In this tutorial, we’ll learn about the prime adam number in java as well as the prime adam number program in java. So, before getting started we’ll first learn a little more about adam and prime numbers. So,
Table of Contents
What are Prime Numbers?
Any natural number which can be divisible by itself or the number which has only two factors i.e 1 and the number itself are called prime numbers.
For example 2,3,5,7,11 and so on…
Note: The number 2 is only an even prime number because most of the numbers are divisible by 2.
What is Adam Numbers?
The Adam Numbers are the type of numbers in which the square of the number and the square of its reverse are reversed to each other.
For Example: Consider a number i.e 12. The square of the 12 is 144. Now reverse the number 12 and find the square of it, i.e square of 21 is 441.
Here square of 12*12 = 144 and the square of its reverse 21*21 = 441 are reverse to each other. That’s why 12 is called Adam Number.
Some numbers other than 12 which are also adam numbers are 13, 22, 31, 101, 102, 103, etc.
What is Prime Adam Number in Java?
The number which is Prime Number, as well as Adam Number, is called Prime Adam Number in Java. The number which will satisfy the below two conditions will be called as Prime Adam Number.
- The given number should be a prime number.
- And its square and the square of its reverse should be reverse of each other.
For Example Number 13.
Here, the number 13 is prime so the first condition is satisfied.
Now, let’s check whether it’s adam number or not. The square of 13 is 13*13 = 169. Now reverse the number i.e 31. The square of 31 is 31*31 = 961. We get the square roots 13 = 169 and 31 = 961. Both the square roots are reverse of each other. So the second condition is also satisfied. Therefore, the number 13 is Prime Adam Number in Java.
Let us learn how to code the prime adam number program in java.
Prime Adam Number Program in Java
Problem Statement: Accept the two positive integers a and b, where a is less than the b as the user input. Display all the prime adam numbers that are in the range between a and b. Display the output with the frequency in the format given below:
Test your program with the following data and some random data:
1st Sample Test Case:
Input:
a = 5, b = 100
Output:
The Prime Adam Numbers are 11, 13, and 31.
The Frequency of Prime Adam Number is: 3
2nd Sample Test Case:
Input:
a = 50, b = 70
Output:
The Prime Adam Numbers are NIL.
The frequency of Prime Adam Number is: 0
3rd Sample Test Case:
Input:
a = 100, b = 200
Output:
The Prime Adam numbers are 101, 103, and 113.
The Frequency of Prime Adam Number is: 3
4th Sample Test Case:
Input:
a = 700, b = 450
Output:
Invalid Input.
Program:
//Learnprogramo - programming made simple import java.util.*; class Main { boolean isPrime(int b) { int c = 0; for(int i=1; i<=b; i++) { if(b%i == 0) { c++; } } if(c == 2) return true; else return false; } int reverseNum(int b) { int r = 0, d = 0; while(b > 0) { d = b%10; r = r*10 + d; b = b/10; } return r; } boolean isAdam(int b) { int rev = reverseNum(b); int sqn = b*b; int sqr = rev * rev; int rsqn = reverseNum(sqn); if(rsqn == sqr) return true; else return false; } public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter the lower limit : "); int a = sc.nextInt(); System.out.print("Enter the upper limit : "); int b = sc.nextInt(); Main ob = new Main(); if(a<1 || b<1 || a>b) { System.out.println("Invalid Input"); } else { int c = 0; System.out.println("THE Prime Adam Numbers are:"); for(int i=a; i<=b; i++) { if(ob.isPrime(i) && ob.isAdam(i)) { c++; System.out.print(i + "\t"); } } if(c == 0) System.out.print("NIL"); System.out.println("\nThe Frequency of Prime Adam Number is:" + c); } } }
Output:
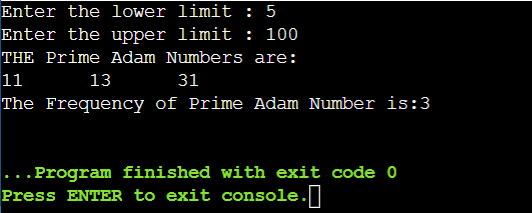
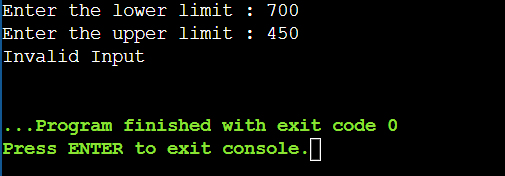
Explanation:
In the above program, we’ve written a login in which the compiler asks the user to enter the range in which you’ve to find prime adam numbers. When the user enters the range values i.e a and b then, the compiler first checks which numbers are prime. Then the second function checks which prime numbers are adam numbers. The numbers which satisfy both the conditions than that numbers are printed on the display.
The counter c in the program counts the number of prime adam numbers present in that range. That c variable is nothing but the frequency.
The upper limit is a and the lower limit is b.
Conclusion
So, in this way we’ve learned how to write the prime adam number program in java and also learned about prime and adam numbers. If you’ve any doubts then please feel free to contact us. We’ll reach you as soon as possible.
Thanks and Happy Coding 🙂
Also Read:
- Prime Number Program in C++
- Menu Driven Program in C
- Playfair Cipher Program in C
- Menu Driven Program in Java
- C Program to find the grade of a student using Switch Case
- Star Pattern Program in Java
- Anagram Program in Java