Today we will learn the Factorial Program in C++ and how to find factorial of a given number using recursion. So before start learning, you should have a little bit of knowledge of factorial of a number. So first of all,
Table of Contents
What is Factorial of a Number?
The Factorial of a Number n is the multiplication of all integers less than or equal to n. Factorial of n is represented by n!.
For Example:
Factorial of 5 is 5!=5*4*3*2*1 which is equal to 120.
Note: 5! here ‘!’ is also called as factorial, bang or shriek.
There are so many ways to find factorial of the number we will see it one by one.
1. Factorial Program in C++ Using for loop
In this program, the compiler will ask the user to enter the number which user want to find the factorial of. After entering the number compiler will print the factorial of that number on the screen using for loop.
#include <iostream> using namespace std; int main() { unsigned int n; unsigned long long fact = 1; cout << "Enter a positive integer to find factorial: "; cin >> n; for(int i = 1; i <=n; ++i) { fact *= i; } cout << "Factorial of " << n << " = " << fact; return 0; }
Output:

Here the factorial of number may be large so we have used unsigned long long.
Logic:
1st Iteration: Here at first i=1,fact=1 and n=5 i.e(i<=n).fact=fact*i i.e fact=1*1=1.now i is incremented,so i becomes 2.
2nd Iteration: Here i=2,fact=1 and n=5 i.e(i<=n).fact=fact*i i.e fact=1*2=2.now i is incremented,so i becomes 3.
3rd Iteration: Here i=3,fact=2 and n=5 i.e(i<=n).fact=fact*i i.e fact=2*3=6.now i is incremented,so i becomes 4.
4th Iteration: Here i=4,fact=6 and n=5 i.e(i<=n).fact=fact*i i.e fact=6*4=24.now i is incremented,so i becomes 5.
5th Iteration: Here i=5,fact=24 and n=5 i.e(i<=n).fact=fact*i i.e fact=24*5=120.now i is incremented,so i becomes 5.
6th Iteration: now i is incremented to 6 which is greater than n 6>5 i.e(i>n) so the program is terminated and the answer is 120.
2. Factorial Program in C+ + Using Recursion
It is the method in which the function calls itself directly or indirectly. Recursion consists of two main conditions i.e base condition and the recursive call.
#include<iostream> using namespace std; int main() { int factorial(int); int fact,value; cout<<"Enter any number: "; cin>>value; fact=factorial(value); cout<<"Factorial of a number is: "<<fact<<endl; return 0; } int factorial(int n) { if(n<0) return(-1); /*Wrong value*/ if(n==0) return(1); /*Terminating condition*/ else { return(n*factorial(n-1)); } }
Output:
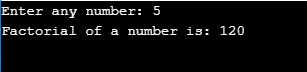
In Above program int factorial(int) is function prototype and in printf,factorial(value) function call is made.
If the user enters 5 as the input then the call to the function factorial(5) is made and 5 which is not equal to 0. So the flow goes to the else statement where return call is made to the factorial(4).
This process continues till the 1 is returned and thus we get the factorial of 5 i.e 120.
3. C++ Program to find Factorial of a Number Using While Loop
It is the same program as a program of for loop.only difference is that we use while loop instead of while loop but the logic is the same.
#include <iostream> using namespace std; unsigned int factorial(unsigned int n) { int i = n, fact = 1; while (n / i != n) { fact = fact * i; i--; } return fact; } int main() { int num = 5; cout << "Factorial of " << num << " is " << factorial(num) << endl; return 0; }
Output:
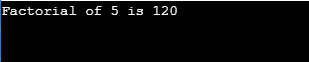
Time Complexity of the above solution is O(n).
4. C++ Program to Find Factorial Using do while Loop
In this program, we will ask the user to enter the number which user want to find factorial of that number.
#include<iostream> #include<conio.h> using namespace std; int main() { long n, i, fact=1; cout<<"Enter any num: "; cin>>n; i=n; if(n>=0) { do { fact=fact*i; i--; } while(i>0); cout<<"\nFactorial: "<<fact; } else { cout<<"fact of -ve num. is not possible"; } }
Output:
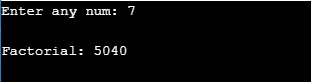
5. Factorial Program in C++ Using Functions
This is the modular approach of the program. If we want to change in the code we need not change the whole code, instead, we will do change in function.
#include<iostream> using namespace std; int factorial(int n); int main() { int num, fact_num = 1; cout << "Enter random number to find the factorial: "; cin >> num; cout <<"Factorial of the given number is "<< factorial(num); return 0; } int factorial(int n) { int count_num, result = 1; for (count_num = 1; count_num <= n; count_num ++) result = result * count_num; return result; }
Output:

6. Using if-else Statement
In this program, we will use the if-else statement to find the factorial of a given number.
#include<iostream> using namespace std; int main() { int num, i, fact_num = 1; cout << "Enter the positive number to find the factorial: "; cin >> num; // if entered number is negative show the error if (num< 0) cout << "Error! You have entered negative number and Factorial for negative number does not exist."; else { for(i = 1; i <= num; ++i) { fact_num*= i; // factorial = factorial*i; } cout << "Factorial of the entered number is " << fact_num; } return 0; }
Output:

In the above program, we have initialized the tree variable num, I and fact_num. So to calculate the factorial if-else statement is used. If the user enters the negative number then the compiler will show an error “You have entered the negative number”.
If the number is positive then control will go to else statement.
Also Read: