In this tutorial, we’ll code the Railway Reservation System Project in C++ in a very easy and understandable way. The Railway Reservation System can be coded using many programming languages, But in this project, we are going to use C++ language as per the demand of students.
Table of Contents
What is Railway Reservation System?
As the name suggests Railway Reservation System is software that handles the entire booking data of the Railway. It is fully based on the concept of reserving train tickets for various destinations. Previously the task of handling the tickets at a time was very difficult, so there was a need for software that can handle all Railway Reservation System.
Therefore the Railway Reservation System was designed. After the release of this system, the stress and workload of the employee were absolutely finished. It was also time-wasting for the travelers to book a Ticket previously. But now it hardly takes 10 to 15 minutes to book a ticket wherever the passenger is.
Features of Railway Reservation System Project in C++
- We have created separate logins for the passengers as well as admin, in which the admin login is password protected.
- In this project, the admin can add, update, delete and create the trains.
- The passenger can book the train only if the train is added by the admin.
- Passengers are able to see the actual data of available trains so that they can choose which they want.
- The entire rights are given to the admin adding, modifying and deleting the train.
- This project use concept of file handling to store the booking data.
- Cancelling the ticket is an main feature of this project.
- Admin is able to change the password of login.
Modules of Railway Reservation System
- Add Train
- Modify Train
- Delete Train
- Search Train
- Book Train
- Cancel Booking
Working of Project
In the zip file, you’ll get an executable .exe file where you can directly run the entire project manually. Now we’ll see the working of the entire application with an explanation.
Main Screen:
When you run the project from any compiler or directly clicking on the executable .exe file you’ll see the following screen shown in the picture.
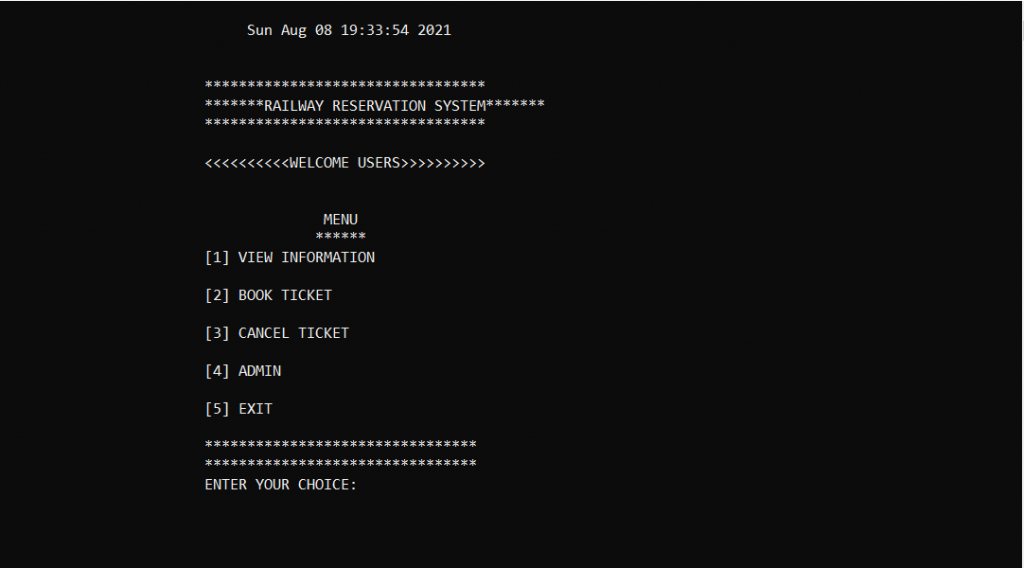
We’ve displayed the menu of View information, Book the ticket, Cancel ticket, Admin, and Exit. If you want to see the details of all available trains then your choice will be 1. Similarly 2 for booking the ticket, 3 for cancellation of ticket. If you are an admin you should give choice as 4.
Now we’ll see each and every menu in detail.
