A switch case in C is used to choose a statement (for a group of the statement) among several alternatives.
It is a control statement that allows us to choose among the many given choices. The expression in switch evaluates to return an integral value, which is then compared to the values present in different cases.
It executes that block of code which matches the case value. If there is no match, then the default block is executed.
Syntax:
switch(exp)
{
case constant-1: statements1;
case constant-2: statements2;
…..
…..
default:statement n;
}
Where, constant1, constant2…… are either integer constants or character constants.
When the switch statement is executed the exp is evaluated and control is transferred directly to the group of statement whose case label value matches the value of the exp.
Statements can be simple or compound statement. But compound statement without { } is allowed in the switch statement.
If none of the case label values matches the value of the expression, then the default part statements will be executed.
If none of the case labels matches to the value of the expression and the default group is not present, then no action will be taken by the switch statement and control will be transferred out of the switch statement.
The following flowchart shows the working of the switch statement:
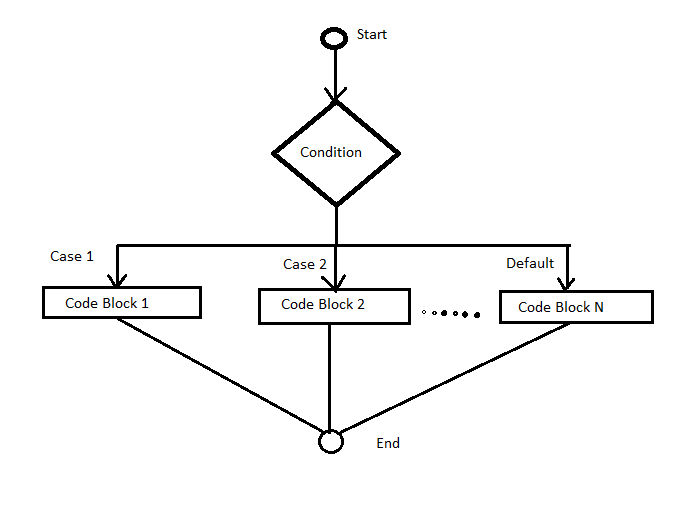
Table of Contents
Rules for using the switch case in c:
The expression (after switch keyword) must yield an integer value i., it can be an integer or character constant.
The case label i.e., values must be unique.
A case label must end with a colon(;).
We don’t use those expressions to evaluate the switch case. which may return floating-point values or strings.
Break statements are used to exit the switch block. It is necessary to use a break after each block, but if you do not use it, then all the consecutive block of codes will get executed after the matching block. But this statement is optional.
Example:
//Learnprogramo #include<stdio.h> int main() { int i=1; switch(i) { case 1: printf("A"); case 2: printf("B"); case 3: printf("C"); break; } }
Output:

The Output was supposed to be only A because only the first case matches, but as there is no break statement after that block, the next blocks are executed, until it encounters a break or reaches the end of the switch block.
The default case is executed when none of the mentioned cases matches the switch expression. Default case can be placed anywhere in the switch case. Even if we don’t include the default case, switch statement works.
A default case label can be used at most one.
Nesting of switch statements is allowed, which means you can have switch statements inside another switch. However, nested switch statements should be avoided as it makes the program more complex and less readable.
In a practical sense, the switch statement may be thought of as an alternative to the use of the nested if-else statement, though it can only replace those if-else statements that test for equality. In such situations, the use of the switch statement is generally much more convenient.
Below is the example of a simple calculator program in C using switch case:
//Learnprogramo #include<stdio.h> int main() { int choice; long num1, num2, x; printf("Please choose your option:" "\n1 = Addition" "\n2 = Subtraction" "\n3 = Multiplication" "\n4 = Division" "\n5 = Squares" "\n6 = exit" "\n\nChoice: "); scanf("%d", &choice); //while loop check whether the choice is in the given range while(choice < 1 || choice > 6) { printf("\nPlease choose the above mentioned option." "\nChoice: "); scanf("%d", &choice); } switch (choice) { case 1: printf("Enter two numbers: \n"); scanf("%ld %ld", &num1, &num2); x = num1 + num2; printf("Sum = %ld", x); break; case 2: printf("Enter two numbers: \n"); scanf("%ld %ld", &num1, &num2); x = num1 - num2; printf("Subtraction = %ld", x); break; case 3: printf("Enter two numbers: \n"); scanf("%ld %ld", &num1, &num2); x = num1 * num2; printf("Product = %ld", x); break; case 4: printf("Enter Dividend: "); scanf("%d", &num1); printf("Enter Divisor: "); scanf("%d", &num2); //while loop checks for divisor whether it is zero or not while(num2 == 0) { printf("\nDivisor cannot be zero." "\nEnter divisor once again: "); scanf("%d", &num2); } x = num1 / num2; printf("\nQuotient = %ld", x); break; case 5: printf("Enter any number: \n"); scanf("%ld", &num1); x = num1 * num1; printf("Square = %ld", x); break; case 6: return; default: printf("\nError"); } }
Output:
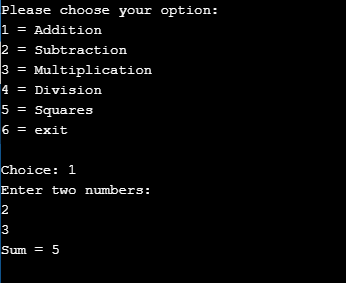
Difference between if-else and switch case in C:
if-else statement | switch statement |
---|---|
This statement will be executed depend upon the output of the test condition inside if statement. | This statement will be executed is decided by the user. |
if-else statement uses multiple statement for multiple choices. | switch statement uses single expression for multiple choices. |
if-else statement test for equality as well as for logical expression using relational and logical operators. | switch statement tests only for equality. |
if statement evaluates integer, character, pointer or floating-point type or boolean type. | switch statement evaluates only character or integer value. |
Either if statement will be executed or else statement is executed. | switch statement execute one case after another till a break statement is appeared or the end of switch statement is reached. |
Difference between else-if Ladder and Switch:
else-if Ladder | Switch Statement |
---|---|
In else-if ladder, the control goes through the every else if statement until it finds true value of the statement or it comes to the end of the else if ladder. | In case of switch case, as per the value of the switch, the control jumps to the corresponding case. |
else–if ladder is not compact and unreadable. | The switch case is more compact than lot of nested else if. So, switch is considered to be more readable. |
There is no need of use of break in else-if ladder. | The use of break statement in switch is essential. |
The else-if ladder accepts integer, float type as well as character. | The variable data type that can be used in expression of switch is integer or character constant only. |
else-if ladder works on the basis of true or false. It uses all relational operators. | Switch case statement work on the basis of equality operator. |
Break Statement:
The break statement is used inside loops and switch statements.
Sometimes it becomes necessary to come out of, the loop even before the loop condition becomes false. In such a situation, the break statement is used to terminate the loop. This statement causes an immediate exit from that loop in which this statement appears.
It can be written as or its syntax is as follows:
break;
When a break statement is encountered, the loop is terminated and the control is transferred to the statement immediately after the loop. The break statement is
generally written along with a condition.
If the break is written inside the nested loop structure then it causes an exit from the innermost loop.
Conclusion:
In this lesson, we have learned the algorithm for switch case in C with the help of proper flowchart in the next lesson we will learn for, while, do-while loops.
Also Read: