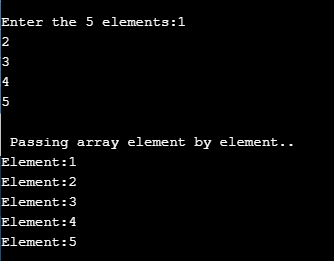
2. Passing Entire Array to Function
In order to pass the entire array, we have to send the name of the array to function. Since array name gives the base address, we are effectively sending entire array.
Name of the array refers to the starting address or base address of the array in memory.
The base address means the memory address of the 0th element of the array. For example, int num[10] ;
So array name num is equivalent=>&num[0]. It is a constant pointer and it cannot be changed.
The function gets access to the original array and so any changes made inside the function affect the original array. Arguments that are passed in this manner are said to be passed by reference rather than by value.
Example:
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//Learnprogramo #include<stdio.h> main() { int arr[5]={10,20,30,40,50}; void disp(int b[5]); void modify(int b[5]); disp(arr); modify(arr); disp(arr); } void disp(int b[5]) { int i; for(i=0;i<5;i++) printf("%%d",b[i]); } void modify(int b[5]) { int i; for(i=0;i<5;i++) b[i]=b[i]*2; }</pre> </div>
Output:
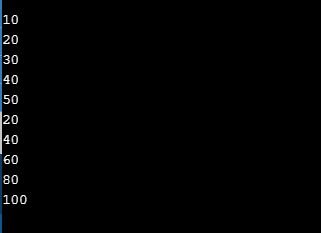
Array Applications
Arrays can be effectively used in searching and sorting elements.
The arrays are used to implement mathematical vectors and matrices, as well as other kinds of rectangular tables.
Many databases, small and large, consist of one-dimensional arrays whose elements are records.
Arrays are used to implement other data structures, such as lists, heaps, hash tables, deques, queues and stacks.
One or more large arrays are sometimes used to emulate in-program dynamic memory allocation, particularly memory pool allocation.
Advantages:
This is a better and convenient way of storing the data of the same data type with the same size.
It allows us to store a known number of elements in it.
It allocates memory in contiguous memory locations for its elements and does not allocate any extra space or memory for its elements. Hence there is no memory overflow or shortage of memory in arrays.
An Iterating the arrays using their index are faster compared to any other methods like linked list etc.
This allows storing the elements in any dimensional array-supports multidimensional array.
Disadvantages:
We must know in advance that how many elements are to be stored in array.
An array is a static data structure. Memory is allocated during compile time. Once Memory is allocated at compile time it cannot be changed at run-time.
C does not perform bounds checking. Process of checking the extreme limit of the array is called bound checking. In C language there is no way for the programmer to determine the size of an array at runtime and so it becomes necessary for the programmer to keep track of the length of the array.
If a C language program attempts to access an array element outside of the actual allocated memory, then a buffer overflow occurs. typically crashing the program. So the programmer needs to explicitly perform a bounds check before accessing an array.
If the number of elements of an array is less than the allocated size of an array, the memory space be wasted.
Inserting element is very difficult because before inserting an element in an array we have to create empty space by shifting other elements one position ahead.
This operation is faster if the array size is smaller, but the same operation will be more and more time consuming and non-efficient in case of an array with large size.
Deletion is not easy because the elements are stored in a contiguous memory location. Like insertion operation, we have to delete element from the array and after deletion empty space will be created and thus we need to fill the space by moving elements up in the array.
Sample Programs of Passing an array to a function in C
Program 1:
In this passing an array to a function in c program, we have created a function which accepts an integer and prints that integer variable as the output. We have used for loop to iterate an array and pass each array element to the function.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//Learnprogramo #include<stdio.h> void PrintArray(int a) { printf("Array Element = %%d \n", a); } int main() { int array[] = {5, 6, 7, 8, 9, 10}; for (int i = 0; i < 6; i++) { PrintArray(array[i]); } return 0; }</pre> </div>
Output:
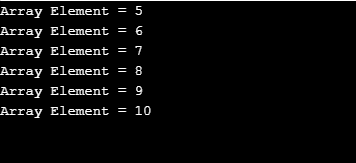
Program 2:
In the above program, we have passed the individual element of array to function. So, instead of passing single elements, we will pass an array to function directly.
<div class="wp-block-codemirror-blocks-code-block code-block"> <pre>//Learnprogramo #include<stdio.h> int SumofNumbers(int a[], int Size) { int Addition = 0; int i; for(i = 0; i < Size; i++) { Addition = Addition + a[i]; } return Addition; } int main() { int i, Size, a[10]; int Addition; printf("Please Enter the Size of an Array: "); scanf("%%d", &Size); printf("\nPlease Enter Array Elements\n"); for(i = 0; i < Size; i++) { scanf("%%d", &a[i]); } Addition = SumofNumbers(a, Size); printf("Sum of All Elements in an Array = %%d \n", Addition); return 0; }</pre> </div>
Output:
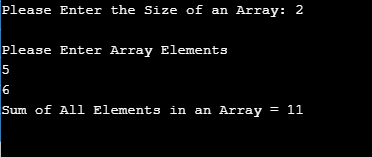
Conclusion:
So in this lesson, we have learned about passing an array to a function in c and also we have ended this lesson. Now, from the next lesson, we will start the next lesson pointers in c.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.
- Multidimensional Array in C.