In this tutorial, we’ll code the Library Management System Project in C++ in a very easy and understandable way. The Library Management System can be coded using many programming languages, But in this project, we are going to use C++ language as per the demand of students.
Table of Contents
What is Library Management System?
As the name suggest the Library Management System is a software which handles the entire data of library. It makes the work of librarian very easy instead of writing data in a notebook. In past the librarians were using notebooks to write the data of books along with students name who borrowed that book. So it was very difficult to keep track on each and every book.
If librarian want’s to search for a particular book then that task was very time consuming. So to make this task easy the programming languages were developed and C++ language is one of them.
Features of Library Management System Project in C++
- We have created separate logins for students and the librarian, in which the librarian is password protected.
- In this project, the librarian can add, update, delete and create books and can also assign the books to the students.
- The students can also view the list of the books available in the entire library database.
- The entire rights are given to the librarian to adding books, issuing books, and modify the book.
- This project uses file handling to store the data of books in a project.
- A Librarian can also be able to change the password.
- Reissuing and returning the books are the main features of this project.
- The student can also be able to see which student has already borrowed the book.
Modules of Library Management System
- Add Book.
- Modify Book.
- Delete Book.
- Search Book.
- Issue Book.
- Return Book.
Working of Project
In the zip file you’ll get executable .exe file where you can directly run the entire project manually. Now we’ll see the working of entire application with explanation.
Main Screen:
When you run the project from any compiler or directly clicking on the executable .exe file you’ll see the following screen shown in the picture.
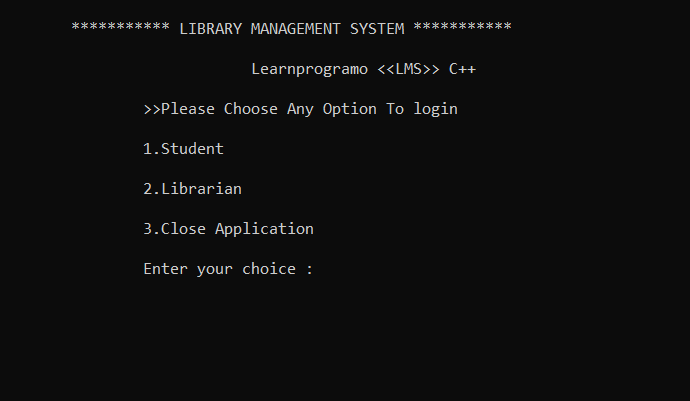
We have displayed the menu of Student, Librarian and close the application. If you’re a student then your choice will be 1 and if you are a librarian then your choice will be 2.
Now we’ll discuss each and every menu in details.
1. Student
The student will not require additional sign in, he or she will be able to access the software directly.
When the student enter the choice as 1 then following screen will be appeared:
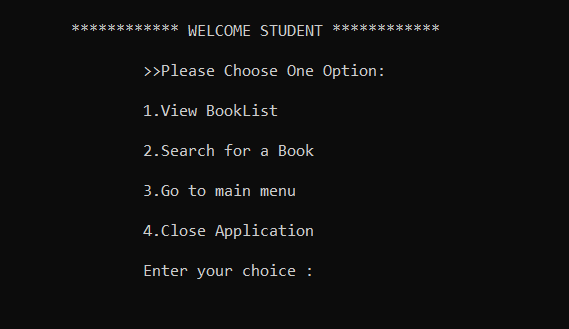
1. View Booklist:
In this menu option all the students according to their branches will be able to view the books present in the database along with their details.
2. Search For a Book:
We have given access to the students to search for a particular book. The student can search book either by book name or by book id. Both the options are available in the project.
3. Go to Main Menu:
When the user has done the required operations and if he want to again move to the main menu, then pressing 3 as choice he’ll moved to the main menu.
4. Close Application:
By pressing the choice as 4 the application will be closed.
2. Librarian:
To access the features of the librarian menu, He will require to sign in using the password which is “learnprogramo“. We’ve also given the facility to change the password in the Librarian menu. Only Librarian has rights to change the password.
