In this lesson, we will learn different types of loops in c such as For loop in c, While loop in C and do while loop in c.
Table of Contents
What is Loop in C?
Loops in C means a sequence of statements is executed until some conditions for termination of the loop are satisfied.
A program loop consists of two segments namely, Body of the loop, and Control statement.
The control statement tests certain conditions & then directs the repeated execution of the statements contained in the body of the loop.
Depending on the position of the control statement in the loop, a control structure may be classified either as the entry controlled loop or exit controlled loop.
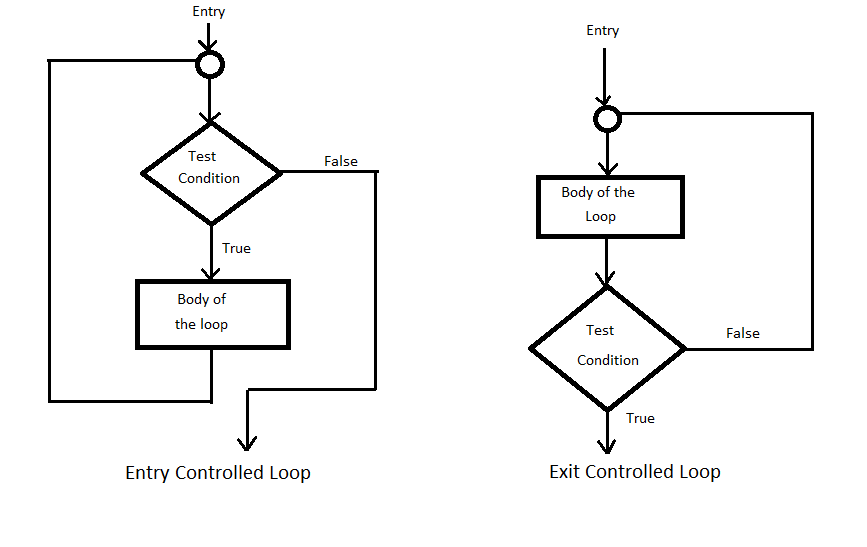
1. Entry Controlled Loop
In the entry controlled loops in C, conditions are tested before the start of the loop execution. If the conditions are satisfied, then the body of the loop will be executed. It is also known as top tested loop.
While loop is an example of entry controlled loop.
2. Exit Controlled Loop
In the case of an exit-controlled loop, the test is performed at the end of the body of the loop execution. If the conditions are not satisfied, then the body of the loop will not be executed. It is also known as bottom tested loops in C.
Do While loop is an example of Exit controlled loop.
A looping process, would include the following four Steps:
1st Step: Setting and initialization of conditional variables.
2nd Step: Execution of the statements in the loop.
3rd Step: Test for a specified value of the conditional variable for the execution of the loop.
4th Step: Incrementing or updating the conditional variable.
The test may be either to determine whether the loop has been repeated the specified no of to determine whether a particular condition has been met.
C language provides three constructs for performing loop operations. They are while statement, do while statement and the for loop in C.
While Loop in C
The simplest of all looping structures in C is the while statement loop.
A General form or syntax of while loop in c as follows:
while (test condition)
{
body of the loop;
}
The while loop is an entry controlled loop statement.
A Test condition is evaluated first and if the condition is true, then the body of the loop is executed. This process of repeated execution of the body continues until the test condition is satisfied. When the condition becomes false, the control is transferred out of the loop.
The body of the loop may have one or more statements.
In the while loop, if the condition is not true, then the body of the loop will not be executed, not even once.
Execution of while loop:
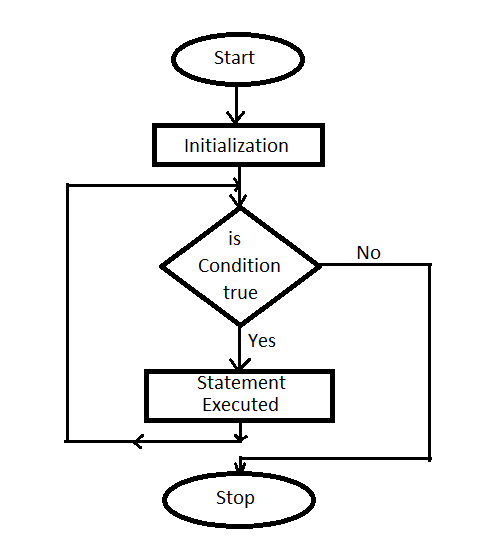
Example: Program to calculate the GCD of two positive integers.
//Learnprogramo #include <stdio.h> int main() { int n1, n2; printf("Enter two positive integers to check GCD: "); scanf("%d %d",&n1,&n2); while(n1!=n2) { if(n1 > n2) n1 -= n2; else n2 -= n1; } printf("GCD = %d",n1); return 0; }
Output:

2. Do While Loop in C
Sometimes, however, it is desirable to have a loop with the test for continuation at the end of the pass.
The do-while construct provides an exit controlled loop. This can be accomplished by means of the while statement.
A General form or syntax of a do-while loop is given below:
do
{
Body of the loop;
}
while (test condition);
Here, firstly the statements inside the loop body are executed and then the condition is evaluated. If the condition is true, then again the loop body is executed and this process continues s until the condition becomes false.
Unlike while loop, here a semicolon is placed after the condition.
In a ‘while’ loop, first, the condition is evaluated and
then the Statements are executed whereas in do-while
loop, first, the statements are executed and then the
Condition is evaluated.
So if initially, the condition is false, the while loop will not execute at all, whereas the do-while loop will always execute at least once.
Execution of do-while loop in c:
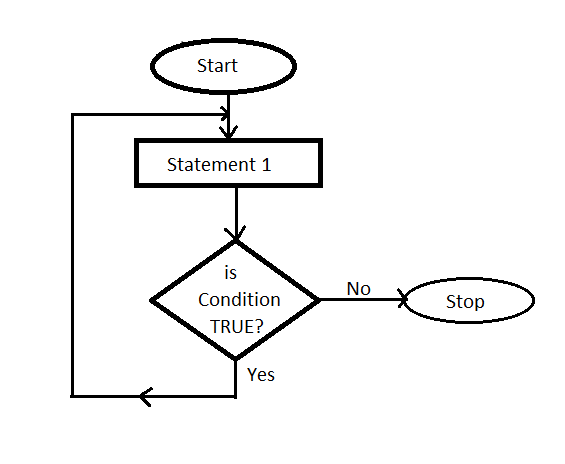
Example:
//Learnprogramo #include <stdio.h> int main() { int j=0; do { printf("Value of variable j is: %d\n", j); j++; } while (j<=3); return 0; }
Output:
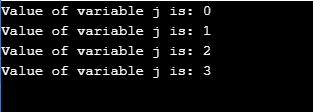
For Loop in C
The for loop in c is another entry controlled loop that provides a more concise loop control structure i.e. In single statement, loop initialization, condition testing and increment or decrement of the loop are done.
The general form or syntax of the for loop is as follows:
for( initialization; test-condition; increment/decrement)
{
body of the loop;
}
The execution of the for statement is as follows:
1. Initialization:
Initialization of the control variable is done first, using an assignment statement such as i = 1 or count = 0. The variable i and count are known as loop-control variables. If we want to initialize more than one variable then we must use a comma as an operator.
2. Test Condition:
The value of the control variable is tested using the test-condition such as relational expression. If the condition is true, the body of the loop is executed. Otherwise, the loop is terminated and the execution continues with the statement
that immediately follows the loops in C.
3. Increment or Decrement:
When the body of the loop is executed, the control is transferred back to the for statement after evaluating the last statement in the loop.
Now, the control variable is incremented or decremented and the new value Of the control variable is again tested to see whether it satisfies the loop condition.
If the condition is satisfied, the body of the loop is again
executed. This process continues until the value of the control variable fails to satisfy the test condition.
Execution of For Loops in C:
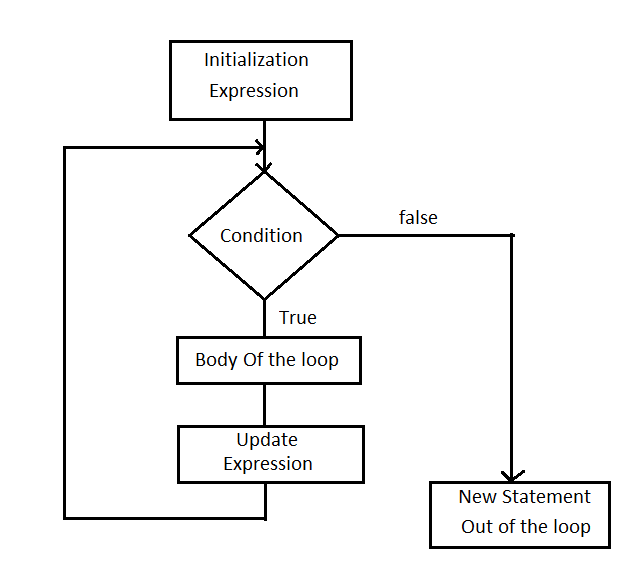
Example: Program to calculate factorial of a given number.
//Learnprogramo #include <stdio.h> int main() { int n, i; unsigned long long fact = 1; printf("Enter an integer: "); scanf("%d", &n); if (n < 0) printf("Error! Factorial of a negative number doesn't exist."); else { for (i = 1; i <= n; ++i) { fact *= i; } printf("Factorial of %d = %llu", n, fact); } return 0; }
Output:

Break Statement in C
The break statement is used inside loops and switch statements.
Sometimes it becomes necessary to come out of, the loop even before the loop condition becomes false. In such a situation, the break statement is used to terminate the loop. This statement causes an immediate exit from that loop in which this statement appears.
It can be written as or its syntax is as follows:
break;
When a break statement is encountered, the loop is terminated and the control is transferred to the statement immediately after the loop. The break statement is
generally written along with a condition.
If the break is written inside the nested loop structure then it causes an exit from the innermost loop.
Example:
//Learnprogramo #include<stdio.h> #include<stdlib.h> void main () { int i; for(i = 0; i<10; i++) { printf("%d ",i); if(i == 5) break; } printf("came outside of loop i = %d",i); }
Output:

Continue Statement in C
The continue statement is used when we want to go to the next iteration of the loop after skipping some statements of the loop. It is used to bypass the rest of the statements in a loop.
The continue statement can be written simply as or its syntax is as follows:
continue;
It is generally used with a condition. When continue statement is encountered all the remaining statements after continue in the current iteration are not executed and the loop continues with the next iteration.
The difference between break and continue is that when the break is encountered the loop terminates and the control is transferred to the next statement following the loop.
But when a continue statement is encountered the loop is not terminated and the control is transferred to the beginning of the loop.
In while and do-while loops, after continue statement the control is transferred to the test condition and then the loop continues, whereas in for loop after continue statement the control is transferred to update expression and then the condition is tested.
The continue statement is not used in a switch statement.
Example:
//Learnprogramo #include <stdio.h> int main() { for (int j=0; j<=8; j++) { if (j==4) { continue; } printf("%d ", j); } return 0; }
Output:

Also Read: