Today we will learn Program to Convert Decimal to Binary in C++ and also convert Binary to Decimal number. Before start learning, you should have a little bit of knowledge of Binary and decimal Numbers.
Table of Contents
What is Binary Number?
The Binary Number is number which has base 2. which consists of only two numerical symbols ‘1’ and ‘0’ and each digit is referred to as a ‘Bit’.
For Example 5 in binary can be written as 0101.
What is Decimal Number?
The number which has base 10 is called a Decimal Number system.
For Example 0101=2^3*0+2^2*1+2^1*0+2^0*1=0+4+0+1=5.
There are so many ways to convert Decimal to binary and vice versa. we will see it one by one.
1. Program to convert Decimal to Binary in C++
In this program, we will ask the user to enter the number which user wants to convert into binary.
Decimal to Binary Conversion Algorithm:
1 Step: At first divide the number by 2 by using modulus operator ‘%’ and store the remainder in the array.
2 Step: divide that number by 2.
3 Step: Now repeat the step until the number is greater than 0.
//Learnprogramo - programming made simple #include <iostream> #include <cmath> using namespace std; long long convertDecimalToBinary(int); int main() { int n, binaryNumber; cout << "Enter a decimal number: "; cin >> n; binaryNumber = convertDecimalToBinary(n); cout << n << " in decimal = " << binaryNumber << " in binary" << endl ; return 0; } long long convertDecimalToBinary(int n) { long long binaryNumber = 0; int remainder, i = 1, step = 1; while (n!=0) { remainder = n%2; cout << "Step " << step++ << ": " << n << "/2, Remainder = " << remainder << ", Quotient = " << n/2 << endl; n /= 2; binaryNumber += remainder*i; i *= 10; } return binaryNumber; }
Output:
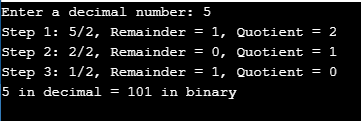
2. Program to Convert Binary to Decimal in C++
In this program, we will convert the Binary input to Decimal.
Binary to Decimal Conversion Algorithm:
1 Step: Take a Binary Input to convert into Decimal.
2 Step: Multiply each binary digit starting from last with the power of 2 i.e(2^0,2^1).
3 Step: make an addition of the multiplied digits.
4 Step: Thus the addition gives the Decimal output.
//Learnprogramo - programming made simple #include <iostream> #include <cmath> using namespace std; int convertBinaryToDecimal(long long); int main() { long long n; cout << "Enter a binary number: "; cin >> n; cout << n << " in binary = " << convertBinaryToDecimal(n) << "in decimal"; return 0; } int convertBinaryToDecimal(long long n) { int decimalNumber = 0, i = 0, remainder; while (n!=0) { remainder = n%10; n /= 10; decimalNumber += remainder*pow(2,i); ++i; } return decimalNumber; }
Output:
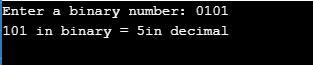
3. Decimal to Binary Conversion Using Bitwise Operator
//Learnprogramo - programming made simple #include <iostream> using namespace std; int decToBinary(int n) { for (int i = 31; i >= 0; i--) { int k = n >> i; if (k & 1) cout << "1"; else cout << "0"; } } int main() { //size of integer asumed as 32 bits int n = 32; decToBinary(n); }
Output:

4. Decimal to Binary Without Using Array
//Learnprogramo - programming made simple #include <cmath> #include <iostream> using namespace std; #define ull unsigned long long int int decimalToBinary(int N) { ull B_Number = 0; int cnt = 0; while (N != 0) { int rem = N % 2; ull c = pow(10, cnt); B_Number += rem * c; N /= 2; cnt++; } return B_Number; } int main() { int N = 15; cout << decimalToBinary(N); return 0; }
Output:

Also Read:
- Prime number program in C++.
- Factorial Program in C++.
- Program to add two numbers in C++.
- Palindrome Program in C++.
- Hello World Program in C++.
- Leap year program in C++.
- C++ Program to Reverse a Number.
- Reverse a String Program in C++.