When the user press the choice as 2. then the software will ask you to enter the correct password as shown in the following image:
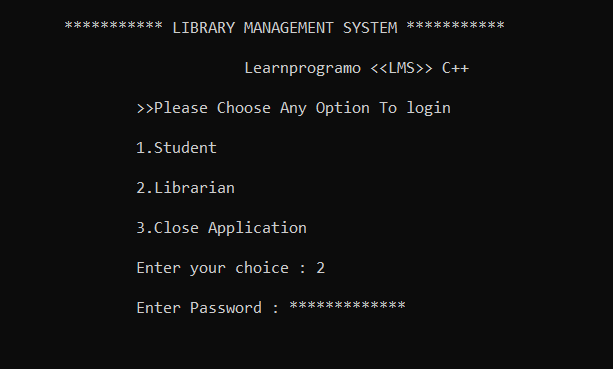
If the password is incorrect the application will show the error of wrong password. And if the password is correct then the librarian menu will be visible to the user where he or she can do the operations displayed in the menu.
The following menu will be visible to the Librarian:
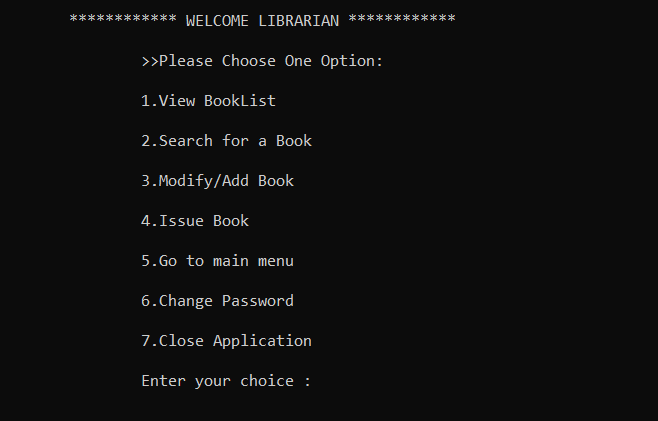
1. View Booklist:
Same as students view booklist, librarians will also able to see the books available in the library database.
2. Search For a Book:
The Librarian can search book either by book name or by book id. Both the options are available in the project.
3. Modify/Add Book:
In this menu option Librarian can do three main operations i.e. Adding a Book, Deleting a Book and Modifying the existing Book.
As we are using the file handling methods in this project, Every time new file is generated to store the details of the books. i.e. Booksdata.txt.
4. Issue Book:
Due to this option The 70% of the work is been reduced. In this option Librarian can do the following operations:
- Issue a Book.
- View Issued Books.
- He can also search the students who issued the books.
- Librarian can also reissue the book to the same student.
- Return the Book.
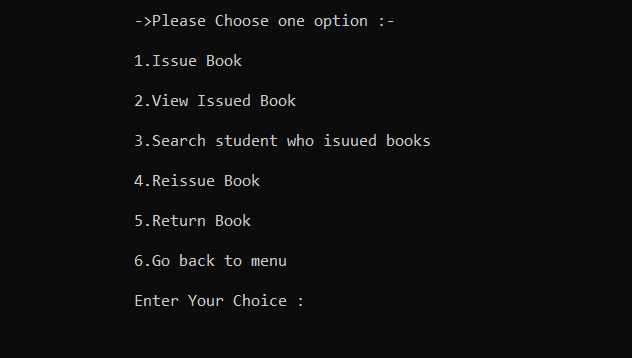
To store the student details the separate file name Student.txt is been created.
Download the Project
By click the following button you can download the zip file of the project which consist of source code and the executable .exe file.
If you need quick project code then please read the following code:
Source Code of Library Management System Project in C++
//Learnprogramo #include<iostream> #include<stdio.h> #include<stdlib.h> #include<fstream> #include<string.h> #include<conio.h> using namespace std; class Lib { public: char bookname[100],auname[50],sc[20],sc1[50]; int q,B,p; Lib() { strcpy(bookname,"NO Book Name"); strcpy(auname,"No Author Name"); strcpy(sc,"No Book ID"); strcpy(sc1,"No Book ID"); q=0; B=0; p=0; } void get(); void student(); void pass(); void librarian(); void password(); void getdata(); void show(int); void booklist(int); void modify(); void see(int); int branch(int); void issue(); void der(char[],int,int); void fine(int,int,int,int,int,int); }; void Lib::getdata() { int i; fflush(stdin); cout<<"\n\t\tEnter the details :-\n"; cout<<"\n\t\tEnter Book's Name : "; cin.getline(bookname,100); for(i=0;bookname[i]!='\0';i++) { if(bookname[i]>='a'&&bookname[i]<='z') bookname[i]-=32; } cout<<"\n\t\tEnter Author's Name : "; cin.getline(auname,50); cout<<"\n\t\tEnter Publication name : "; cin.getline(sc1,50); cout<<"\n\t\tEnter Book's ID : "; cin.getline(sc,20); cout<<"\n\t\tEnter Book's Price : "; cin>>p; cout<<"\n\t\tEnter Book's Quantity : "; cin>>q; } void Lib::show(int i) { cout<<"\n\t\tBook Name : "<<bookname<<endl; cout<<"\n\t\tBook's Author Name : "<<auname<<endl; cout<<"\n\t\tBook's ID : "<<sc<<endl; cout<<"\n\t\tBook's Publication : "<<sc1<<endl; if(i==2) { cout<<"\n\t\tBook's Price : "<<p<<endl; cout<<"\n\t\tBook's Quantity : "<<q<<endl; } } void Lib::booklist(int i) { int b,r=0; system("cls"); b=branch(i); system("cls"); ifstream intf("Booksdata.txt",ios::binary); if(!intf) cout<<"\n\t\tFile Not Found."; else { cout<<"\n\t ************ Book List ************ \n\n"; intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { if(b==B) { if(q==0 && i==1) { } else { r++; cout<<"\n\t\t********** "<<r<<". ********** \n"; show(i); } } intf.read((char*)this,sizeof(*this)); } } cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); if(i==1) student(); else librarian(); } void Lib::modify() { char ch,st1[100]; int i=0,b,cont=0; system("cls"); cout<<"\n\t\t>>Please Choose one option :-\n"; cout<<"\n\t\t1.Modification In Current Books\n\n\t\t2.Add New Book\n\n\t\t3.Delete A Book\n\n\t\t4.Go back\n"; cout<<"\n\n\t\tEnter your choice : "; cin>>i; if(i==1) { system("cls"); b=branch(2); ifstream intf1("Booksdata.txt",ios::binary); if(!intf1) { cout<<"\n\t\tFile Not Found\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); librarian(); } intf1.close(); system("cls"); cout<<"\n\t\tPlease Choose One Option :-\n"; cout<<"\n\t\t1.Search By Book Name\n\n\t\t2.Search By Book's ID\n"; cout<<"\n\t\tEnter Your Choice : "; cin>>i; fflush(stdin); if(i==1) { system("cls"); cout<<"\n\t\tEnter Book Name : "; cin.getline(st1,100); system("cls"); fstream intf("Booksdata.txt",ios::in|ios::out|ios::ate|ios::binary); intf.seekg(0); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;b==B&&bookname[i]!='\0'&&st1[i]!='\0'&&(st1[i]==bookname[i]||st1[i]==bookname[i]+32);i++); if(bookname[i]=='\0'&&st1[i]=='\0') { cont++; getdata(); intf.seekp(intf.tellp()-sizeof(*this)); intf.write((char*)this,sizeof(*this)); break; } intf.read((char*)this,sizeof(*this)); } intf.close(); } else if(i==2) { cout<<"\n\t\tEnter Book's ID : "; cin.getline(st1,100); system("cls"); fstream intf("Booksdata.txt",ios::in|ios::out|ios::ate|ios::binary); intf.seekg(0); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;b==B&&sc[i]!