Today we will learn C++ Program to Add Two Numbers and also learn Program to Add Two Numbers in C++.
The Addition is the Arithmetic Operation in which the sum of two numbers is performed.
For Example, The addition of 5 and 6 is 11.
Table of Contents
Algorithm of Adding two Numbers
1 Step: START.
2 Step: Initialize integers A, B and C.
3 Step: Accept two integers A and B from User.
3 Step: Now do the operation using formula C=A+B.
4 Step: Now the addition is stored in C Variable.
5 Step: Print C.
6 Step: STOP.
Now we will do this arithmetic operation in C++. There are different methods to make an Addition in C++ we will see it one by one.
1. C++ Program to Add Two Numbers
In this program, we will ask the user to enter the two numbers and do addition using formula. This addition will store in the third Variable and at the end, the value stored in the third variable is printed.
#include <iostream> using namespace std; int main() { int firstNumber, secondNumber, sumOfTwoNumbers; cout << "Enter two integers: "; cin >> firstNumber >> secondNumber; // sum of two numbers in stored in variable sumOfTwoNumbers sumOfTwoNumbers = firstNumber + secondNumber; // Prints sum cout << firstNumber << " + " << secondNumber << " = " << sumOfTwoNumbers; return 0; }
Output:
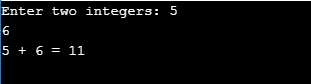
In the above program, when the compiler asks the user to enter two integers, the two integers are stored in the variables firstNumber and secondNumber.
These two integers are added using + operator and their addition is stored in the third variable sumOfTwoNumbers.
Finally, the output is printed on the screen.
2. Addition Using Class and Function in C++
In this program we will do addition of two numbers using class and declare function for addition.
#include <iostream> using namespace std; class Add{ public: int num1, num2; void ask(){ cout<<"Enter the first number: "; cin>>num1; cout<<"Enter the second number: "; cin>>num2; } int sum(int n1, int n2){ return n1+n2; } //This function displays the addition result void show(){ cout<<sum(num1, num2); } }; int main(){ //Creating object of class Add Add obj; //asking for input obj.ask(); //Displaying the output obj.show(); return 0; }
Output:
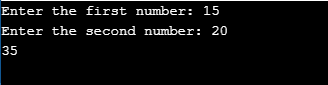
Note: In the above program the sum() function from the main is not called because the sum() function is called from the show() function.
3. C++ Program to Add Two Numbers Using Function Overloading
The Function Overloading is an ability to create multiple functions which can have the same name but with the different implementations. Function Overloading is also called as Method Overloading.
In this program, we will declare three functions with the same name but their operations will be different.
#include <iostream> using namespace std; int sum(int, int); float sum(float, float); float sum(int, float); int main(){ int num1, num2, x; float num3, num4, y; cout<<"Enter two integer numbers: "; cin>>num1>>num2; //This will call the first function cout<<"Result: "<<sum(num1, num2)<< endl; cout<<"Enter two float numbers: "; cin>>num3>>num4; //This will call the second function cout<<"Result: " <<sum(num3, num4)<< endl; cout<<"Enter one int and one float number: "; cin>>x>>y; //This will call the third function cout<<"Result: " <<sum(x, y)<< endl; return 0; } int sum(int a, int b){ return a+b; } float sum(float a, float b){ return a+b; } float sum(int a, float b){ return a+b; }
Output:
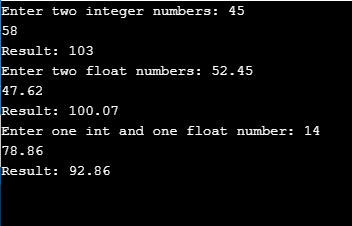
4. C++ Addition Program Using Class
In this program, we will declare one class to do the addition of two integers.
#include <iostream> using namespace std; class Maths { int x, y; public: void input() { cout << "Input two integers\n"; cin >> x >> y; } void add() { cout << "Result: " << x + y; } }; int main() { Maths m; m.input(); m.add(); return 0; }
Output:
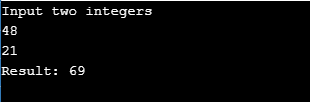
In the above program, we have declared class Maths to perform the addition of two numbers.
Also Read:
- Prime number Program in C++.
- Factorial Program in C++.
- Program to add two numbers in C.
- Palindrome Program in C++.