Table of Contents
What are Functions in C?
A function is defined as “a named independent or self-contained block of C code that performs a specific task and optionally returns a value to the Calling program or may receive values from the calling program”.
Some programs might have thousands or millions of lines and to manage such programs it becomes quite difficult as there might be too many syntax errors or logical errors present in the program. To manage such type of programs, the concept of modular programming is used.
The entire program is divided into a series of modules and each module is intended to perform a particular task. The detailed work to be solved by the module is described in the module (subprogram) only and the main program only contains a series of modulus that are to be executed.
Modular programming emphasis on breaking of large programs into small problems to increase the maintainability, readability of the code and to make the program handy to make any changes in future or to correct the errors.
Sometimes there is a need for us repeatedly to execute One block of statements in one place of the program, loop statements can be used in such case.
But, a block of statements need to be repeatedly executed in many parts of the program, then repeated coding, as well as wastage of the vital Computer resource memory, will be wasted.
Introduction
If we adopt the modular programming technique, these disadvantages can be eliminated. The modules incorporated in C are called the functions and each function in the program is meant for doing a specific task. C functions are easy to use and very efficient also.
In this chapter, We will see how functions are defined and how they are accessed from various places within a C program. We will then consider the manner in which information is passed to a function, recursive functions etc.
Concept of Function:
Basically a function has the following characteristics:
Unique Name: A function is named with a unique name.
Performs a Specific Task: The task is a discrete job that the program must perform as part of its overall operation, such as sorting an array into numerical order, or calculating a cube root, etc.
Independent: A function can perform its task without interference from or interfering with other parts of the program.
May Receive Values from the Calling Program: Calling program can pass values to function for processing whether directly or indirectly.
May Return a Value to the Calling Program: The called function may pass something back to the calling program.
As we already know, every program consists of one or more functions. One of these functions must be called main().
Execution of the program will always begin by carrying out the instructions in main( ). Additional functions will be subordinate to main( ), and perhaps to one another.
If a program contains multiple functions, their definitions may appear in any order, though they must be independent of one another. That is, one function definition cannot be embedded within another.
Types of Functions in C
There are two types of Functions in C they are explained below:
Predefined or Standard Library Functions in C:
These are the functions which come along with compiler and placed in libraries or in header files (.h files like <stdio.h>).
We just call them whenever there is a need to use them. Examples, puts( ), gets( ), printf( ), scanf( ) etc.
User Defined Functions:
The functions we create for specific task is known as user defined functions.
1. Standard Library Functions in C
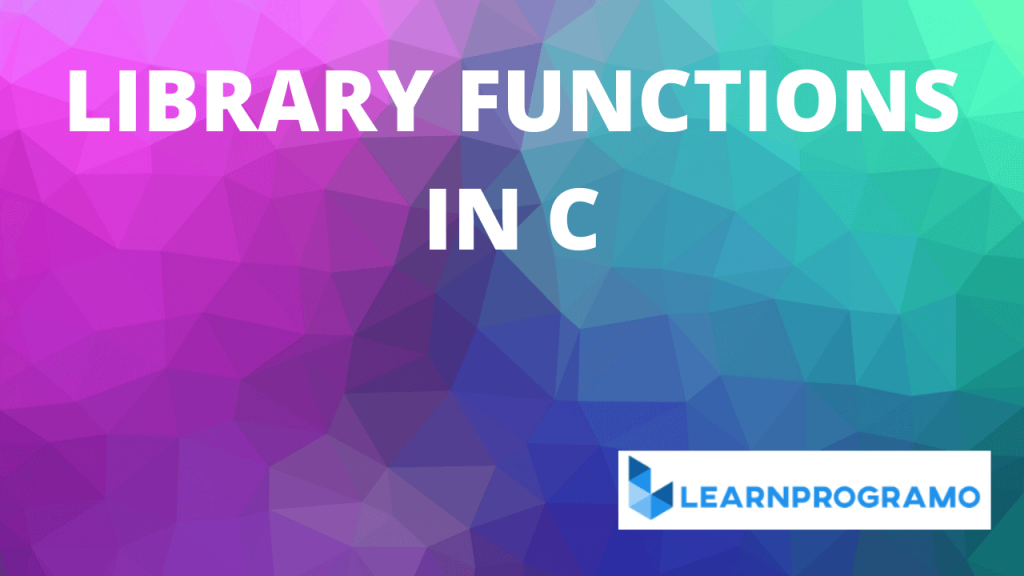
C language has the facility to provide library functions for performing some operations. These functions are present in the C library and they are predefined.
The prototype and definitions of the functions are present in their respective header files and must be included in your program to access them.
To use a library function we have to include corresponding header file using the preprocessor directive #include.
For example, to use input output functions like printf( ) and scanf( ) we have to include <stdio.h>, for mathematical library functions we have to include math.h.
Let us see some predefined functions from <ctype.h>. All character handling functions are defined in this header file.
These functions take the int equivalent of one character as a parameter and return an int that can either be another character or a value representing a boolean value namely true or false.
Function Name and Description
Function Name | Description |
---|---|
1. int isalnum(int c) | Checks Whether the passed character is alphanumeric. |
2. int isalpa(int c) | Checks whether passed character is alphabetic. |
3. int iscntrl(int c) | Checks whether passed character is control character. |
4. int isdigit(int c) | Checks whether passed character is decimal digit. |
5. int isgraph(int c) | Checks whether passed character has graphical representation using locale. |
6. int islower(int c) | Checks whether passed character is lowercase letter. |
7. int isprint(int c) | Checks whether passed character is printable. |
8. int ispunct(int c) | Checks whether the passed character is a punctuation character. |
9. int isspace(int c) | Checks whether the passed character is white space. |
10. int isupper(int c) | Checks whether passed character is an uppercase letter. |
11. int isxdigit(int c) | Checks whether the passed character is a hexadecimal digit. |
The library also contains two conversion functions that accept and returns an “int”.
Function Name | Description |
---|---|
int tolower(int c) | converts uppercase letters to lowercase. |
int toupper(int c) | converts lowercase letters to uppercase. |
Math.h Functions:
Let us consider the functions defined in the header <math.h>. The <math.h> header defines various mathematical functions.
All the functions available in this library take double as an argument and return double as the result.
Function Name | Description |
---|---|
double cos(double x) | Return the cosine of radian angle x. |
double sin(double x) | Return the sine of a radian angle x. |
double exp(double x) | Returns the value of e raised to the xth power. |
double log(double x) | Returns the natural logarithm(base e) of x. |
double log10(double x) | Returns the common logarithm(base 10) of x. |
double pow(double x, double y) | Returns x raised to the power of y. |
double sqrt(double x) | Returns the square root of x. |
double cei(double x) | Returns the smallest integer value greater than equal to x. |
double fabs(double x) | Returns the absolute value of x. |
double floor(double x) | Returns the largest integer value less than or equal to x. |
double fmod(double x, double y) | Returns the remainder of x divided by y. |
2. User defined Functions in C
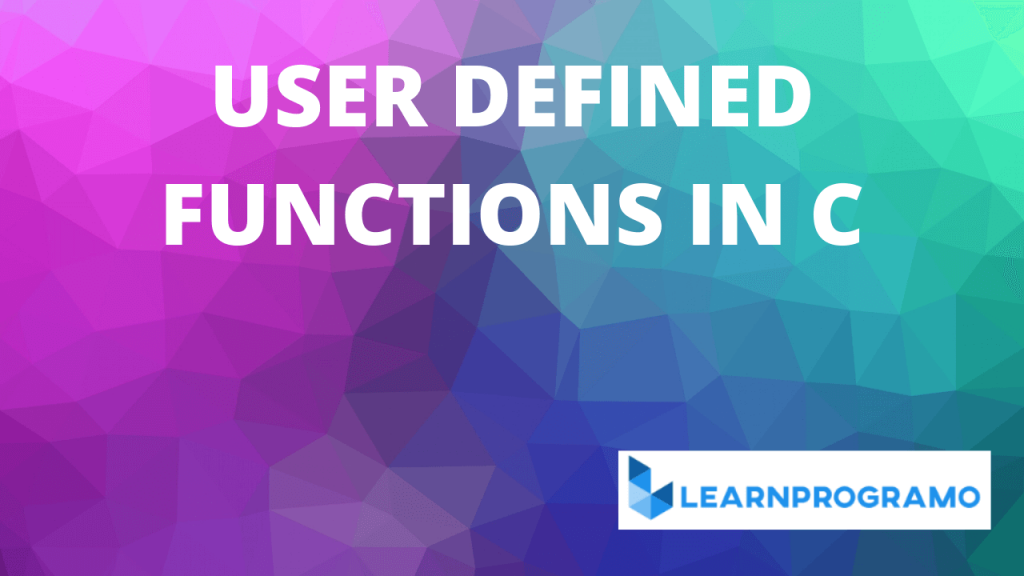
user can create their own functions for performing any specific task of the program. These types of functions are called user-defined functions in C.
To define user-defined functions we have to follow three steps:
1st Step: Function Declaration or function prototype.
2nd Step: Function definition or function body.
3rd Step: Calling of function.
Function Declaration or Function Prototype
A function declaration tells the compiler about a function name and how to call the function. Declaring of a function can be called a function prototype.
Function declaration informs the compiler about the following three things:
Name of the function, Number and type of argument received by the function and the type of value returned by the function.
A function declaration tells the compiler that a function with these features will be defined and used later in the program.
Function declaration Syntax is as follows:
return_type function_name(datatype arg1, datatype arg2,…datatype argn);
Where,
return type: Specifies the data type of value that the function should return to the calling program. It can be any of data types like char, float, int, long, double etc. If there is no return value, specify a return type of void.
function name: It can have any name as long as the rules for C variable names are followed and must be unique.
parameter list: Parameter or argument list contains variables names along with their data types. These arguments are kind of inputs for the function.
A function can accept any of C basic data types. This parameter list just acts as a placeholder. If a function does not receive any values, parameter-list can be declared as void.
Example:
A function which is used to add two integer variables will be having two integer arguments. For example, the function declaration is as follows:
int max(int a, int b);
Parameter names are optional in function declaration only their type is required, so the following is also a valid declaration:
int max(int, int);
Function Definition
Function definition contains the block of code to perform a specific task. It may include list of the arguments, return variable and local declarations.
Syntax of defining a function is as follows:
return_type function_name (datatype arg1, datatype arg2,…,datatype argn)
{
local variable declaration;
set of statements – Block of code
}
where the first line of a function definition must be the same as function header or prototype. Second-line may be variable or constant declaration local to that function. Set of statements perform some specific task.
The function may contain a special statement called return statement to return value from a function. The return statement is optional. It may be absent if the function does not return any value.
Example:
int max(int a, int b) { int result; if(a>b) result=a; else result=b; return result; }
The function definition can be placed anywhere in the program. But generally, all definitions are placed after the main( ) function. If the function definition is placed before the main( ) function, then function declaration s not needed.
Note that a function definition cannot be placed inside another function definition.
Conclusion:
In this chapter we have learned about what are functions in c now in the next chapter we will learn function calling methods in C.