='\0'&&st1[i]!='\0'&&st1[i]==sc[i];i++); if(sc[i]=='\0'&&st1[i]=='\0') { cont++; getdata(); intf.seekp(intf.tellp()-sizeof(*this)); intf.write((char*)this,sizeof(*this)); break; } intf.read((char*)this,sizeof(*this)); } intf.close(); } else { cout<<"\n\t\tIncorrect Input.....:(\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); modify(); } if(cont==0) { cout<<"\n\t\tBook Not Found.\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); modify(); } else cout<<"\n\t\tUpdate Successful.\n"; } else if(i==2) { system("cls"); B=branch(2); system("cls"); getdata(); ofstream outf("Booksdata.txt",ios::app|ios::binary); outf.write((char*)this,sizeof(*this)); outf.close(); cout<<"\n\t\tBook added Successfully.\n"; } else if(i==3) { system("cls"); b=branch(2); ifstream intf1("Booksdata.txt",ios::binary); if(!intf1) { cout<<"\n\t\tFile Not Found\n"; cout<<"\n\t\tPress any key to continue....."; getch(); intf1.close(); system("cls"); librarian(); } intf1.close(); system("cls"); cout<<"\n\t\tPlease Choose One Option for deletion:-\n"; cout<<"\n\t\t1.By Book Name\n\n\t\t2.By Book's ID\n"; cout<<"\n\t\tEnter Your Choice : "; cin>>i; fflush(stdin); if(i==1) { system("cls"); cout<<"\n\t\tEnter Book Name : "; cin.getline(st1,100); ofstream outf("temp.txt",ios::app|ios::binary); ifstream intf("Booksdata.txt",ios::binary); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;b==B&&bookname[i]!='\0'&&st1[i]!='\0'&&(st1[i]==bookname[i]||st1[i]==bookname[i]+32);i++); if(bookname[i]=='\0'&&st1[i]=='\0') { cont++; intf.read((char*)this,sizeof(*this)); } else { outf.write((char*)this,sizeof(*this)); intf.read((char*)this,sizeof(*this)); } } intf.close(); outf.close(); remove("Booksdata.txt"); rename("temp.txt","Booksdata.txt"); } else if(i==2) { cout<<"\n\t\tEnter Book's ID : "; cin.getline(st1,100); ofstream outf("temp.txt",ios::app|ios::binary); ifstream intf("Booksdata.txt",ios::binary); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;b==B&&sc[i]!='\0'&&st1[i]!='\0'&&st1[i]==sc[i];i++); if(sc[i]=='\0'&&st1[i]=='\0') { cont++; intf.read((char*)this,sizeof(*this)); } else { outf.write((char*)this,sizeof(*this)); intf.read((char*)this,sizeof(*this)); } } outf.close(); intf.close(); remove("Booksdata.txt"); rename("temp.txt","Booksdata.txt"); } else { cout<<"\n\t\tIncorrect Input.....:(\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); modify(); } if(cont==0) { cout<<"\n\t\tBook Not Found.\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); modify(); } else cout<<"\n\t\tDeletion Successful.\n"; } else if(i==4) { system("cls"); librarian(); } else { cout<<"\n\t\tWrong Input.\n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); modify(); } cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); librarian(); } int Lib::branch(int x) { int i; cout<<"\n\t\t>>Please Choose one Branch :-\n"; cout<<"\n\t\t1.Class 12th\n\n\t\t2.CS\n\n\t\t3.EC\n\n\t\t4.CIVIL\n\n\t\t5.MECHANICAL\n\n\t\t6.1ST YEAR\n\n\t\t7.Go to menu\n"; cout<<"\n\t\tEnter youur choice : "; cin>>i; switch(i) { case 1: return 1; break; case 2: return 2; break; case 3: return 3; break; case 4: return 4; break; case 5: return 5; break; case 6: return 6; break; case 7: system("cls"); if(x==1) student(); else librarian(); default : cout<<"\n\t\tPlease enter correct option :("; getch(); system("cls"); branch(x); } } void Lib::see(int x) { int i,b,cont=0; char ch[100]; system("cls"); b=branch(x); ifstream intf("Booksdata.txt",ios::binary); if(!intf) { cout<<"\n\t\tFile Not Found.\n"; cout<<"\n\t\t->Press any key to continue....."; getch(); system("cls"); if(x==1) student(); else librarian(); } system("cls"); cout<<"\n\t\tPlease Choose one option :-\n"; cout<<"\n\t\t1.Search By Name\n\n\t\t2.Search By Book's ID\n"; cout<<"\n\t\tEnter Your Choice : "; cin>>i; fflush(stdin); intf.read((char*)this,sizeof(*this)); if(i==1) { cout<<"\n\t\tEnter Book's Name : "; cin.getline(ch,100); system("cls"); while(!intf.eof()) { for(i=0;b==B&&q!=0&&bookname[i]!='\0'&&ch[i]!='\0'&&(ch[i]==bookname[i]||ch[i]==bookname[i]+32);i++); if(bookname[i]=='\0'&&ch[i]=='\0') { cout<<"\n\t\tBook Found :-\n"; show(x); cont++; break; } intf.read((char*)this,sizeof(*this)); } } else if(i==2) { cout<<"\n\t\tEnter Book's ID : "; cin.getline(ch,100); system("cls"); while(!intf.eof()) { for(i=0;b==B&&q!=0&&sc[i]!='\0'&&ch[i]!='\0'&&ch[i]==sc[i];i++); if(sc[i]=='\0'&&ch[i]=='\0') { cout<<"\n\t\tBook Found :-\n"; show(x); cont++; break; } intf.read((char*)this,sizeof(*this)); } } else { cont++; cout<<"\n\t\tPlease enter correct option :("; getch(); system("cls"); see(x); } intf.close(); if(cont==0) cout<<"\n\t\tThis Book is not available :( \n"; cout<<"\n\t\tPress any key to continue....."; getch(); system("cls"); if(x==1) student(); else librarian(); } void Lib::issue() { char st[50],st1[20]; int b,i,j,d,m,y,dd,mm,yy,cont=0; system("cls"); cout<<"\n\t\t->Please Choose one option :-\n"; cout<<"\n\t\t1.Issue Book\n\n\t\t2.View Issued Book\n\n\t\t3.Search student who isuued books\n\n\t\t4.Reissue Book\n\n\t\t5.Return Book\n\n\t\t6.Go back to menu\n\n\t\tEnter Your Choice : "; cin>>i; fflush(stdin); if(i==1) { system("cls"); b=branch(2); system("cls"); fflush(stdin); cout<<"\n\t\t->Please Enter Details :-\n"; cout<<"\n\t\tEnter Book Name : "; cin.getline(bookname,100); cout<<"\n\t\tEnter Book's ID : "; cin.getline(sc,20); //strcpy(st,sc); der(sc,b,1); cout<<"\n\t\tEnter Student Name : "; cin.getline(auname,100); cout<<"\n\t\tEnter Student's ID : "; cin.getline(sc1,20); cout<<"\n\t\tEnter date : "; cin>>q>>B>>p; ofstream outf("student.txt",ios::binary|ios::app); outf.write((char*)this,sizeof(*this)); outf.close(); cout<<"\n\n\t\tIssue Successfully.\n"; } else if(i==2) { ifstream intf("student.txt",ios::binary); system("cls"); cout<<"\n\t\t->The Details are :-\n"; intf.read((char*)this,sizeof(*this)); i=0; while(!intf.eof()) { i++; cout<<"\n\t\t********** "<<i<<". ********** \n"; cout<<"\n\t\tStudent Name : "<<auname<<"\n\t\t"<<"Student's ID : "<<sc1<<"\n\t\t"<<"Book Name : "<<bookname<<"\n\t\t"<<"Book's ID : "<<sc<<"\n\t\t"<<"Date : "<<q<<"/"<<B<<"/"<<p<<"\n"; intf.read((char*)this,sizeof(*this)); } intf.close(); } else if(i==3) { system("cls"); fflush(stdin); cout<<"\n\t\t->Please Enter Details :-\n"; cout<<"\n\n\t\tEnter Student Name : "; cin.getline(st,50); cout<<"\n\n\t\tEnter Student's ID : "; cin.getline(st1,20); system("cls"); ifstream intf("student.txt",ios::binary); intf.read((char*)this,sizeof(*this)); cont=0; while(!intf.eof()) { for(i=0;sc1[i]!='\0'&&st1[i]!='\0'&&st1[i]==sc1[i];i++); if(sc1[i]=='\0'&&st1[i]=='\0') { cont++; if(cont==1) { cout<<"\n\t\t->The Details are :-\n"; cout<<"\n\t\tStudent Name : "<<auname; cout<<"\n\t\tStudent's ID : "<<sc1; } cout<<"\n\n\t\t******* "<<cont<<". Book details *******\n"; cout<<"\n\t\tBook Name : "<<bookname; cout<<"\n\t\tBook's ID : "<<sc; cout<<"\n\t\tDate : "<<q<<"/"<<B<<"/"<<p<<"\n"; } intf.read((char*)this,sizeof(*this)); } intf.close(); if(cont==0) cout<<"\n\t\tNo record found."; } else if(i==4) { system("cls"); fflush(stdin); cout<<"\n\t\t->Please Enter Details :-\n"; cout<<"\n\n\t\tEnter Student's ID : "; cin.getline(st,50); cout<<"\n\t\tEnter Book's ID : "; cin.getline(st1,20); fstream intf("student.txt",ios::in|ios::out|ios::ate|ios::binary); intf.seekg(0); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;sc[i]!='\0'&&st1[i]!='\0'&&st1[i]==sc[i];i++); for(j=0;sc1[j]!='\0'&&st[j]!='\0'&&st[j]==sc1[j];j++); if(sc[i]=='\0'&&sc1[j]=='\0'&&st[j]=='\0'&&st1[i]=='\0') { d=q; m=B; y=p; cout<<"\n\t\tEnter New Date : "; cin>>q>>B>>p; fine(d,m,y,q,B,p); //fn1 intf.seekp(intf.tellp()-sizeof(*this)); //fn3 intf.write((char*)this,sizeof(*this)); //fn5 cout<<"\n\n\t\tReissue successfully."; //fn3 break; } intf.read((char*)this,sizeof(*this)); } intf.close(); } else if(i==5) { system("cls"); b=branch(2); system("cls"); fflush(stdin); cout<<"\n\t\t->Please Enter Details :-\n"; cout<<"\n\t\tEnter Book's ID : "; cin.getline(st1,20); der(st1,b,2); cout<<"\n\n\t\tEnter Student's ID : "; cin.getline(st,20); cout<<"\n\t\tEnter Present date : "; cin>>d>>m>>y; ofstream outf("temp.txt",ios::app|ios::binary); ifstream intf("student.txt",ios::binary); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;sc[i]!='\0'&&st1[i]!='\0'&&st1[i]==sc[i];i++); for(j=0;sc1[j]!='\0'&&st[j]!='\0'&&st[j]==sc1[j];j++); if(sc[i]=='\0'&&sc1[j]=='\0'&&st[j]=='\0'&&st1[i]=='\0'&&cont==0) { cont++; intf.read((char*)this,sizeof(*this)); fine(q,B,p,d,m,y); cout<<"\n\t\tReturned successfully."; } else { outf.write((char*)this,sizeof(*this)); intf.read((char*)this,sizeof(*this)); } } intf.close(); outf.close(); getch(); remove("student.txt"); rename("temp.txt","student.txt"); } else if(i==6) { system("cls"); librarian(); } else cout<<"\n\t\tWrong Input.\n"; cout<<"\n\n\t\tPress any key to continue....."; getch(); system("cls"); librarian(); } void Lib::fine(int d,int m,int y,int dd,int mm,int yy) { long int n1,n2; int years,l,i; const int monthDays[12] = {31, 28, 31, 30, 31, 30,31, 31, 30, 31, 30, 31}; n1 = y*365 + d; for (i=0; i<m - 1; i++) n1 += monthDays[i]; //fn1353 years = y; if (m <= 2) years--; l= years / 4 - years / 100 + years / 400; n1 += l; n2 = yy*365 + dd; for (i=0; i<mm - 1; i++) n2 += monthDays[i]; years = yy; if (m <= 2) years--; l= years / 4 - years / 100 + years / 400; n2 += l; n1=n2-n1; n2=n1-15; if(n2>0) cout<<"\n\t\tThe Total Fine is : "<<n2; } void Lib::der(char st[],int b,int x) { int i,cont=0; fstream intf("Booksdata.txt",ios::in|ios::out|ios::ate|ios::binary); intf.seekg(0); intf.read((char*)this,sizeof(*this)); while(!intf.eof()) { for(i=0;b==B&&sc[i]!='\0'&&st[i]!='\0'&&st[i]==sc[i];i++); if(sc[i]=='\0'&&st[i]=='\0') { cont++; if(x==1) { q--; } else { q++; } intf.seekp(intf.tellp()-sizeof(*this)); intf.write((char*)this,sizeof(*this)); break; } intf.read((char*)this,sizeof(*this)); } if(cont==0) { cout<<"\n\t\tBook not found.\n"; cout<<"\n\n\t\tPress any key to continue....."; getch(); system("cls"); issue(); } intf.close(); } void Lib::get() { int i; cout<<"\n\t*********** LIBRARY MANAGEMENT SYSTEM ***********\n"<<"\n\t\t\t Learnprogramo <<LMS>> C++\n"; cout<<"\n\t\t>>Please Choose Any Option To login \n"; cout<<"\n\t\t1.Student\n\n\t\t2.Librarian\n\n\t\t3.Close Application\n"; cout<<"\n\t\tEnter your choice : "; cin>>i; if(i==1) { system("cls"); student(); } else if(i==2) pass(); else if(i==3) exit(0); else { cout<<"\n\t\tPlease enter correct option :("; getch(); system("CLS"); get(); } } void Lib::student() { int i; cout<<"\n\t************ WELCOME STUDENT ************\n"; cout<<"\n\t\t>>Please Choose One Option:\n"; cout<<"\n\t\t1.View BookList\n\n\t\t2.Search for a Book\n\n\t\t3.Go to main menu\n\n\t\t4.Close Application\n"; cout<<"\n\t\tEnter your choice : "; cin>>i; if(i==1) booklist(1); else if(i==2) see(1); else if(i==3) { system("cls"); get(); } else if(i==4) exit(0); else { cout<<"\n\t\tPlease enter correct option :("; getch(); system("cls"); student(); } } void Lib::pass() { int i=0; char ch,st[21],ch1[21]={"learnprogramo"}; cout<<"\n\t\tEnter Password : "; while(1) { ch=getch(); if(ch==13) { st[i]='\0'; break; } else if(ch==8&&i>0) { i--; cout<<"\b \b"; } else { cout<<"*"; st[i]=ch; i++; } } ifstream inf("password.txt"); inf>>ch1; inf.close(); for(i=0;st[i]==ch1[i]&&st[i]!='\0'&&ch1[i]!='\0';i++); if(st[i]=='\0'&&ch1[i]=='\0') { system("cls"); librarian(); } else { cout<<"\n\n\t\tWrong Password.\n\n\t\ttry again.....\n"; getch(); system("cls"); get(); } } void Lib::librarian() { int i; cout<<"\n\t************ WELCOME LIBRARIAN ************\n"; cout<<"\n\t\t>>Please Choose One Option:\n"; cout<<"\n\t\t1.View BookList\n\n\t\t2.Search for a Book\n\n\t\t3.Modify/Add Book\n\n\t\t4.Issue Book\n\n\t\t5.Go to main menu\n\n\t\t6.Change Password\n\n\t\t7.Close Application\n"; cout<<"\n\t\tEnter your choice : "; cin>>i; switch(i) { case 1:booklist(2); break; case 2:see(2); break; case 3:modify(); break; case 4:issue(); break; case 5:system("cls"); get(); break; case 6:password(); break; case 7:exit(0); default:cout<<"\n\t\tPlease enter correct option :("; getch(); system("cls"); librarian(); } } void Lib::password() { int i=0,j=0; char ch,st[21],ch1[21]={"learnprogramo"}; system("cls"); cout<<"\n\n\t\tEnter Old Password : "; while(1) { ch=getch(); if(ch==13) { st[i]='\0'; break; } else if(ch==8&&i>0) { i--; cout<<"\b \b"; } else { cout<<"*"; st[i]=ch; i++; } } ifstream intf("password.txt"); intf>>ch1; intf.close(); for(i=0;st[i]==ch1[i]&&st[i]!='\0'&&ch1[i]!='\0';i++); if(st[i]=='\0'&&ch1[i]=='\0') { system("cls"); cout<<"\n\t**The Password Should be less than 20 characters & don't use spaces**\n\n"; cout<<"\n\t\tEnter New Password : "; fflush(stdin); i=0; while(1) { j++; ch=getch(); if(ch==13) { for(i=0;st[i]!=' '&&st[i]!='\0';i++); if(j>20 || st[i]==' ') { cout<<"\n\n\t\tYou did't follow the instruction \n\n\t\tPress any key for try again....."; getch(); system("cls"); password(); librarian(); } st[i]='\0'; break; } else if(ch==8&&i>0) { i--; cout<<"\b \b"; } else { cout<<"*"; st[i]=ch; i++; } } ofstream outf("password.txt"); outf<<st; outf.close(); cout<<"\n\n\t\tYour Password has been changed Successfully."; cout<<"\n\t\tPress any key to continue......"; getch(); system("cls"); librarian(); } else { cout<<"\n\n\t\tPassword is incorrect.....\n"; cout<<"\n\t\tEnter 1 for retry or 2 for menu"; cin>>i; if(i==1) { system("cls"); password(); } else { system("cls"); librarian(); } } } int main() { Lib obj; obj.get(); getch(); return 0; }
Steps to Run the Project
- Extract the Downloaded Zip file or copy the above source code.
- Open the file using C++ Compiler such as Dev C++ etc.
- paste the source code and save the file in any of the computer locations.
- Compile the project using compile option.
- There is no error in the source code, if found then correct it.
- Now run the project.
- The executable .exe file will be generated in the location you choose.
Summary:
In this way, we’ve created and executed the Library Management System Project in C++. If you have any doubt then please feel free to contact us. We’ll solve your doubts as soon as possible.
Thanks and HAPPY CODING 🙂
Also Read: