In this tutorial, we’ll code the Vaccine Management System Project in C++ in a very easy and understandable way. The Vaccine Management System can be coded using many programming languages, But in this project, we are going to use C++ language as per the demand of students.
Table of Contents
What is Vaccine Management System?
As the name suggests, the vaccine management system is software that handles all the data related to vaccination. The data contains the name of the persons who have taken the vaccine and also tells the current status of vaccine availability. Previously the task of handling the vaccination data was very difficult, so there was a need for software that can handle all the vaccination data.
Therefore the Vaccine Management System was designed. After the release of this system, the stress and workload of employees were absolutely finished. It was also time-wasting for the employees to handle the whole vaccination data with the help of a notebook. But now it hardly takes 5 to 10 minutes to search the vaccination status of a particular person.
Features of Vaccine Management System Project in C++
- Only Admin is able to login into the software.
- Admin has to enter the username, password and the captcha before accessing the data.
- The Project use the concept of file handling to store the vaccination data.
- Admin is able to create a new record of vaccination.
- All the vaccination status can be viewed at a time.
- There is a separate feature of search where admin can search a particular person very quickly.
- The entire rights are given to the admin for adding, deleting and modifying the data.
Modules of Vaccine Management System
- Add New Vaccine Record
- View Vaccine Data
- Search
- View All Data
Working of Project
In the zip file, you’ll get an executable .exe file where you can directly run the entire project manually. Now we’ll see the working of the entire application with an explanation.
Main Screen
When you run the project from any compiler or directly click on the executable .exe file you’ll see the following welcome screen shown in the picture.
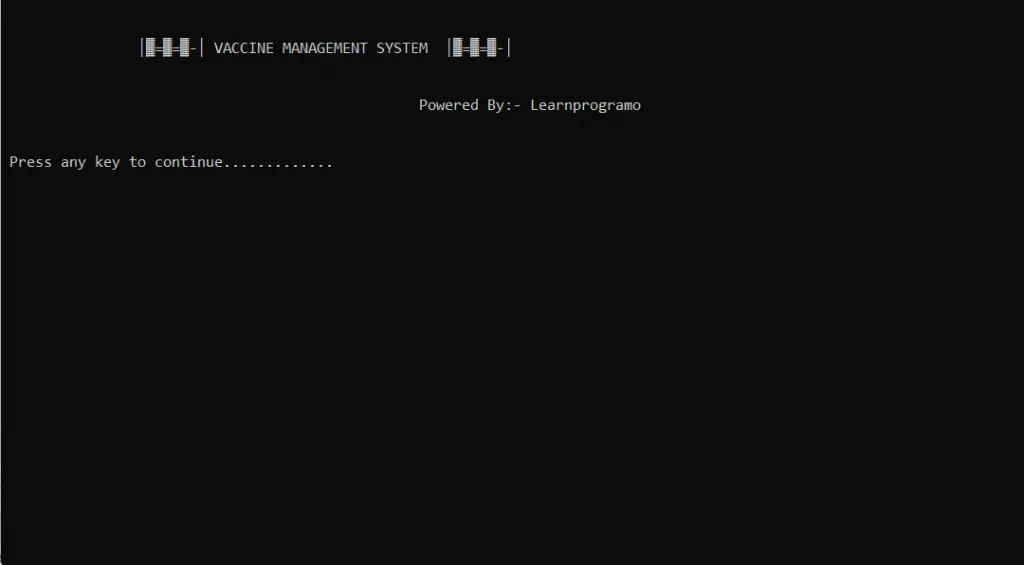
The software will ask you to press any key from the keyboard to move further, so press any key from the keyboard. After pressing any key from the keyboard you will jump to the next login page.
Login Page
After successfully pressing any key from the keyboard the following login page will appear.
