Today we will learn leap year program in Java and how to write a program to find leap year in Java. So before start learning, you should have a little bit of knowledge of leap year. So,
Table of Contents
What is Leap Year?
Generally, a normal year has 365 days but, a leap year has 366 days i.e 1 day extra in the month of February. The Leap year occurs every 4 years.
Logic: The year which is divisible by 4, and 400 is called a leap year.If year divisible by 4 but not divisible by 100 is also called a leap year.
The year not divisible by 4 and 100 but divisible by 400 is not a leap year.
There are many ways to check whether the year is a leap year or not. So, we will see one by one.
1. Leap Year Program in Java Using If Statement
In this program, we will ask the user to input year which user want to check whether the entering year is a leap year or not.
//Learnprogramo import java.util.Scanner; public class learnprogramo { private static Scanner sc; public static void main(String[] args) { int year; sc = new Scanner(System.in); System.out.println("\n Please Enter any year you wish: "); year = sc.nextInt(); if (( year%400 == 0)|| (( year%4 == 0 ) &&( year%100 != 0))) { System.out.format("\n %d is a Leap Year. \n", year); } else { System.out.format("\n %d is NOT a Leap Year. \n", year); } } }
Output:
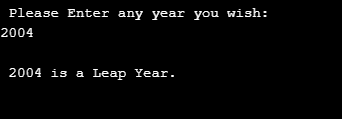
Program Logic:
In above program we have used a logic in if statement i.e (((year%4==0)&&(year%100!=0))||(year%400==0)).Here “||” is logical OR and “&&” is logical AND.
Our condition is (year%400==0).This condition will check whether the remainder is 0 or not. In the first condition (year%4) also check whether the remainder is 0 or not and if the condition gets FALSE then the program will exit.
Second Condition (year%100!=0).In this condition, if the remainder is not equal to 0 then it is a leap year. In Leap year definition we have defined that if the year which is divisible by 4 and 400 but not 100 is Leap Year.
2. Leap Year Program in Java Using else-if Statement
In this program, the user will enter any year and the program will check whether the year is leap year not using else-if statement.
//Learnprogramo import java.util.Scanner; public class learnprogramo { private static Scanner sc; public static void main(String[] args) { int year; sc = new Scanner(System.in); System.out.println("\n Please Enter any year you wish: "); year = sc.nextInt(); if ( year % 400 == 0) { System.out.format("\n %d is a Leap Year. \n", year); } else if (year%100 == 0) { System.out.format("\n %d is NOT a Leap Year. \n", year); } else if(year%4 == 0) { System.out.format("\n %d is a Leap Year. \n", year); } else { System.out.format("\n %d is NOT a Leap Year. \n", year); } } }
Output:
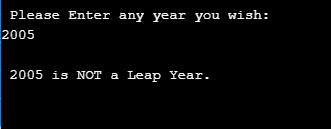
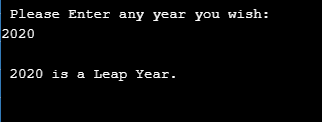
In the above program, we have just divided the logic and declared it using the else-if statement. But the logic behind the program is the same.
3. Using Nested if-else
Now in this program, we will ask the user to enter the year and the program will perform its operations using nested if statements.
//Learnprogramo import java.util.Scanner; public class Main { private static Scanner sc; public static void main(String[] args) { int year; sc = new Scanner(System.in); System.out.println("\n Please Enter any year you wish: "); year = sc.nextInt(); if ( year % 4 == 0) { if (year%100 == 0) { if(year%400 == 0) { System.out.format("\n %d is a Leap Year. \n", year); } else { System.out.format("\n %d is NOT a Leap Year. \n", year); } } else { System.out.format("\n %d is a Leap Year. \n", year); } } else { System.out.format("\n %d is NOT a Leap Year. \n", year); } } }
Output:
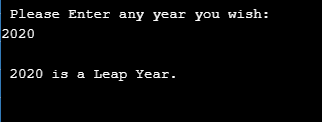
Condition 1st:(year%4) if the condition is false the given number is not leap year but the condition is true then we have to check for century year. So the compiler goes to the next condition.
Condition 2nd:(year%400) if the condition is false the year is not a leap. But, if the condition is true then the compiler will jump to the next condition.
Condition 3rd: This is the final condition which decides whether the entered year is a leap year or not. If (year%100!=0) then the year is a leap year, else year is not a leap.
4. Using Class
In this program, we will use class LeapYear to check whether the entered year is a leap year or not.
//Learnprogramo public class LeapYear { public int CheckLeapYear(int year) { if (( year%400 == 0)|| (( year%4 == 0 ) && ( year%100 != 0))) { return year; } else { return 0; } } }
We have to create a separate class LeapYear.java. Now we will create an object of Leapyear in the main class where we will check whether the entered year is Leap Year or not.
//Learnprogramo import java.util.Scanner; public class learnprogramo { private static Scanner sc; public static void main(String[] args) { int year, leap; sc = new Scanner(System.in); System.out.println(" Please Enter any year you wish: "); year = sc.nextInt(); LeapYear ly = new LeapYear(); leap = ly.CheckLeapYear(year); if(leap != 0) { System.out.format("\n %d is a Leap Year. \n", year); } else { System.out.format("\n %d is NOT a Leap Year. \n", year); } } }
Conclusion:
So we have learned about Leap Year Program in Java using four different methods.
Also Read:
- How to return an array in java.
- Convert int to string in java
- How to convert string to integer in java.
- 20 different star pattern programs in java.
- 20 different number pattern programs in java.
- How to reverse a string in java.
- Anagram Program in Java.