View Information:
In this option, the user will be able to see all the details of the available trains. The details of all the trains will be displayed here which are stored in the file name “train_details”. Only the admin can add the train details, no one other than the admin can do operations on train details.
The passengers did not require additional sign-in to book the train. He or she’ll be able to book the train directly.
When the user chooses the option as 1 then the following screen will appear:
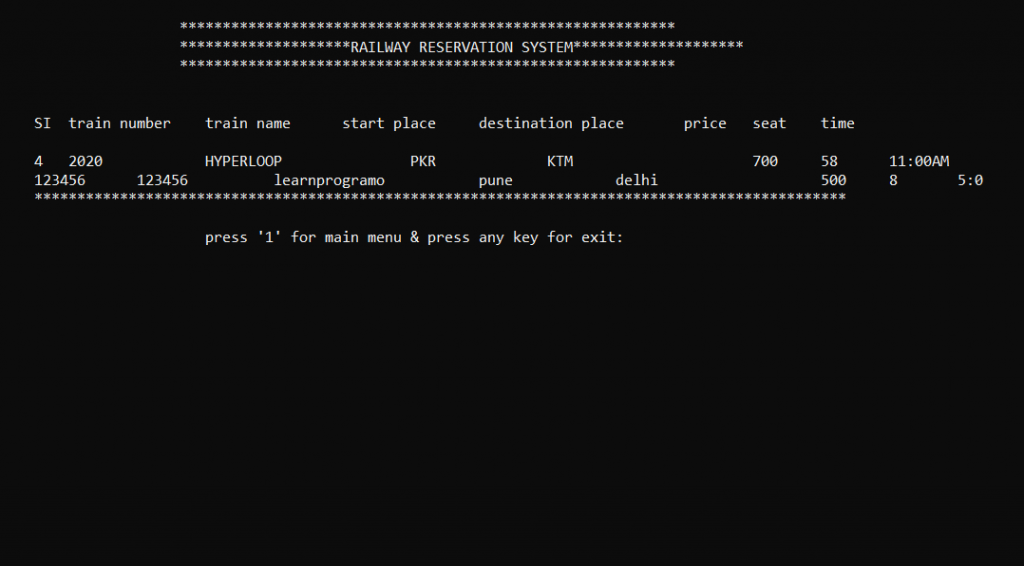
The above screen contains all the details of trains. If you want to return to the main menu then press 1 or press any key to exit the application.
Book Ticket:
In this section, passengers will be able to book the tickets. When the Passenger chooses option 2 then the following screen will appear.
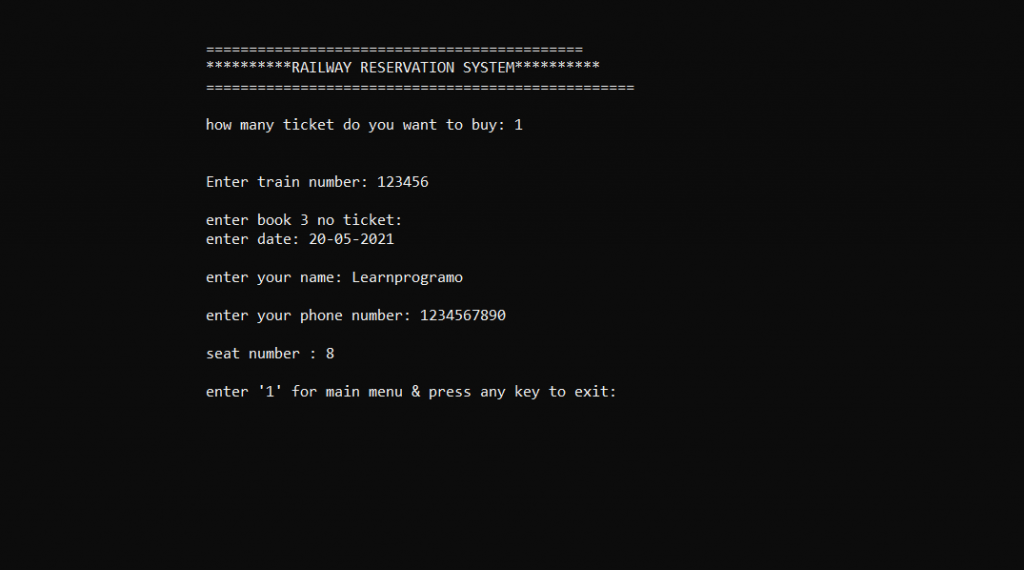
After entering the choice as 2, the software will ask the passenger to enter the following information:
- No of tickets which passenger wants to book.
- Train number.
- Date on which passenger want to travel.
- Name of the passengers.
- Contact Number of each passenger.
The software will ask for the above details for each and every passenger. Suppose passengers enter no of tickets as 2 then the software’ll ask for the above details of two passengers. After entering the details, the software will automatically allocate the seat number to the passenger.
Cancel Ticket:
If the passenger’s mind changed and he wants to cancel the ticket urgently then the software also has the facility of canceling the ticket on the spot. To cancel the ticket, the passenger should choose option 3.
After choosing option 3, the following screen will appear:
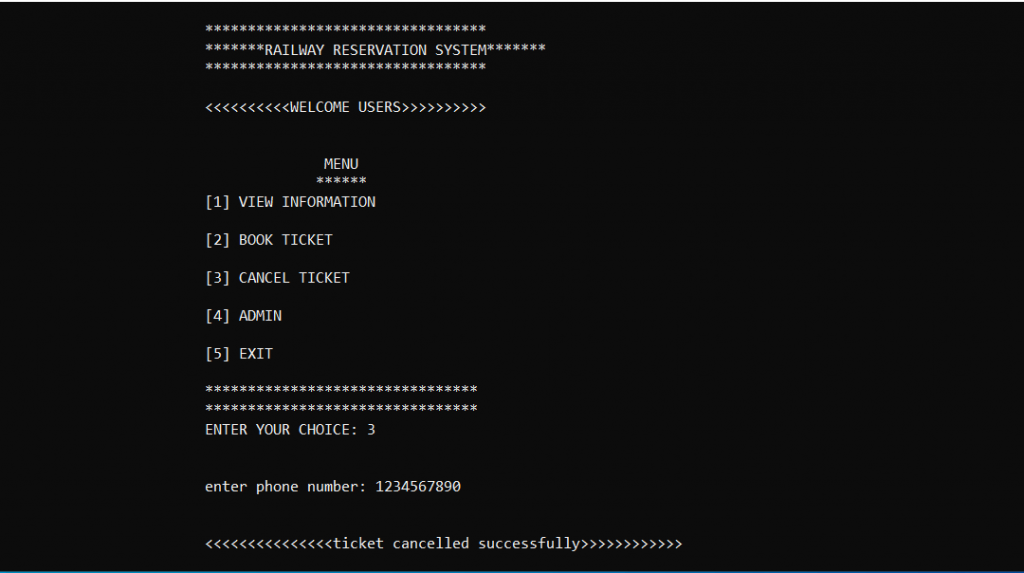
The ticket will be canceled only on the basis of the contact number. If the passenger’s contact number matches with the number which he entered while booking the ticket.
The success message will appear after the cancellation of the ticket.
Admin:
The Admin is also called the heart of the software or project because he’s the only one who can handle the entire Train Booking System. We’ve given all the read, write, update and delete permissions only to the admin. If the user is admin then he should enter the choice as 4.
After entering the choice as 4 the following admin panel will appear:
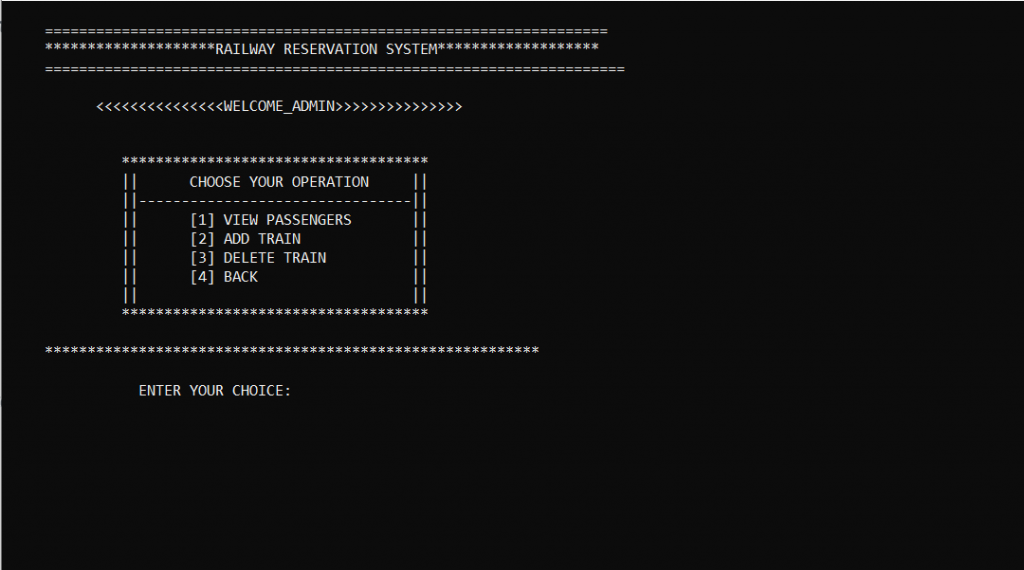
The admin panel is password-protected so, the user other than admin will not be able to access the dashboard. The password for admin login is “1234567”. After entering the correct password, the following dashboard will be visible to the admin.
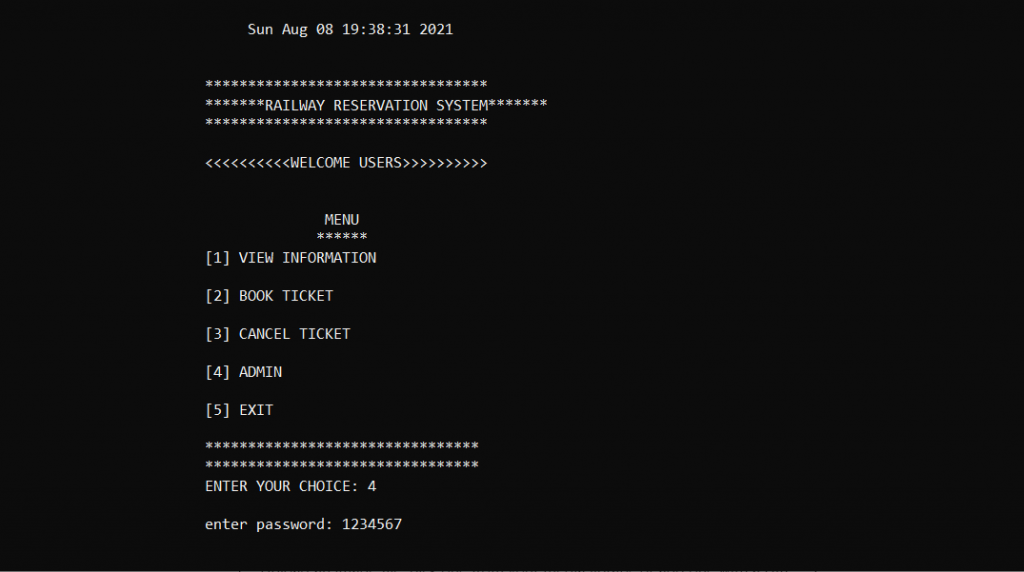
Now we’ll discuss each and every operation which is present on the admin dashboard.
1. View Passengers:
In this menu option, all the booking details of the passengers will be displayed with the train number and seat number. Press 1 if you want to access the main menu or press 0 for a back operation.
2. Add Train:
Admin will be able to add the trains which are ready to travel on a specific date. While adding the train, the software will ask each and every following detail of the train:
- Serial number.
- Train number.
- Name of the Train.
- Start Place.
- Destination Place.
- Price of the Ticket.
- Number of available Seats.
- Time of Departure.
And after entering all details press 0 for no or press 1 for confirming train.
3. Delete Train:
If the admin wants to delete the train due to some issues, then the admin will be able to instantly delete the train by choosing this option. Trian will be deleted only on the basis of train number.
4. Back:
This option will be chosen if the admin wants to access the main menu.
Exit:
By choosing the 5th option railway reservation system project in c++ will be closed.
Download the Project
By clicking the following button you can download the zip file of the project which consists of the source code and the executable .exe file.
If you need quick project code then please read the following code:
Source Code of Railway Reservation System Project in C++
//Learnprogramo //---------------------------rail way reservation project by using c programming language-------------------------- //---------------------------------------header file start----------------------------------------- #include<stdio.h> #include<windows.h> #include<stdlib.h> #include<conio.h> #include<time.h> #include<string.h> //---------------------------------------header file close------------------------------------------- //---------------------------------------user define function start---------------------------------- void viewinfo(); void bookticket(); void cancelticket(); void admin(); void password(); void viewpassenger(); void addtrain(); void dlttrain(); void awrite(); void aread(); void bookticket_write(); void viewpassengers_read(); //-----------------------------------------user define function close----------------------------------- //-----------------------------------------structure start---------------------------------------------- struct adddata { char si[10]; char train_number[10]; char train_name[20]; char start[10]; char destination[10]; char price[10]; int seat; char time[10]; } add[1000]; struct bookticket { char train_number[20]; char name[20]; char phone[20]; char date[20]; int seat; }book[1000]; //---------------------------------------structure close---------------------------------------------- //---------------------------------------global variable---------------------------------------------- int k=0,u=0; char trn_nmbr[100],name[100],phn[100]; //---------------------------------------main function start------------------------------------------ int main() { aread(); viewpassengers_read(); system("COLOR 0f"); int ch; time_t currentTime; time(¤tTime); printf("\n\t\t\t %s\n",ctime(¤tTime)); printf("\n\t\t\t*********************************\n"); printf("\t\t\t*******RAILWAY RESERVATION SYSTEM*******\n"); printf("\t\t\t*********************************\n"); printf("\n\t\t\t<<<<<<<<<<WELCOME USERS>>>>>>>>>>\n"); printf("\n\n\t\t\t\t MENU\n"); printf("\t\t\t ******"); printf("\n\t\t\t[1] VIEW INFORMATION\n"); printf("\n\t\t\t[2] BOOK TICKET\n"); printf("\n\t\t\t[3] CANCEL TICKET\n"); printf("\n\t\t\t[4] ADMIN"); printf("\n\n\t\t\t[5] EXIT\n"); printf("\n\t\t\t********************************"); printf("\n\t\t\t********************************"); printf("\n\t\t\tENTER YOUR CHOICE: "); scanf("%d",&ch); switch(ch) { case 1: viewinfo(); break; case 2: bookticket(); break; case 3: cancelticket(); break; case 4: password(); break; case 5: system("cls"); printf("\n\t\t\t =========================================\n"); printf("\t\t\t *******RAILWAY RESERVATION SYSTEM*******\n"); printf("\t\t\t ===============================================\n"); printf("\n\n\t\t\tBMROUGHT TO YOU BY\n\n"); printf("\t\t\t\t***Learnprogramo***\n"); getch(); exit(0); break; default: system("cls"); printf("\n\t\t\t==============================================\n"); printf("\t\t\t *******RAILWAY RESERVATION SYSTEM*******\n"); printf("\t\t\t ==============================================\n"); printf("\n\n\t\t\t<<<<<<<<YOU ENTERED WRONG CHOICE>>>>>>>>\n"); printf("\t\t\t<<<<<<<<PLEASE ENTER RIGHT THING>>>>>>>>\n"); getch(); system("cls"); main(); } return 0; } //---------------------------------------main function close-------------------------------------------------- //---------------------------------------book ticket function----------------------------------------------- void bookticket() { int c,j,n,i,found=-1; char v,train_number[10]; system ("cls"); aread(); printf("\n\n\t\t\t============================================"); printf("\n\t\t\t**********RAILWAY RESERVATION SYSTEM**********\n"); printf("\t\t\t=================================================="); printf("\n\n\t\t\thow many ticket do you want to buy: "); scanf("%d",&n); for(j=u;j<u+n;j++) { printf("\n\n\t\t\tEnter train number: "); scanf("%s", book[j].train_number); for(i=0;i<k;i++) { if(strcmp(book[j].train_number,add[i].train_number)==0) { if(add[i].seat==0) { printf("\n\n\t\t\tnot available seat"); getch(); system("cls"); main(); } else { found=1; printf("\n\t\t\tenter book %d no ticket: ",j+1); printf("\n\t\t\tenter date: "); scanf("%s",book[j].date); printf("\n\t\t\tenter your name: "); scanf("%s",book[j].name); printf("\n\t\t\tenter your phone number: "); scanf("%s",book[j].phone); printf("\n\t\t\tseat number : %d",add[i].seat ); book[j].seat=add[i].seat; bookticket_write(); add[i].seat--; awrite(); } } } if(found==-1) { printf("\n\n\t\t\ttrain not found!!!"); getch(); system("cls"); main(); } } u=j; bookticket_write(); printf("\n\n\t\t\tenter '1' for main menu & press any key to exit: "); scanf("%d",&c); if(c==1) { system("cls"); main(); } if(c!=1) { exit; } } //---------------------------------------cancel ticket function--------------------------------------------- void cancelticket() { viewpassengers_read(); char pnnmbr[100]; int location = -1,e; printf ("\n\n\t\t\tenter phone number: "); scanf ("%s",pnnmbr); for (e=0;e<u;e++) { if (strcmp(pnnmbr,book[e].phone)==0) { location=e; break; } } if (location==-1) { printf ("\n\n\t\t\t<<<<<<<<<<<<<<Data Not Found>>>>>>>>>>>>>>>>> \n"); getch(); system("cls"); main(); } else { for (e=location;e<u;e++) { strcpy(book[e].date,book[e+1].date); strcpy(book[e].train_number,book[e+1].train_number); strcpy(book[e].name,book[e+1].name); strcpy(book[e].phone,book[e+1].phone); bookticket_write(); } u--; bookticket_write(); printf("\n\n\t\t\t<<<<<<<<<<<<<<<ticket cancelled successfully>>>>>>>>>>>>"); getch(); system("cls"); main(); } } //-------------------------------------admin portal function---------------------------------------- void admin() { int chhh; system("cls"); printf("\n =================================================================="); printf("\n ********************RAILWAY RESERVATION SYSTEM*******************"); printf("\n ===================================================================="); printf("\n\n"); printf(" <<<<<<<<<<<<<<<WELCOME_ADMIN>>>>>>>>>>>>>>>\n"); printf("\n\n"); printf(" ************************************\n"); printf(" || CHOOSE YOUR OPERATION ||\n"); printf(" ||--------------------------------||\n"); printf(" || [1] VIEW PASSENGERS ||\n"); printf(" || [2] ADD TRAIN ||\n"); printf(" || [3] DELETE TRAIN ||\n"); printf(" || [4] BACK ||\n"); printf(" || ||\n"); printf(" ************************************\n\n"); printf(" **********************************************************\n"); printf("\n\t\tENTER YOUR CHOICE: "); scanf("%d",&chhh); switch(chhh) { case 1: viewpassenger(); break; case 2: addtrain(); break; case 3: dlttrain(); break; case 4: system("cls"); getch(); main(); break; default: getch(); printf("\n\t\t\tyou entered wrong KEY!!!!"); getch(); system("cls"); main(); } getch(); } //-----------------------------password function---------------------------------- void password() { int number=1234567; int pass; printf("\n\t\t\tenter password: "); scanf("%d",&pass); if(pass==number) { printf("\n\n\t\t\t<<<<<login successfully>>>>>"); getch(); system("cls"); admin(); } else { printf("\n\n\t\t\t\t ERROR!!!!!"); printf("\n\n\t\t\t<<<<<<<<wrong password>>>>>>>>"); getch(); system("cls"); main(); } } //------------------------------------delete train function---------------------------------------------- void dlttrain() { aread(); char train[100]; int location = -1,f; printf ("\n\n\tenter train number: "); scanf ("%s",train); for (f=0;f<k;f++) { if (strcmp(train,add[f].train_number)==0) { location=f; break; } } if (location==-1) { printf ("\n\n\t<<<<<<<<<<<<<<Data Not Found>>>>>>>>>>>>>>>>> \n"); getch(); system("cls"); admin(); } else { for (f=location;f<k;f++) { strcpy(add[f].si,add[f+1].si); strcpy(add[f].train_number,add[f+1].train_number); strcpy(add[f].train_name,add[f+1].train_name); strcpy(add[f].start,add[f+1].start); strcpy(add[f].destination,add[f+1].destination); strcpy(add[f].price,add[f+1].price); strcpy(add[f].time,add[f+1].time); awrite(); } k--; awrite(); printf("\n\n\t<<<<<<<<<<<<<train deleted successfully>>>>>>>>>>>>>"); getch(); system("cls"); admin(); } } //--------------------------------------view passengers function---------------------------------------- void viewpassenger() { int a,j; system("cls"); viewpassengers_read(); printf("\n\t\t\t **********************************************************"); printf("\n\t\t\t ********************RAILWAY RESERVATION SYSTEM********************"); printf("\n\t\t\t **********************************************************"); printf("\n\n\t\t\ttrain number\t\tname\t\tphone number\t\tdate\t\tseat\n"); printf("\n\t\t\t**********************************************************************************\n"); for(j=0;j<u;j++) { printf("\n\t\t\t%s\t\t\t%s\t\t%s\t\t%s\t%d",book[j].train_number,book[j].name,book[j].phone,book[j].date,book[j].seat); book[j].seat++; } printf("\n\t\t\t**********************************************************************************\n"); printf("\n\n\t\t\tenter '1' for main menu & enter '0' for back: "); scanf("%d",&a); if(a==1) { system("cls"); main(); } if(a==0) { system("cls"); admin(); } } //--------------------------------------add train function-------------------------------------------- void addtrain() { system("cls"); int ch; aread(); int i,a; printf("\n\t\t **********************************************************"); printf("\n\t\t ********************RAILWAY RESERVATION SYSTEM********************"); printf("\n\t\t **********************************************************"); printf("\n\n\t\t\thow many trains do you want to add: "); scanf("%d",&a); for(i=k;i<k+a;i++) { printf("\n\t\t\tenter %d train details: ",i+1); printf("\n\t\t\tenter serial number: "); scanf("%s",add[i].si); printf("\n\t\t\tenter train number: "); scanf("%s",add[i].train_number); printf("\n\t\t\tenter train name: "); scanf("%s",add[i].train_name); printf("\n\t\t\tenter start place: "); scanf("%s",add[i].start); printf("\n\t\t\tenter destination place: "); scanf("%s",add[i].destination); printf("\n\t\t\t enter price: "); scanf("%s",add[i].price); printf("\n\t\t\t enter seat: "); scanf("%d", & add[i].seat); printf("\n\t\t\t enter time: "); scanf("%s",add[i].time); } printf("\n\n\t\t\tconfirm train: (y=1/n=0):- "); scanf("%d",&ch); if(ch==1) { awrite(); k=i; awrite(); system("cls"); printf("\n\n\t\t\t**********************************************************"); printf("\n\t\t\t********************RAILWAY RESERVATION SYSTEM********************"); printf("\n\t\t\t**********************************************************"); printf("\n\n"); printf("\n\t\t\t\t **********************************"); printf("\n\t\t\t\t *<<<<<train add successfully>>>>>*"); printf("\n\t\t\t\t **********************************"); getch(); system("cls"); main(); } if(ch==0) { system("cls"); admin(); } if((ch!=1)&&(ch!=0)) { system("cls"); main(); } } //-----------------------------------view information function-------------------------------------- void viewinfo() { int ch,i; system("cls"); aread(); printf("\n\t\t **********************************************************"); printf("\n\t\t ********************RAILWAY RESERVATION SYSTEM********************"); printf("\n\t\t **********************************************************"); printf("\n\n\n SI\ttrain number\ttrain name\tstart place\tdestination place\tprice\tseat\ttime\n\n"); for(i=0;i<k;i++) { printf(" %s\t%s\t\t%s\t\t%s\t\t%s\t\t\t%s\t%d\t%s\n",add[i].si,add[i].train_number,add[i].train_name,add[i].start,add[i].destination,add[i].price,add[i].seat,add[i].time); } printf(" ***********************************************************************************************\n"); printf("\n\t\t\tpress '1' for main menu & press any key for exit: "); scanf("%d",&ch); switch(ch) { case 1: system("cls"); main(); break; default: exit(0); } } //------------------------------------------book ticket file start----------------------------------------- void bookticket_write() { FILE *booklist; booklist=fopen("booklist.txt","w"); fwrite(&book,sizeof(book),1,booklist); fclose(booklist); FILE *booklistreport; booklistreport=fopen("booklistreport.txt","w"); fwrite(&u,sizeof(u),1,booklistreport); fclose(booklistreport); } void viewpassengers_read() { FILE *booklist; booklist=fopen("booklist.txt","r"); fread(&book,sizeof(book),1,booklist); fclose(booklist); FILE *booklistreport; booklistreport=fopen("booklistreport.txt","r"); fread(&u,sizeof(u),1,booklistreport); fclose(booklistreport); } //-----------------------------------------add train file start--------------------------------------------------- void awrite() { FILE *train_details; train_details = fopen("train_details.txt","w"); fwrite(&add,sizeof(add),1,train_details); fclose(train_details); FILE *train_report; train_report = fopen("train_report.txt","w"); fwrite(&k,sizeof(k),1,train_report); fclose(train_report); } void aread() { FILE *train_details; train_details = fopen("train_details.txt","r"); fread(&add,sizeof(add),1,train_details); fclose(train_details); FILE *train_report; train_report = fopen("train_report.txt","r"); fread(&k,sizeof(k),1,train_report); fclose(train_report); } //----------------------------------------------------file close---------------------------------------------- //----------------------------------------------------program close----------------------------------------
Steps to Run the Project
- Extract the Downloaded Zip file or copy the above source code.
- Open the file using C++ Compiler such as Dev C++ etc.
- Paste the source code and save the file in any of the computer locations.
- Compile the project using compile option.
- There is no error in the source code, if found then correct it.
- Now run the project.
- The executable .exe file will be generated in the location you choose.
Summary:
In this way, we’ve created and executed the Railway Reservation System Project in C++. If you have any doubt then please feel free to contact us. We’ll solve your doubts as soon as possible.
Thanks and HAPPY CODING :)
Also Read:
- 50+ Interesting C Projects.
- 25+ C++ Projects.
- Library Management System Project in C++.
- Airline Reservation System Project in C++.