Table of Contents
What is Two Dimensional Array in C?
An Array Consisting of two subscripts is known as Two Dimensional Array in C. These are often known as array of the array.
Two Dimensional (2D) array is a fixed-length, homogeneous data type, row and column-based data structure which is used to store similar data type element in a row and column-based structure.
A two-dimensional array is referred to as a matrix or a table. A matrix has two subscripts, one denotes the row and another denotes the column.
In other words, a two-dimensional array is an array of a one-dimensional array.
The general syntax for declaration of a 2D array is given below:
data_type array_name[row] [column];
where data_type specifies the data type of the array. array_name specifies the name of the array, row specifies the number of rows in the array and column specifies the number of columns in the array.
The total number of elements that can be stored in a two array can be calculated by multiplying the size of all the dimensions.
For example The array int x[10][20] can store total (10*20) = 200 elements.
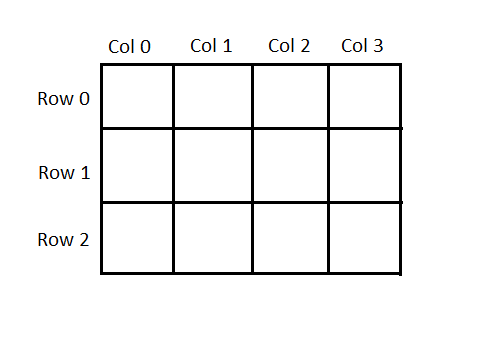
Initialization of Two Dimensional Array in C
Like the one dimensional arrays, two-dimensional arrays may be initialized by following their declaration with a list of initial values enclosed in braces.
Example: int a[2][3]={0,0,0,1,1,1}; initializes the elements of the first row to zero and the second row to one. The initialization is done row by row.
The above statement can also be written as:
int a[2][3]={{0,0,0},{1,1,1}};
By surrounding the elements of each row by braces.
We can also initialize a two dimensional array in c in the form of a matrix as shown below:
int a[2][3]={{0,0,0},{1,1,1}};
When the array is completely initialized with all values, explicitly we need not specify the size of the first dimension.
Example: int a[ ][3]={{0,2,3},{2,1,2}};
If the values are missing in an initializer, they are automatically set to zero.
Example: int a[2][3]={{1,1},{2}};
Will initialize the first two elements of the first row to one, the first element of the second row to two and all other elements to zero.
We can also initialize the array at the run time also using for loop construct as follows:
//Learnprogramo for(i=0;i<3;i++) for(j=0;j<3;j++) scanf("%d",&arr[i][j]);
The above code will initialize 2D array having 3 rows and 3 columns at run time.
Accessing Two Dimensional Array Elements
An element in a two-dimensional array is accessed by using the subscripts i.e., row index and column index of the array.
A two-dimensional array can be seen as a table with ‘x’ rows and ‘y’ columns where the row number ranges from 0 to (x-1) and column number ranges from 0 to (y – 1).
For Example int matrix[3][4];
The two-dimensional array matrix, which contains three rows and four columns can be shown in the below table:
Rows | 0. Column 0 | 1. Column 1 | 2. Column 2 | 3. Column 3 |
---|---|---|---|---|
0. Row 0 | 1. a[0][0] | 2. a[0][1] | 3. a[0][2] | 4. a[0][3] |
1. Row 1 | 5. a[1][0] | 6. a[1][1] | 7. a[1][2] | 8. a[1][3] |
2. Row 2 | 9. a[2][0] | 10. a[2][1] | 11. a[2][2] | 12. a[2][3] |
The above Matrix has 12 integer elements.
The matrix[0][0] element in the first row, first column.
Matrix[2][3] element in the last row, last column.
Matrix is the address of the first element.
A matrix[1] is the address of Row 1.
A matrix[1] is a one-dimensional array (Row1).
For processing 2D arrays, we use two nested for loops. The outer for loop corresponds to the row and the inner for loop corresponds to the column.
Sample Programs
Program 1: Sorting the elements in Matrix and then printing it.
//Learnprogramo #include <stdio.h> void main () { int arr[3][3],i,j; for (i=0;i<3;i++) { for (j=0;j<3;j++) { printf("Enter a[%d][%d]: ",i,j); scanf("%d",&arr[i][j]); } } printf("\n printing the elements ....\n"); for(i=0;i<3;i++) { printf("\n"); for (j=0;j<3;j++) { printf("%d\t",arr[i][j]); } } }
Output:
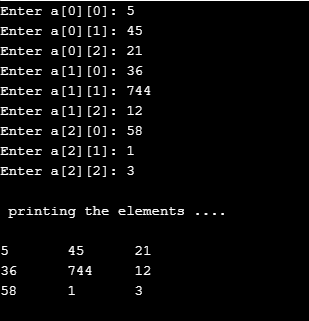
Program 2: Addition of two Matrix Program in C.
//Learnprogramo #include <stdio.h> int main() { float a[2][2], b[2][2], result[2][2]; printf("Enter elements of 1st matrix\n"); for (int i = 0; i < 2; ++i) for (int j = 0; j < 2; ++j) { printf("Enter a%d%d: ", i + 1, j + 1); scanf("%f", &a[i][j]); } printf("Enter elements of 2nd matrix\n"); for (int i = 0; i < 2; ++i) for (int j = 0; j < 2; ++j) { printf("Enter b%d%d: ", i + 1, j + 1); scanf("%f", &b[i][j]); } for (int i = 0; i < 2; ++i) for (int j = 0; j < 2; ++j) { result[i][j] = a[i][j] + b[i][j]; } printf("\nSum Of Matrix:"); for (int i = 0; i < 2; ++i) for (int j = 0; j < 2; ++j) { printf("%.1f\t", result[i][j]); if (j == 1) printf("\n"); } return 0; }
Output:
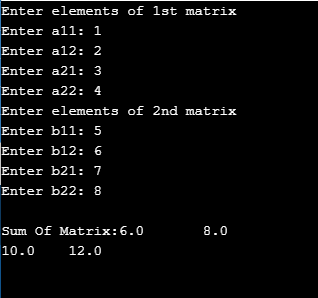
Conclusion:
In this lesson we have learned about two dimensional array in C. Now in the next lesson, we will learn the multidimensional array in C.
Also Read:
- Operators in C.
- Tokens in C.
- History of C Language.
- The algorithm in C.
- If else Statement in C.
- Input-Output Functions in C.
- Storage Classes in C.
- Array in C.