In this tutorial, we’ll learn how to write a menu-driven program in java using functions, while loop, and switch case. But before start writing the program we’ll first learn about menu-driven programs. What actually they are and where they’re used. So,
Table of Contents
What is Menu Driven Program in Java?
As the name suggests the menu driven program is a type of computer program which accepts the input from the user by showing a list of options. And users have to select any of the options to perform any operation. An example of a menu driven program is a washing machine which consists of a menu driven programmed microprocessor.
The second example of menu driven program in java is a Simple calculator which we used to perform some calculations. If we press ‘+’ for addition then the addition of two numbers case is executed. We can also do the calculations related to mensuration i.e calculating the area of the circle, the circumference of the cylinder, etc.
Menu driven programs are beneficial in a situation where the multitasking machines can perform a single task at a time. So without wasting any time we’ll see how to write menu driven program in java.
In this tutorial, we’ll code menu driven programs in java using different ways. Let’s see them one by one:
Menu Driven Program in Java Using While Loop
In this program, we’ll write a java program to perform string operations using a while loop.
//Learnprogramo - programming made simple import java.util.Scanner; public class Main { public static void main(String args[]) { int ch; String str,str1,str2; boolean str3; int length; Scanner sc = new Scanner(System.in); while(true) { // displaying the menu System.out.println("\n1: Length Of String"); System.out.println("2: String Concatenation"); System.out.println("3: String Comparison"); System.out.println("4: String Trimming"); System.out.println("5: Quit"); System.out.print("\n Make your choice: "); ch = sc.nextInt(); // reading user's choice switch (ch) { case 1: // for length of string sc.nextLine(); System.out.println("Enter string: "); str=sc.nextLine(); length = str.length(); System.out.println("Length of string: " + length); break; case 2: //for string concatenation sc.nextLine(); System.out.println("Enter First string: "); str=sc.nextLine(); System.out.println("Enter Second string: "); str1=sc.nextLine(); str2 = str.concat(str1); System.out.println("First String: "+ str); System.out.println("Second String: "+ str1); System.out.println("\nConcatenated string: "+ str2); break; case 3: // for string comparison sc.nextLine(); System.out.println("Enter First string: "); str=sc.nextLine(); System.out.println("Enter Second string: "); str1=sc.nextLine(); str3 = str.equals(str1); System.out.println("First String: "+ str); System.out.println("Second String: "+ str1); System.out.println("\nComparison Of String: "+ str3); break; case 4: // for string trimming sc.nextLine(); System.out.println("Enter string: "); str=sc.nextLine(); str1= str.trim(); System.out.println("\nTrimmed String:" + str1); break; case 5: System.exit(0); default: System.out.println("Invalid choice!!! Please make a valid choice. \n\n"); } } } }
Output:
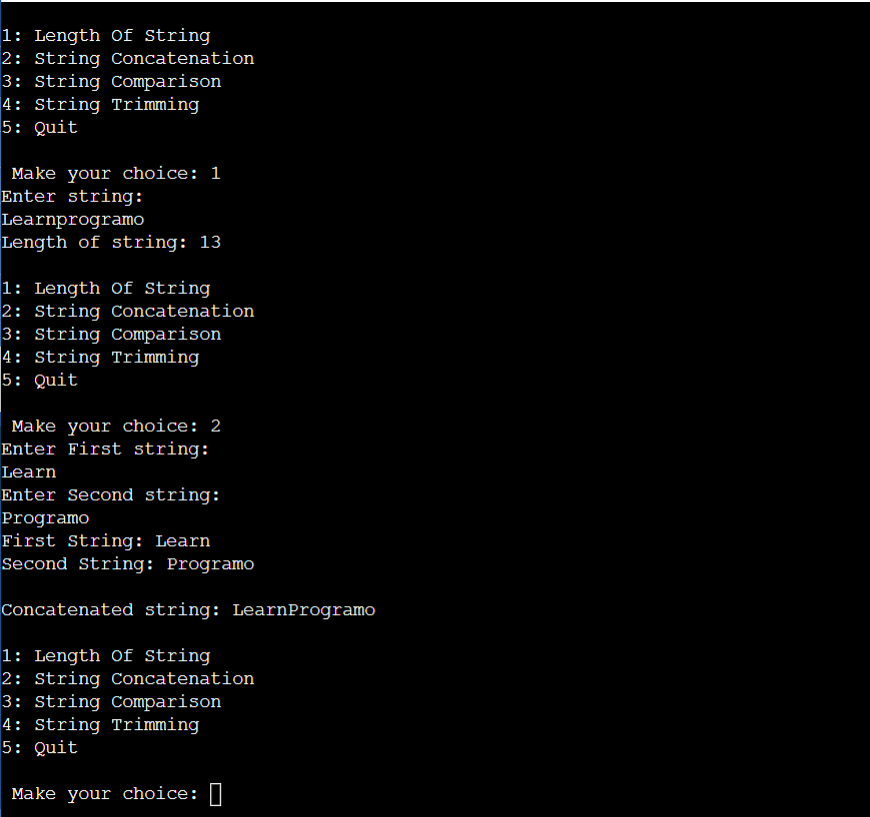
Explanation:
In the above program, we’ve used a while loop to perform string operations. After executing the program the compiler displays the list of menu options. And then the user has to choose the option by pressing the numbers which are assigned to the menu. If the user enters 1 and selects the Length of the string then the compiler asks the user to enter the string and then displays the output as the length of the entered string.
Similarly, after choosing the menu option 2nd the compiler asks the user to enter the first and the second string for concatenation. And after the concatenated string will be displayed on the screen.
If the user enters the wrong choice then the message “Invalid choice!!! Please make a valid choice.” will be displayed.
By choosing menu option 5 the program will be exited.
Menu Driven Program in Java Using Switch Case
In this program, we’ll build one simple calculator which accepts some input from the user and then perform calculations.
//Learnprogramo - programming made simple import java.util.Scanner; //Creating Class public class Main { //Creating main method public static void main(String[] args){ //Declaring all variables int a,b,c; int choice; Scanner scanner = new Scanner(System.in); //Creating infinite while loop while(true) { //Creating menu System.out.println("Press 1 for Addition"); System.out.println("Press 2 for Subtraction"); System.out.println("Press 3 for Multiplication"); System.out.println("Press 4 for Division"); System.out.println("Press 5 to Quit\n \n "); //Asking user to make choice System.out.println("Make your choice"); choice = scanner.nextInt(); //Creating switch case branch switch (choice) { //First case for finding the addition case 1: System.out.println("Enter the first number "); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a + b; System.out.println("The sum of the numbers is = " + c +"\n"); break; //Second case for finding the difference case 2: System.out.println("Enter the first number "); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a - b; System.out.println("The difference of the numbers is = " + c +"\n"); break; //Third case for finding the product case 3: System.out.println("Enter the first number"); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a * b; System.out.println("The product of the numbers is = " + c + "\n"); break; //Fourth case for finding the quotient case 4: System.out.println("Enter the first number"); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a / b; System.out.println("The quotient is = " + c +"\n"); break; //Fifth case to quit the program case 5: System.exit(0); //default case to display the message invalid choice made by the user default: System.out.println("Invalid choice!!! Please make a valid choice. \\n\\n"); } } } }
Output:
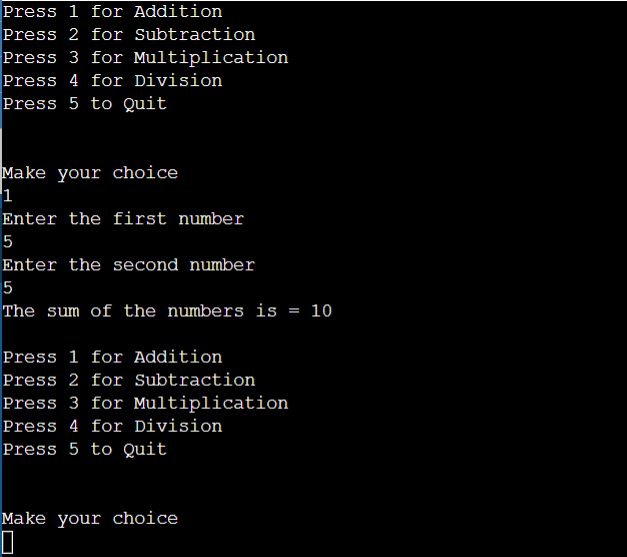
Explanation:
If we write a simple calculator program using c then we use a switch case to perform different operations. Similarly in Java, we can use a switch case to build a calculator using functions or while loops. In this program, we’re writing a program using a switch case. We’ll do the basic mathematical operations such as Addition, Subtraction, Multiplication, and Division. Users have to input 1 to perform Addition Similarly 2 for Subtraction, 3 for Multiplication, and 4 for Division.
