We can convert Int to String in Java using Integer.toString(), String.valueOf(), Integer(int).toString() methods. The Integer can also be converted into String using DecimalFormat, StringBuffer and String.format() method.
So Today we will learn different methods for How to Convert Int to String in Java. The methods are as follows:
- Using Integer.toString(int)
- Converting Using String.valueOf(int).
- Using Integer(int).toString().
- Convert Using String.format().
- DecimalFormat.
- StringBuffer or StringBuilder.
- Using Special Radix.
These methods are as same as we convert String to Integer in Java. Now we will learn the above methods one by one.
Table of Contents
1. How to Convert Int to String in Java Using Integer.toString(int)
In this program, we will make use of java inbuilt method called Integer.toString(int). So, first of all
What is Integer.toString() Method?
This is a java inbuilt method which returns the String objects representing the Integer values.
Syntax: public static String toString(int i)
Here the argument i is returned and converted to the string and the Sign is preserved if the given number is negative.
Now we will see how the program is written in java.
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int a = 5678; int b = -5678; String str1 = Integer.toString(a); String str2 = Integer.toString(b); System.out.println("String str1 = " + str1); System.out.println("String str2 = " + str2); } }
Output:
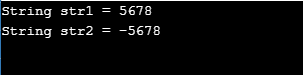
2. How to Convert Int to String in Java Using String.valueOf(int)
In the previous program, we use Integer.toString(int) method which returns the string object. But in this program, we will use another java inbuilt method called String.valueOf().
What is String.valueOf() Method?
With the help of String.valueOf() method we can convert int to string, long to a string, boolean to a string, float to string etc. Generally, it returns the string representations.
Syntax: String.valueOf(boolean b), String.valueOf(char ch).
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { String str3 = String.valueOf(5678); System.out.println("String str3 = " + str3); } }
Output:
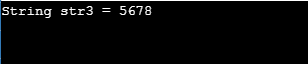
3. Using Integer(int).toString() Method
This is an alternative to Integer.toString(int) method. But the only difference is, we use Integer.toString(int) when our variable is in a primitive type.
And If the variable is already an instance of Integer then we use Integer(int).toString() method.
Syntax: String j = obj.toString().
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int n = 5678; Integer obj = new Integer(n); String j = obj.toString(); System.out.println("String j = " + j); } }
Output:
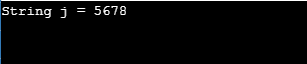
4. How to Convert Int to String in Java Using String.format() Method
This method was first introduced in JDK 1.5 release. The main purpose of this method is to format given arguments into the string.
Syntax: public static String format(Locale L, String Format, Object…args) Where,
L= locale which is addressed during formatting.
format= Which includes the format specifier. and
args= the arguments which refer to the format specifier.
Now let us see how the java program is written.
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int i=500; String s=String.format("%d",i); System.out.println(s); } }
Output:

5. Using the DecimalFormat Method
This is a type of class which comes under java.text package. The main motive of this class is to format given number into the string.
With the help of DecimalFormat method, we can also specify the no of decimal places and the comma for number separation.
Syntax: DecimalFormat obj=new DecimalFormat(“$”)
Before start writing a program, we need to import java.text.DecimalFormat.
//Learnprogramo import java.text.DecimalFormat; public class Learnprogramo { public static void main(String args[]) { int j = 56789; DecimalFormat obj = new DecimalFormat("#,###"); String str = obj.format(j); System.out.println(str); } }
Output:
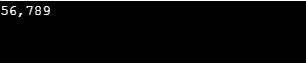
6. Using StringBuffer or StringBuilder
These are the two types of classes which concatenate multiple values in a single string. StringBuffer is slower than the StringBuilder but StringBuilder is not Thread-safe than StringBuffer.
So In this program, we will convert Int to String in Java Using StringBuffer and StringBuilder.
Using a StringBuffer:
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int f = 12345; StringBuffer sb = new StringBuffer(); sb.append(f); String str = sb.toString(); System.out.println("String str = " + str); } }
Output:

Using a StringBuilder:
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int f = 12345; StringBuilder sb = new StringBuilder(); sb.append(f); String str = sb.toString(); System.out.println("String str = " + str); } }
Output:
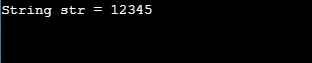
In the above program, the append method is used to add a new argument at the end of the string.
7. Using Special Radix Method
In this method, all of the examples use the radix base as 10. These are the methods where we will convert into binary, octal and hexadecimal.
Binary Method:
The Numbers are said to be Binary if their base is 2. Basically, they are in the form of ‘0’ and ‘1’.
Now let us see how to convert binary numbers into a string.
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int j = 500; String binaryString = Integer.toBinaryString(j); System.out.println(binaryString); } }
Output:
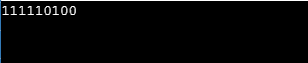
Octal Method:
The number is said to be Octal if and only if that number’s base is 8. It uses digits from 0 to 7.
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int j = 500; String octalString = Integer.toOctalString(j); System.out.println(octalString); } }
Output:
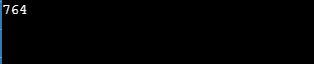
Hexadecimal Method:
The number with base 16 is said to be as Hexadecimal Number. It contains numbers from ‘0’ to ‘9’ and Alphabets ‘A’ to ‘F’ represents numbers from ’10’ to ’15’.
//Learnprogramo public class Learnprogramo { public static void main(String args[]) { int j = 500; String hex = Integer.toHexString(j); System.out.println(hex); } }
Output:
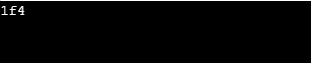
Also Read:
- Different Star Pattern Program in Java.
- Number Pattern Program in java.
- Program to reverse a String in Java.
- How to return an array in java.