Today we will learn C program to Convert Decimal to Binary and also convert Binary to Decimal number. Before start learning, you should have a little bit of knowledge of Binary and decimal Numbers.
Table of Contents
What is Binary Number?
The Binary Number is number which has base 2. which consists of only two numerical symbols ‘1’ and ‘0’ and each digit is referred to as a ‘Bit’.
For Example 5 in binary can be written as 0101.
What is Decimal Number?
The number which has base 10 is called a Decimal Number system.
For Example 0101=2^3*0+2^2*1+2^1*0+2^0*1=0+4+0+1=5.
There are so many ways to convert Decimal to binary and vice versa. we will see it one by one.
1. Program to Convert decimal to Binary
In this program, we will ask user to enter the number which the users want to convert it into Binary.
Decimal to Binary Conversion Algorithm:
1 Step: At first divide the number by 2 by using modulus operator ‘%’ and store the remainder in the array.
2 Step: divide that number by 2.
3 Step: Now repeat the step until the number is greater than 0.
#include <stdio.h> int main() { int a[10], number, i, j; printf("\n Please Enter the Number You want to Convert : "); scanf("%d", &number); for(i = 0; number > 0; i++) { a[i] = number % 2; number = number / 2; } printf("\n Binary Number of a Given Number = "); for(j = i - 1; j >= 0; j--) { printf(" %d ", a[j]); } printf("\n"); return 0; }
Output:
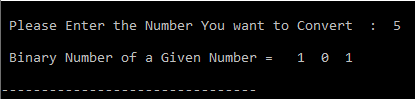
Explanation:
Let us consider the input number as 5. At first, the value of i is 0.
1st Iteration: for(i=0;number>0;i++) i.e (i=0;5>0;0++) conition is true and a[0]=number%2=5%2=1. Therefore,number=number/2=2.5.
2nd Iteration: for(i=0;number>0;i++) i.e (i=1;2>0;1++) conition is true and a[1]=number%2=2.5%2=0. Therefore,number=2.5/2=1.25.
3rd Iteration: for(i=0;number>0;i++) i.e (i=2;1>0;2++) conition is true and a[2]=number%2=1.25%2=1. Therefore,number=number/2=0.
In this program, we have used for loop.
2. C Program to Convert Binary to Decimal
In this program, we will convert the Binary input to Decimal.
Binary to Decimal Conversion Algorithm:
1 Step: Take a Binary Input to convert into Decimal.
2 Step: Multiply each binary digit starting from last with the power of 2 i.e(2^0,2^1).
3 Step: make an addition of the multiplied digits.
4 Step: Thus the addition gives the Decimal output.
#include <math.h> #include <stdio.h> int convert(long long n); int main() { long long n; printf("Enter a binary number: "); scanf("%lld", &n); printf("%lld in binary = %d in decimal", n, convert(n)); return 0; } int convert(long long n) { int dec = 0, i = 0, rem; while (n != 0) { rem = n % 10; n /= 10; dec += rem * pow(2, i); ++i; } return dec; }
Output:

3. C program to Convert Decimal to Binary Using While loop
In this program, we have used while loop instead of for loop but the logic behind the conversion is the same.
#include <stdio.h> int main() { int a[10], number, i = 1, j; printf("\n Please Enter the Number You want to Convert : "); scanf("%d", &number); while(number != 0) { a[i++] = number % 2; number = number / 2; } printf("\n Binary Number of a Given Number = "); for(j = i - 1; j > 0; j--) { printf(" %d ", a[j]); } return 0; }
Output:
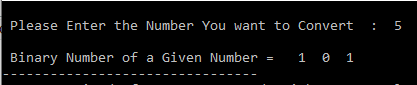
4. Decimal to Binary Using Function
In this program, we will use the user-defined function and Bitwise AND operator to convert a decimal number into binary.
#include <stdio.h> void Decimal_to_Binary(int number) { int j; for(int i = 31; i >= 0; i--) { j = number >> i; if(j & 1) printf("1"); else printf("0"); } } int main() { int number; printf("\n Please Enter the Number You want to Convert : "); scanf("%d", &number); Decimal_to_Binary(number); return 0; }
Output:
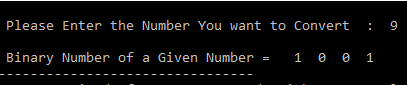
In the above program, we have used a user-defined function Decimal_to_Binary.
Also Read:
- C Program to print Prime Numbers from 1 to n
- C Program to Make Simple Calculator
- Armstrong Number Program in C
- Fibonacci Series Program in C
- Reverse a String in C
- Program to Reverse a Number in C
- Hello World Program in C
- Palindrome Program in C
- Leap Year Program in C
- Factorial Program in C
- Prime Number Program in C