Table of Contents
What is Pointer Arithmetic in C?
A Pointer Arithmetic in C is very important where we can perform addition, subtraction etc on pointers in c.
The C language allows five Pointer arithmetic in C operations to be performed on pointers.
- Increment ++
- Decrement —
- Addition +
- Subtraction –
- Differencing
1. Increment
When a pointer is incremented using ++, the address in the pointer increments by sizeof(datatype). The new value in the pointer will be:
(current address in pointer) + sizeof(data_type)
Example:
Incrementing a pointer to an int will cause its value to be incremented by sizeof(int). Similarly, a pointer to a float will be incremented by sizeof(float).
For Example:
int n=20; int *ptr=&n;
n=20 and ptr=1000 after ptr++ value of ptr=1002
//Learnprogramo #include<stdio.h> int main(){ int number=50; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p+1; printf("After increment: Address of p variable is %u \n",p); return 0; }
Output:

The same concept applies for decrementing a pointer, i.e., if a pointer is decremented, it is decreased by sizeof(datatype).
2. Decrement
When a pointer is incremented using –, the address in the pointer decrements by sizeof(datatype). The new value in the pointer will be:
(current address in pointer) – sizeof(data_type)
Example:
decrementing a pointer to an int will cause its value to be decremented by sizeof(int). Similarly, a pointer to a float will be decremented by sizeof(float).
For Example:
int n=20; int *ptr=&n;
n=20 and ptr=1000 after ptr– value of ptr=998
//Learnprogramo #include <stdio.h> void main(){ int number=50; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p-1; printf("After decrement: Address of p variable is %u \n",p); }
Output:

3. Addition
C allows integers to be added to or subtracted from pointers. When a pointer is incremented by integer i, the new value will be,
(current address in pointer) + i * sizeof(data_type)
Example:
int *ptr, n=20; ptr=&n; ptr=ptr+3
This code will increment the address in ptr by 3*sizeof(int). If the sizeof(int) is 2, then the pointer will increment by 6.
n=20 and ptr=1000 after ptr=ptr+3 value of ptr becomes 1006.
//Learnprogramo #include<stdio.h> int main(){ int number=50; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p+3; printf("After adding 3: Address of p variable is %u \n",p); return 0; }
Output:

Subtraction is performed in the same way.
4. Subtraction
C allows integers to be added to or subtracted from pointers. When a pointer is decremented by integer i, the new value will be,
(current address in pointer) – i * sizeof(data_type)
Example:
int *ptr, n=20; ptr=&n; ptr=ptr-3
This code will increment the address in ptr by 3*sizeof(int). If the sizeof(int) is 2, then the pointer will decrement by 6.
n=20 and ptr=1000 after ptr=ptr+3 value of ptr becomes 994.
//Learnprogramo #include<stdio.h> int main(){ int number=50; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p-3; printf("After subtracting 3: Address of p variable is %u \n",p); return 0; }
Output:

5. Differencing
Differencing is the subtraction of two pointers. The subtraction of two pointers gives the number of elements between the two pointers. The result is an integer which is:
(address in pointer1 = address in pointer2) / sizeof(data_type)
Example:
int a[5] = {10,20,30,40,50};
int *p, *q;
p=&a[0]; q=&a[3];
printf(“%d”, q-p);
The output of q-p will be (1006-1000)/sizeof(int) i.e 6/2=3.
//Learnprogramo #include<stdio.h> int main() { int a = 7, b ; int *p; p = &a; b = *p; }
Relationship Between Array and Pointer Arithmetic in C
Arrays and pointers are very closely related. All arrays are implemented as pointers in ‘C’. The array name is a constant pointer and it stores the base address of the array.
int a[5];
Here, a is the array name and it is also a pointer. It stores the base address of the array i.e. &a[0].
An array operation is converted to corresponding pointer operation. The C compiler internally converts a subscripted notation a[i] to the form *(a+i).
a[i]=*(a+i)
Consider the example:
int a[5]={1,2,3,4,5};
printf(“%d”,a[2]);
Here, a[2] is converted to *(a+2) i.e *(9800+2)=*(9804)=2.
printf(“%d”,2[a]);
This statement is also valid since:
a[i]->*(a+i)->*(i+a)->i[a]
Similarly, a 2D array, an element a[i][j] can also be accessed using a pointer notation.
a[i][j]->*(a[i]+j)->*(*(a+i)+j
1. Pointer to Array
To make a pointer point to an array, it must be assigned the base address of the array. The array name contains the base address.
Syntax:
pointer = arrayname; OR pointer = &arrayname[0];
Example:
int a[5], *ptr; ptr=a;
To access array elements using the pointer, the * operator can be used. Array elements can be accessed using a pointer in two ways:
Method 1:
Initialize pointer with the base address of the array and access each element using *pointer.
Method 2:
Initialize pointer with the base address of an array and Use i as an index for array elements.
In the method first, the pointer moves from one element to the next till it reaches the end of the array. In the method second, the pointer remains at the first element and the index changes.
The following program shows array elements are accessed using a pointer.
//Learnprogramo #include<stdio.h> void main() { int a[]={10,20,30,40,50}; int *ptr,i; ptr=a; for(i=0;i<5;i++,ptr++) { printf("\naddress=%u\n",&a[i]); printf("elements=%d%d",a[i],*ptr); } }
Output:
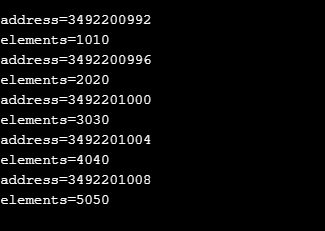
2. Array of Pointers
Just like we can have an array of any built-in data type like int, float, etc. We can create an array of pointers. In an array of pointers, each element is a pointer which stores an address.
Syntax:
data_type *arrayname[size];
Example:
int *ptr[3];
p is an array of 3 integer pointers p[0] to p[2]. Each pointer is assigned an address of an integer variable.
For Example:
int a,b,c;
A ptr[0]=&a; ptr[1]=&b; ptr[2]=&c;
The pointers can be assigned addresses of array elements. In the following example, each pointer in the array is assigned the address of an array element.
int arr[3] = {1,2,3}; int *ptr[3];
ptr[0]=&arr[0]; ptr[1]=&arr[1]; ptr[2]=&arr[2];
To access the value at the address in the pointer, the indirection(*) operator must be used with the pointer.
In the following program, we have an array of three pointers which are assigned the addresses of three integer variables.
//Learnprogramo #include<stdio.h> main() { int arr[3]={1,2,3}; int i,*ptr[3]; for(i=0;i<3;i++) ptr[i]=&arr[i]; for(i=0;i<3;i++) printf("\n address=%u value=%d", ptr[i], *ptr[i]); }
Output:
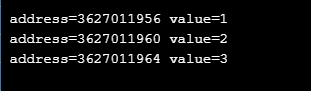
3. Initializing Array of Pointers
An array of pointers can be initialized using the following syntax:
Syntax:
data_type *arrayname[size]= {address1, address2,….};
Example:
int a[]={0,1,2,3,4}; int *p[]={a, a+1, a+2, a+3, a+4};
for(i=0;i<5;i++) printf(“%d”,*p[i]);
An array of Pointer arithmetic in c with Multidimensional Array
A multidimensional array is an array of arrays. A 2-D array consists of multiple 1-D arrays.
For Example: int a[10][20];
Here, a is a two-dimensional array with 10 rows and 20 columns. We can think of array a as 10 arrays, each containing 20 elements. The 10 arrays are a[0] to a[9].
The name of each array a[0] to a[9] is a pointer to the array and stores the base address. This base address can be assigned to a pointer.
Since there are multiple arrays, we need multiple pointers. Hence, we need an array of pointers.
int a[3][2]={1,2,3,4,5,6}; int *ptr[]={a[0],a[1],a[2]};
Here, ptr is an array of pointers. It can also be initialized as follows: int *ptr[] = {a,a+1,a+2};
Each element of the array can be accessed as:
for(i=0;i<3;i++)
for(j=0;j<2;j++)
printf(“%d”, *ptr[i]+j);
Conclusion:
In this lesson, we have learned about pointer arithmetic in c. Now, in the next lesson, we will learn How to declare function pointer in c.