Today we will learn C++ program for prime number between 1 to n. So before starting you should have a little bit of knowledge about prime numbers.
Table of Contents
What are Prime Numbers?
Any natural number which can be divisible by itself or the number which has only two factors i.e 1 and the number itself are called prime numbers.
For example 2,3,5,7,11 and so on…
Note: The number 2 is only even prime number because most of the numbers are divisible by 2.
There are different methods to print prime numbers from 1 to n. We will see it one by one.
1. C++ Program for Prime Number Between 1 to n
In this program, we will ask the user to enter the starting and the ending number so that the compiler will fetch and prints all the prime numbers between those intervals.
//Learnprogramo - programming made simple #include <iostream> using namespace std; int main() { int low, high, i, flag; cout << "Enter two numbers(intervals): "; cin >> low >> high; cout << "Prime numbers between " << low << " and " << high << " are: "; while (low < high) { flag = 0; for(i = 2; i <= low/2; ++i) { if(low % i == 0) { flag = 1; break; } } if (flag == 0) cout << low << " "; ++low; } return 0; }
Output:
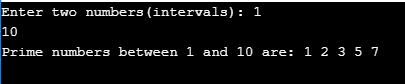
In the above program, the lower interval is assigned to variable low and the highest interval is assigned to the high. So in the output, we have given low as 1 and the high as 10 and the compiler have printed all the prime numbers between 1 and 10.
2. C++ Program for Prime Number Between 1 to n Using Functions
In this program, we will declare a user-defined function to print all the prime numbers between 1 and n.
//Learnprogramo - programming made simple #include <iostream> using namespace std; int isPrimeNumber(int); int main() { bool isPrime; int count; cout<<"Enter the value of n:"; cin>>count; for(int n = 2; n < count; n++) { // isPrime will be true for prime numbers isPrime = isPrimeNumber(n); if(isPrime == true) cout<<n<<" "; } return 0; } // Function that checks whether n is prime or not int isPrimeNumber(int n) { bool isPrime = true; for(int i = 2; i <= n/2; i++) { if (n%i == 0) { isPrime = false; break; } } return isPrime; }
Output:

After compiling the above program we have given the input n as 15 so the compiler has printed all the prime numbers up to 15.
3. Print Prime Numbers When Greatest Number is Entered first
In this program, we will first give inputs lowest and greatest number between which we want to print all prime numbers.
//Learnprogramo - programming made simple #include <iostream> using namespace std; int main() { int low, high, flag, temp; cout << "Enter two numbers(intevals): "; cin >> low >> high; //swapping numbers if low is greater than high if (low > high) { temp = low; low = high; high = temp; } cout << "Prime numbers between " << low << " and " << high << " are: "; while (low < high) { flag = 0; for(int i = 2; i <= low/2; ++i) { if(low % i == 0) { flag = 1; break; } } if (flag == 0) cout << low << " "; ++low; } return 0; }
Output:

4. Printing all Prime Numbers Between 1 and 100
In this program we will print all the prime numbers between 1 and 100.
//Learnprogramo - programming made simple #include <iostream> using namespace std; int isPrimeNumber(int); int main() { bool isPrime; for(int n = 2; n < 100; n++) { // isPrime will be true for prime numbers isPrime = isPrimeNumber(n); if(isPrime == true) cout<<n<<" "; } return 0; } // Function that checks whether n is prime or not int isPrimeNumber(int n) { bool isPrime = true; for(int i = 2; i <= n/2; i++) { if (n%i == 0) { isPrime = false; break; } } return isPrime; }
Output:

Also Read:
- Prime number program in C++.
- Factorial Program in C++.
- Program to add two numbers in C++.
- Palindrome Program in C++.
- Hello World Program in C++.
- Leap year program in C++.
- C++ Program to Reverse a Number.
- Decimal to Binary and Vice Versa in C++.
- Reverse a String in C++.
- Bubble Sort Program in C++.