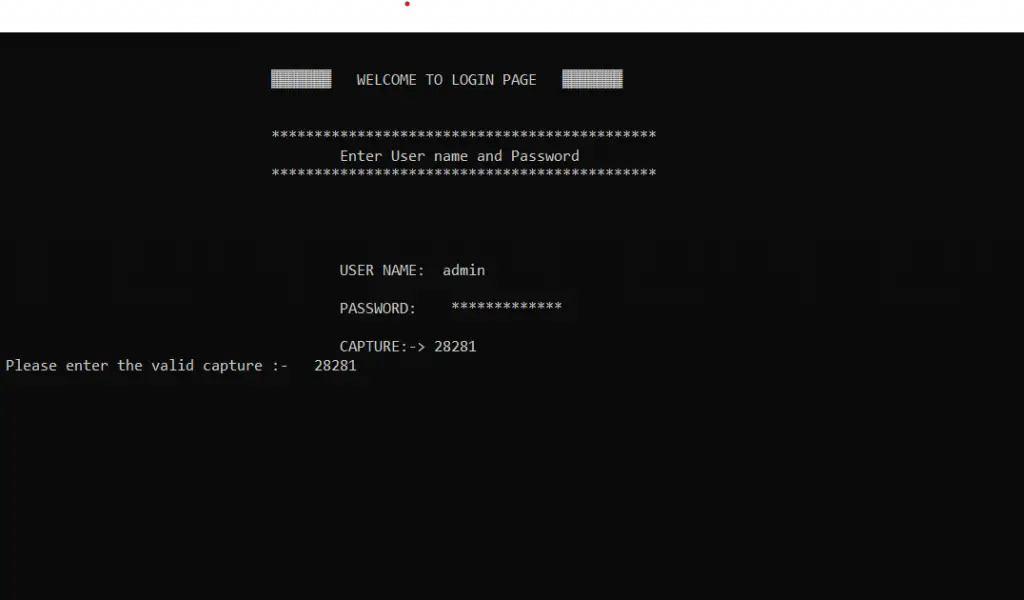
On the Login page, you have to enter the login credentials before accessing the data. The Admin has to first enter the username as “admin” then password as “learnprogramo” and then enter the captcha i.e numbers displayed on the screen. If the username, password, and captcha match then only you will be successfully logged in to the dashboard. We have also added some security to the application.
If the user other than admin tries to log in then, after 2 bad attempts the software will be blocked for some time.
Dashboard
After the successful login of Admin, the following dashboard screen will appear.
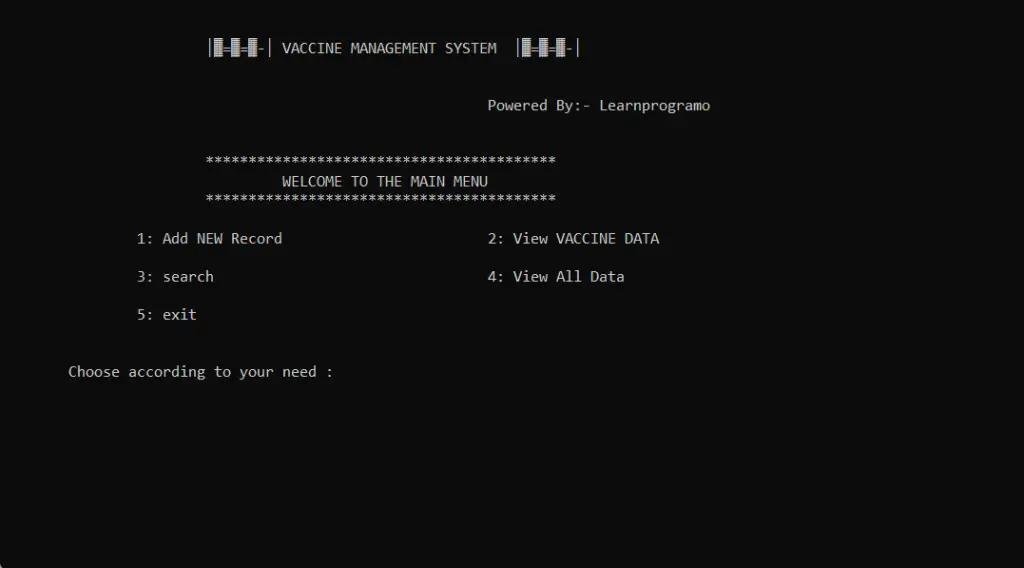
We’ve displayed the menu of Add new record, Display vaccine data, Search person, View all data. If you want to add a vaccination record the simply press 1, Similarly 2 for View Vaccine data, 3 for searching a record, and if the admin wants to see all vaccination data then simply press 4.