In the program, the input entered by the user is stored in the choice variable. Then the compiler compares the choice variable with the numbers and jumps to the matched case. The cases used in this program accept input numbers to perform operations. If we want to perform the addition then the first and second number is taken by the user.
Then the compiler calculates the addition and prints the result on the screen. If the user enters the wrong choice then the message “Invalid choice!!! Please make a valid choice.” is printed. If the user wants to stop the execution of the program then he or she should choose menu option 5.
Menu Driven Program in Java Using Functions
In this program, we’ll write menu driven program in java using functions.
//Learnprogramo - programming made simple import java.util.Scanner; //Creating Class public class Main { //Declaring all the Variables and Scanner class static Scanner scanner = new Scanner(System.in); static double length,breadth,side,radius,area; static double pi =3.14; static int choice; //Creating main method public static void main(String[] args) { //Asking user to make a choice System.out.println("Enter 1 for Area of Rectangle"); System.out.println("Enter 2 for Area of Square"); System.out.println("Enter 3 for Area of Circle"); System.out.println("Enter 4 to Quit"); choice = scanner.nextInt(); //Creating switch block switch (choice) { //Creating cases and calling methods as per the users choice case 1: rectangleArea(); break; case 2: squareArea(); break; case 3: circleArea(); break; case 4: System.exit(0); default: System.out.println("You have entered the wrong choice\n"); } } //Method to evaluate the Area of Rectangle public static void rectangleArea(){ System.out.println("Enter the length of the Rectangle"); length=scanner.nextDouble(); System.out.println("Enter the breadth of the Rectangle"); breadth=scanner.nextDouble(); area=length*breadth; System.out.println("The Area of the Rectangle = "+area +"square unit"); } //Method to evaluate the Area of Square public static void squareArea(){ System.out.println("Enter the side of the Square"); side=scanner.nextDouble(); area = side*side; System.out.println("The area of the Square = "+area +"square unit"); } //Method to evaluate the Area of Circle public static void circleArea(){ System.out.println("Enter the readius of the Circle"); radius=scanner.nextDouble(); area=pi*radius*radius; System.out.println("The area of the Circle = "+area +"square unit"); } }
Output:
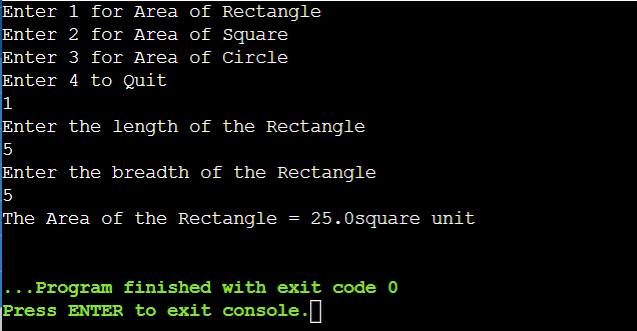
Explanation:
In the above program, we’ve used functions to write menu driven programs in java. After executing the above program the compiler will display the list of menu options. The user has to choose the option and enter it. The compiler will compare the input entered by the user and then call the function.
Suppose if the user enters input 1 then the compiler will store that input into choice and check for the condition choice==1 then the function rectangleArea() will be executed. The logic of calculating the area of a rectangle is written in the rectangleArea() function which accepts the length and the breadth and displays the area as the output.
After calculating the area the compiler displays the desired area of the circle on the screen. If you enter the wrong choice then the compiler will print the “You have entered the wrong choice” message.
After choosing menu option 4 the program will be stopped.
Frequently Asked Questions
What is Menu Driven Program in Java?
Ans: As the name suggests the menu driven program is a type of computer program which accepts the input from the user by showing a list of options. And users have to select any of the options to perform any operation.
What are Menu Driven Programs?
Ans: Menu driven programs are the type of codes that are written in java that accept the input from the user and then perform any task chosen from the menu list displayed.
How do you create a menu based program in Java?
Ans: The menu based program in java can be written using a switch case, while loops and functions.
What is menu driven function?
Ans: The menu driven function is a function that is called when the input entered by the user is matched with the menu option to perform any operation. This menu driven function is parameterized function which accepts the parameters and then performs any task.
Conclusion
In this way, we’ve learned how to write menu driven programs in java using switch case, while loop, functions. We’ve written three different programs to clearly understand the menu concept in java. If you have any doubts then please feel free to contact us. We’ll contact you as soon as possible.
Thanks and Happy Coding 🙂
Also Read: