Today we will learn C++ program to reverse a string and also how to write a program to Reverse a String in C++.
Table of Contents
What is reversing a String?
Reversing a string means changing the positions of the characters in such a way that the last character comes in the first position, second last on the second position and so on.
There are so many ways to reverse a string we will see it one by one.
1. C++ Program to Reverse a String Using Built-in Function
In this program, we will use inbuilt library function called as reverse[begin..end] which reverses the string and print that string on the screen.
//Learnprogramo - programming made simple #include <iostream> #include<bits/stdc++.h> using namespace std; int main() { string greeting = "Learnprogramo"; //Note that it takes the iterators to the start and end of the string as arguments reverse(greeting.begin(),greeting.end()); cout<<greeting<<endl; }
Output:

2. Reverse a String Using for loop
In this program, we will use for loop to reverse a string.
//Learnprogramo - programming made simple #include <iostream> #include <string> using namespace std; // Function to reverse a string string reverse(string const &s) { string rev; for (int i = s.size() - 1; i >= 0; i--) { rev = rev.append(1, s[i]); }; return rev; } // main function int main() { std::string s("Learnprogramo programming made simple"); s = reverse(s); cout << s; return 0; }
Output:

In the above program, we have given input string as “Learnprogramo programming made simple”. After executing the compiler will print the reverse of the input string.
3. Reverse a String Using While Loop
In this program, we will use while loop to do the reverse of a string. After executing this program the compiler will ask the user to enter the string that user want to make the reverse of. After giving input the compiler will print output on the screen.
//Learnprogramo - programming made simple #include<iostream> using namespace std; #include<cstring> int main() { char str[100], temp; int i=0, j; cout<<"Enter the String : "; gets(str); j=strlen(str)-1; while(i<j) { temp=str[i]; str[i]=str[j]; str[j]=temp; i++; j--; } cout<<"Reverse of the String = "<<str; }
Output:

4. C++ Program to Reverse a String Using Recursion
The program is said to be recursive if and only if the function can call itself directly or indirectly. In this program, we will declare a recursive function which can reverse an input string.
//Learnprogramo - programming made simple #include <iostream> using namespace std; void reverse(const string& a); int main() { string str; cout << " Please enter a string " << endl; getline(cin, str); reverse(str); return 0; } void reverse(const string& str) { size_t numOfChars = str.size(); if(numOfChars == 1) cout << str << endl; else { cout << str[numOfChars - 1]; reverse(str.substr(0, numOfChars - 1)); } }
Output:
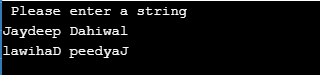
In the above program, the reverse() function is recursive. This function prints the last character of the string (numofChars-1). Every array starts with zero so we have use -1.
substr() gives the string up to second last character and it is again passed to the reverse() function.
When the reverse() function is called again then second last character is printed because the string contains one character less than from the last.
Now one character from the last is cut off from the string again and then passed to the reverse() function.
This process continues until the length of the string equal to 1 when the final character is printed and at last the loop ends.
5. Using function
In this program, we will declare user-defined function to reverse a string.
//Learnprogramo - programming made simple #include <iostream> using namespace std; void reverse_String(string& greet, int n,int i) { if(n==i){return; } swap(greet[i],greet[n]); reverse_String(greet,n-1,i+1); } int main() { string greeting = "Learnprogramo"; cout<<"String before reversal: "<<greeting<<endl; reverse_String(greeting,greeting.length()-1,0); cout<<"String after reversal: "<<greeting<<endl; }
Output:

In the above program, we have declared user-defined function reverse_String() to reverse a string.
6. Reverse a String in C++ Using Iterators
The unique way to loop through the characters of a string to backwards is by using reverse iterators. The iterators are of type read-only so it is must use const reverse iterators which are returned by std::string::crbegin and std::string::crend.
std::string::crbegin points to the last character of the string and std::string::crend which is points to the first character of the string.
//Learnprogramo - programming made simple #include <iostream> #include <string> using namespace std; // Function to reverse a string string reverse(string const &s) { string rev; std::string::const_reverse_iterator it = s.crbegin(); while (it != s.crend()) { rev = rev.append(1, *(it++)); }; return rev; } // main function int main() { std::string s("Jai Bajrangbali"); s = reverse(s); cout << s; return 0; }
Output:

Also Read:
- Prime number program in C++.
- Factorial Program in C++.
- Program to add two numbers in C++.
- Palindrome Program in C++.
- Hello World Program in C++.
- Leap year program in C++.
- C++ Program to Reverse a Number.
- Decimal to Binary and Vice Versa in C++.
- Prime numbers Between 1 to n in C++.