Now we’ll see each and every menu item in detail.
Add New Record
In this section, the admin can add a number of records of persons.
While adding a new record the software will ask for the following information of person:
- Name of the person.
- Citizenship No / Aadhar No
- Person’s Gender
- Age, Profession
- Blood Pressure, Body Temperature
- Your Address
- Mobile Number
- Name of the Vaccine you’ve Taken.
After entering the above information the new record will be successfully added to the database. This project is using the concept of file handling so the data will be stored in a separate file called “vaccinetrail“.
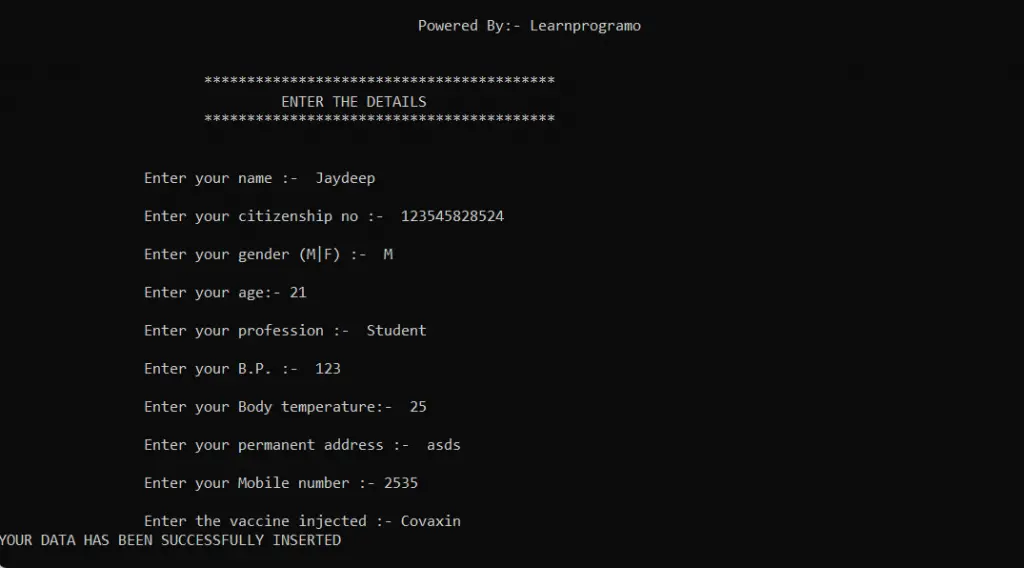
View Vaccine Data
After pressing 2 in the dashboard you’ll be jumping to the following screen.
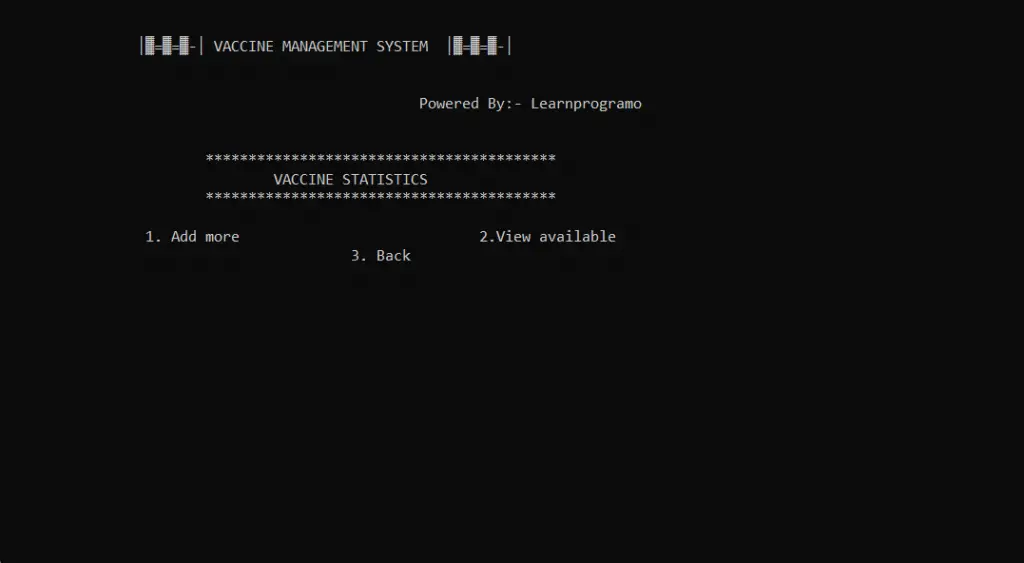
In this section, you’ll be adding or viewing the no of vaccine doses that are remained. If you want to add new vaccine doses then press 1 and enter the no of vaccine doses you want to add and then press enter. Now the doses are successfully added.
And if you want to see the no of available vaccine doses then press 2. after pressing the 2 you’ll be able to see the no of available vaccine doses. If you want to go back then press 3.
Search
In this section, the admin can be able to search for a specific person according to Citizenship No, Age, Profession, Gender, and Vaccine.
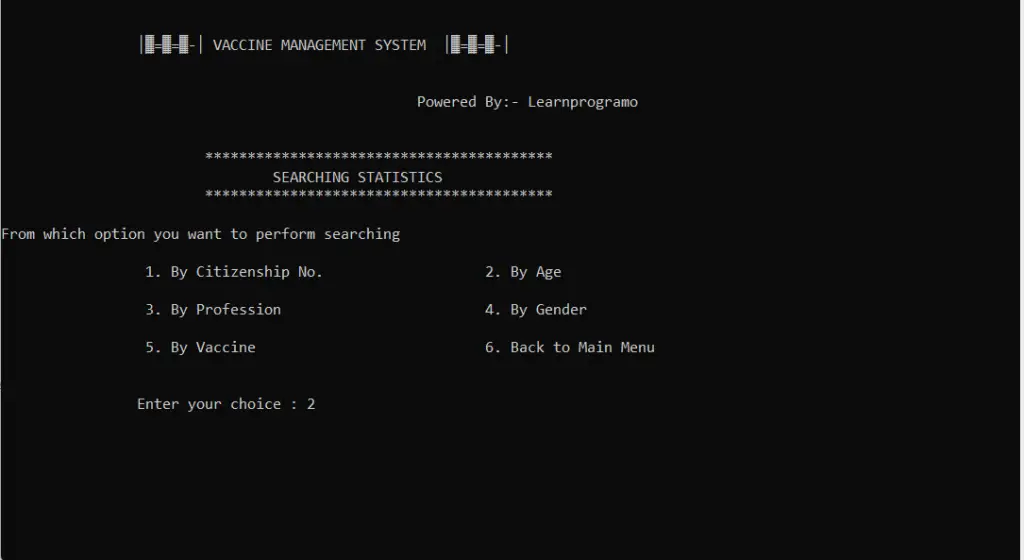
Suppose the admin wants to search persons who have taken covaxin dose then choose Search by Vaccine. After entering the covaxin name then the list of people who have taken the covaxin dose will appear.
View All Data
This is the last section of the Vaccine Management System Project in C++. In this section, the admin will be able to see all the data of records that were added by the admin.
