Today we will learn leap year program in C++ and how to write a program in C++ to check leap year. So before start learning, you should have a little bit of knowledge of leap year. So,
Table of Contents
What is Leap Year?
Generally, a normal year has 365 days but, a leap year has 366 days i.e 1 day extra in the month of February. The Leap year occurs every 4 years.
Logic: The year which is divisible by 4, and 400 is called a leap year.If year divisible by 4 but not divisible by 100 is also called a leap year.
The year not divisible by 4 and 100 but divisible by 400 is not a leap year.
There are many ways to check whether the year is a leap year or not. So, we will see one by one.
1. Leap Year Program in C++
In this program, the compiler will ask the user to enter any year to check whether the given year is a leap year or not. After entering the year the compiler will check and print the output on the screen.
//learnprogramo - Programming made simple #include <iostream> using namespace std; int main() { int year; cout << "Enter a year: "; cin >> year; if (year % 4 == 0) { if (year % 100 == 0) { if (year % 400 == 0) cout << year << " is a leap year."; else cout << year << " is not a leap year."; } else cout << year << " is a leap year."; } else cout << year << " is not a leap year."; return 0; }
Output:
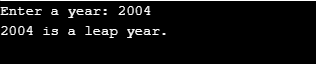
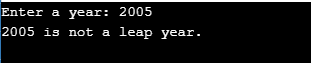
Condition 1st:(year%4) if the condition is false the given number is not leap year but the condition is true then we have to check for century year. So the compiler goes to the next condition.
Condition 2nd:(year%400) if the condition is false the year is not a leap. But, if the condition is true then the compiler will jump to the next condition.
Condition 3rd: This is the final condition which decides whether the entered year is a leap year or not. If (year%100!=0) then the year is a leap year, else year is not a leap.
2. Leap Year Program in C++ in Single Line
In this Program, we will check whether the year is a leap year or not using Logical And and Logical OR Operators.
//Learnprogramo - Programming made simple #include <iostream> using namespace std; int main() { int year; cout << "Enter a year you want to check: "; cin >> year; //condition if ((year % 4 == 0) && (year % 100 != 0) || (year % 400 == 0)) cout << year << " is a leap year." << endl; else cout << year << " is not a leap year." << endl; return 0; }
Output:
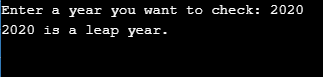
Program Logic:
In above program we have used a logic in if statement i.e (((year%4==0)&&(year%100!=0))||(year%400==0)).Here “||” is logical OR and “&&” is logical AND.
Our condition is (year%400==0).This condition will check whether the remainder is 0 or not. In the first condition (year%4) also check whether the remainder is 0 or not and if the condition gets FALSE then the program will exit.
Second Condition (year%100!=0).In this condition, if the remainder is not equal to 0 then it is a leap year. In Leap year definition we have defined that if the year which is divisible by 4 and 400 but not 100 is Leap Year.
3. Program in C++ to Check Leap Year Using Function
In this program, we will declare a user-defined function to check whether the given year is a leap year or not.
//Learnprogramo - Programming made simple #include <iostream> using namespace std; bool leapYear(int y); int main(){ int y; cout<<"Enter year: "; cin>>y; //function call bool flag = leapYear(y); if(flag == true) cout<<y<<" is a leap Year"; else cout<<y<<" is not a leap Year"; return 0; } bool leapYear(int y){ bool isLeapYear = false; if (y % 4 == 0) { if (y % 100 == 0) { if (y % 400 == 0) { isLeapYear = true; } } else isLeapYear = true; } return isLeapYear; }
Output:

In the above program, we have declared a user-defined function leapYear() which check for leap year.
When the compiler starts executing the program as it reaches to the line bool flag=leapYear(y) then the compiler directly jumps to the function bool leapyear(int y).
4. Using else-if Statements
//Learnprogramo - programming made simple #include<iostream> using namespace std; int main() { int yr; cout<<"Enter year :"; cin>>yr; if((yr%4==0) && (yr%100!=0)) { cout<<"This is a Leap Year"; } else if((yr%100==0) && (yr%400==0)) { cout<<"This is a Leap Year"; } else if(yr%400==0) { cout<<"This is a Leap Year"; } else { cout<<"This is not a Leap Year"; } }
Output:

Also Read:
- Prime number program in C++.
- Factorial Program in C++.
- Leap Year Using C
- Program to add two numbers in C++.
- Palindrome Program in C++.
- Hello World Program in C++.
- Program to reverse a number in C++.