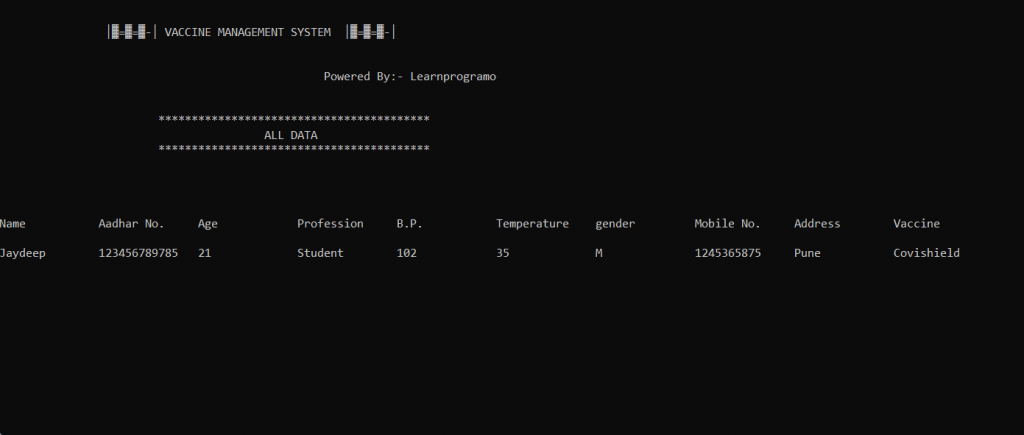
All the data will appear in this section. The data shown in this tab is read from the file “vaccinetrail“.
Exit
By choosing the 5th option vaccine management system project in c++ will be closed.
Download the Project
By clicking the following button you can download the zip file of the project which consists of the source code and the executable .exe file.
If you need quick project code then please read the following code:
//Learnprogramo //---------------------------Vaccine Management System project by using c++ programming language-------------------------- //---------------------------------------header file start----------------------------------------- #include<iostream> #include<stdio.h>//standard input output #include<fstream> #include<conio.h>//console input output #include<string.h> #include<windows.h> //for sleep #include<iomanip>//manipulate the output of C++ #include<time.h>//manipulte date and time info #define num_of_vaccine 200 using namespace std; int password();//b void menu();//c void show();//a class Vaccine { int age; int temperature; int bloodPressure; char gender; char name[100]; char citizenship[100]; char profession[100]; char address[100]; char mobileNumber[100]; char vaccine[100]; public: void setData();//1 void addNew();//2 void showData();//3 void showList();//4 void searchData();//6 void viewVaccine();//5 void search_by_citizenship();//6.1 void search_by_age();//6.2 void search_by_profession();//6.3 void search_by_gender();//6.4 void search_by_vaccine();//6.5 void view_all();//6.6 }; //---------------------------------------- void Vaccine::setData()//1 { cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t ENTER THE DETAILS "; cout<<"\n\t\t\t*****************************************\n\n"; fflush(stdin); cout<<"\n\t\t Enter your name :- "; gets(name); cout<<"\n\t\t Enter your citizenship no :- "; gets(citizenship); cout<<"\n\t\t Enter your gender (M|F) :- "; cin>>gender; cout<<"\n\t\t Enter your age:- "; cin>>age; fflush(stdin); cout<<"\n\t\t Enter your profession :- "; gets(profession); cout<<"\n\t\t Enter your B.P. :- "; cin>>bloodPressure; cout<<"\n\t\t Enter your Body temperature:- "; cin>>temperature; fflush(stdin); cout<<"\n\t\t Enter your permanent address :- "; gets(address); cout<<"\n\t\t Enter your Mobile number :- "; gets(mobileNumber); cout<<"\n\t\t Enter the vaccine injected :- "; gets(vaccine); } //------------------------------------------------------ void Vaccine::addNew()//2 { fstream outin; outin.open("vaccinetrial.txt",ios::app|ios::out); setData(); outin.write((char*)this,sizeof(Vaccine)); cout<<"YOUR DATA HAS BEEN SUCCESSFULLY INSERTED "<<endl; getch(); outin.close(); } //--------------------------------------------- void Vaccine::showData()//3 { cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:-Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t DETAILS "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"\t\t Name is: "<<name<<endl; cout<<"\t\t Citizenship number is: "<<citizenship<<endl; cout<<"\t\t Your age is "<<age<<endl; cout<<"\t\t Profession is : "<<profession<<endl; cout<<"\t\t Gender is : "<<gender<<endl; cout<<"\t\t Blood pressure is :"<<bloodPressure<<endl; cout<<"\t\t Body temperature is : "<<temperature<<endl; cout<<"\t\t Address is "<<address<<endl; cout<<"\t\t Mobile number is: "<<mobileNumber<<endl; cout<<"\n\t\t vaccine injected : "<<vaccine<<endl; } //------------------------------------------ void Vaccine::showList()//4 { cout<<"\n"; cout<<setw(15)<<setiosflags(ios::left)<<name; cout<<setw(15)<<citizenship; cout<<setw(15)<<age; cout<<setw(15)<<profession; cout<<setw(15)<<bloodPressure; cout<<setw(15)<<temperature; cout<<setw(15)<<gender; cout<<setw(15)<<mobileNumber; cout<<setw(15)<<address; cout<<setw(15)<<vaccine<<endl; } //----------------------------------------- void Vaccine ::viewVaccine()//5 { ifstream ind; int i=0; ind.open("vaccinetrial.txt"); ind.seekg(0,ios::beg); while(ind.read((char*)this,sizeof(Vaccine))) { i++; } ind.close(); int ch; int s=num_of_vaccine-i; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\tVACCINE STATISTICS"; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"\t\t 1. Add more \t\t\t2.View available \n\t\t\t\t\t 3. Back"<<endl; cin>>ch; int f_var=0; fstream file("count.txt",ios::in); file>>f_var; file.close(); switch(ch) { case 1: int m; cout<<"\t Enter number of vaccines you want to add :"<<endl; cin>>m; f_var=f_var+m; file.open("count.txt",ios::out); file.seekg(0); file<<f_var; cout<<"\t\t Now total number of vaccines are : "<<f_var+s; file.close(); break; case 2: file.open("count.txt",ios::in); cout<<"\n\nAvailable number of vaccines are : "<<s+f_var; file.close(); break; case 3: system("cls"); menu(); default: system("cls"); cout<<"\nEnter valid option "<<endl; menu(); } file.close(); getch(); } //----------------------------------------- void Vaccine::searchData()//6 { Vaccine item; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo"<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\tSEARCHING STATISTICS"; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"From which option you want to perform searching "<<endl; cout<<"\n\t\t 1. By Citizenship No. \t\t\t 2. By Age "<<endl; cout<<"\n\t\t 3. By Profession \t\t\t 4. By Gender "<<endl; cout<<"\n\t\t 5. By Vaccine \t\t\t\t 6. Back to Main Menu "<<endl; cout<<"\n\n\t\tEnter your choice : "; int ch; cin>>ch; switch(ch) { case 1: system("cls"); search_by_citizenship(); getch(); break; case 2: system("cls"); search_by_age(); getch(); break; case 3: system("cls"); search_by_profession(); getch(); break; case 4: system("cls"); search_by_gender(); getch(); break; case 5: system("cls"); search_by_vaccine(); getch(); break; case 6: system("cls"); menu(); break; default: system("cls"); cout<<"ENTER VALID OPTION"<<endl; menu(); } } //------------------------------------------------ void Vaccine::search_by_citizenship()//6.1 { ifstream in; in.open("vaccinetrial.txt"); int flag,p =0; char cs[100]; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t Citizenship SEARCHING "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"Enter your Citizenship number "<<endl; fflush(stdin); gets(cs); show(); while(!in.eof()) { if(in.read(reinterpret_cast<char*>(this),sizeof(*this))){ if(strcmp(cs,citizenship)==0) { showList(); flag=1; p++; } } } if(flag=0) { cout<<"Citizenship you entered doesnot exist"; } cout<<"\n\n\n\nNO of people vaccinated by this age"<<p; in.close(); } //--------------------------------------------------- void Vaccine::search_by_age()//6.2 { ifstream in; in.open("vaccinetrial.txt"); int flag =0; int a,p=0; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t AGE SEARCHING "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"Enter Age by which you want to search "<<endl; cin>>a; show(); while(!in.eof()) { if(in.read(reinterpret_cast<char*>(this),sizeof(*this))){ if(a==this->age) { showList(); flag=1; p++; } } } if(flag==0) { cout<<"Age you entered is not found"<<endl; } cout<<"\n\n\n\nNO of people vaccinated by this age"<<p; in.close(); } //---------------------------------------------------------------------------------- void Vaccine::search_by_profession()//6.3 { ifstream in; in.open("vaccinetrial.txt"); int flag =0; int p=0; char pf[100]; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t PROFESSION SEARCHING "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"Enter Profession by which you want to search:"<<endl; fflush(stdin); gets(pf); show(); while(!in.eof()) { if(in.read(reinterpret_cast<char*>(this),sizeof(*this))>0){ if(strcmp(pf,profession)==0) { showList(); flag=1; p++; return; } } } if(flag==0) { cout<<"SORRY!! No people by this Profession is vaccinated."<<endl; } cout<<"\n\n\n No of people vaccinated by this profession: "<<p<<endl; in.close(); } //------------------------------------------------------------------- void Vaccine::search_by_gender()//6.4 { ifstream in("vaccinetrial.txt"); int flag =0; char g; int p=0; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t GENDER SEARCHING "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"Enter gender by which you want to search "<<endl; cin>>g; show(); while(!in.eof()) { if(in.read(reinterpret_cast<char*>(this),sizeof(*this))){ if(g==this->gender) { showList(); flag=1; p++; } } } if(flag==0) { cout<<"SORRY!! No people by this Gender is vaccinated."<<endl; } cout<<"\n\n\n NO. of people vaccinated by this gender: "<<p<<endl; in.close(); } //------------------------------------------------------------------------- void Vaccine::search_by_vaccine()//6.5 { ifstream in; in.open("vaccinetrial.txt"); int flag =0; int p=0; char pf[100]; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t PROFESSION SEARCHING "; cout<<"\n\t\t\t*****************************************\n\n"; cout<<"Enter Vaccine to search: "; fflush(stdin); gets(pf); show(); while(!in.eof()) { if(in.read(reinterpret_cast<char*>(this),sizeof(*this))>0){ if(strcmp(pf,vaccine)==0) { showList(); flag=1; p++; return; } } } if(flag==0) { cout<<"SORRY!! No people by this vaccine is vaccinated."<<endl; } cout<<"\n\n\n No of people vaccinated by this vaccine: "<<p<<endl; in.close(); } //--------------------------------------------------------- void show()//a { cout<<"\n\n\n"; cout<<setw(15)<<setiosflags(ios::left)<<"Name "; cout<<setw(15)<<"Aadhar No."; cout<<setw(15)<<"Age"; cout<<setw(15)<<"Profession"; cout<<setw(15)<<"B.P."; cout<<setw(15)<<"Temperature"; cout<<setw(15)<<"gender"; cout<<setw(15)<<"Mobile No."; cout<<setw(15)<<"Address"; cout<<setw(15)<<"Vaccine"<<endl; } //------------------------------------------------- void Vaccine::view_all(){ fstream outfile; outfile.open("vaccinetrial.txt",ios::binary|ios::in); if(!outfile){ cout<<"File doesnot exist"; } cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t\tALL DATA "; cout<<"\n\t\t\t*****************************************\n\n"; show(); while(!outfile.eof()){ if(outfile.read(reinterpret_cast<char*>(this),sizeof(*this))>0){ showList(); } } getch(); outfile.close(); } //-------------------------------------------------------------------- int password()//b { char cname[30],pass[20]; int ch, i=0,cap=0,capt=0; cout<<"\n\n\n\n\t\t\t\t\tUSER NAME: "; fflush(stdin); gets(cname); cout<<"\n\t\t\t\t\tPASSWORD: "; while((ch=getch()) != 13) { cout<<"*"; pass[i]=ch; i++; } pass[i] = '\0'; srand(time(0)); cap=rand(); cout<<"\n\n\t\t\t\t\tCAPTURE:-> "<<cap<<endl; cout<<" Please enter the valid capture :- "; cin>>capt; if( (strcmp(cname,"admin")==0) && (strcmp(pass,"learnprogramo")==0) && cap==capt) return 1; else return 0; } //---------------------------------------- void menu()//c { system("cls"); cout<<"\n\n\t\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl<<endl; cout<<"\n\t\t\t*****************************************\n"; cout<<"\t\t\t\t WELCOME TO THE MAIN MENU"; cout<<"\n\t\t\t*****************************************\n"; cout<<"\n\t\t1: Add NEW Record\t\t\t 2: View VACCINE DATA"<<endl; cout<<"\n\t\t3: search \t\t\t\t 4: View All Data"<<endl; cout<<"\n\t\t5: exit"<<endl; } //---------------------------------------- int main()//MAINFUNCTION--------------------------------------------------------------------------------------------- { Vaccine obj; int k=3; int num_vac; cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo "<<endl; cout<<"\t\n\n Press any key to continue............. "<<endl; getch(); B: system("cls"); cout<<" \n\n\t\t\t\t\xB2\xB2\xB2\xB2\xB2\xB2\xB2 WELCOME TO LOGIN PAGE \xB2\xB2\xB2\xB2\xB2\xB2\xB2"<<endl; cout<<"\n\n\t\t\t\t*********************************************"; cout<<"\n\t\t\t\t\tEnter User name and Password\n"; cout<<"\t\t\t\t*********************************************\n"; while(k>=1) { int c = password(); if(c==1) break; else cout<<"\n\n\t\t Wrong Password Or User Name \n\n\t\t You Can try Only "<<k-1<<" times more"; k--; if(k<1) { for(int i=59; i>=0; i--) { system("cls"); cout<<"\n\n\n\n\n\n\n\n\t\t\t\t\tYOU ARE BLOCKED FOR 1 MINUTE!!"; cout<<"\n\n\n\n\n\n\t\t\t\t\tTRY AFTER "<<i<<" SECONDS....."; Sleep(1000); } k=3; goto B; } } int ch; do{ cout<<"\n\n\t\t\xB3\xB2=\xB2=\xB2-\xB3 VACCINE MANAGEMENT SYSTEM \xB3\xB2=\xB2=\xB2-\xB3\n\n"<<endl; cout<<"\t\t\t\t\t\t Powered By:- Learnprogramo"<<endl; menu(); cout<<"\n\t\n\tChoose according to your need : "; cin>>ch; Vaccine v1; switch(ch) { case 1: system("cls"); obj.addNew(); break; case 2: system("cls"); obj.viewVaccine(); break; case 3:system("cls"); obj.searchData(); break; case 4:system("cls"); obj.view_all(); break; case 5: system("cls"); exit(0); default: system("cls"); cout<<"\n\n\n\n\n\n\n\n\t\t\t\t\t\tTHANK YOU!!"; cout<<"\n\n\t\t\t\t\t****HAVE A NICE DAY*****"; Sleep(3000); exit(0); } }while(ch!=0); return 0; } //----------------------------------------------------------------------------------------------------------------------
Steps to Run the Project
- Extract the Downloaded Zip file or copy the above source code.
- Open the file using C++ Compiler like Dev C++ etc.
- Paste the source code and save the file in any of the computer locations.
- Compile the project using compile and run option.
- There is not error in the source code, if found then correct it.
- Now run the project
- The executable .exe file will be generated in the location you choosed.
Summary
In this way, we’ve created and executed the Vaccine Management System Project in C++. If you have any doubt then please feel free to contact us. We’ll solve your doubts as soon as possible.
Thanks and HAPPY CODING 🙂
Also Read:
- 50+ Interesting C Projects.
- 25+ C++ Projects.
- Library Management System Project in C++.
- Railway Mangement System Project in C++.