Table of Contents
Compilation Process in C
Programs written in any high-level programming language must be translated to the machine language as a representation of instructions for the computer to execute them. This process is called a Compilation Process in C.
And the program performing the compilation job or task is called the compiler.
The compilation is the process of automatic translation of the code written in the programming language to the machine code.
The C language compilation process consists of :
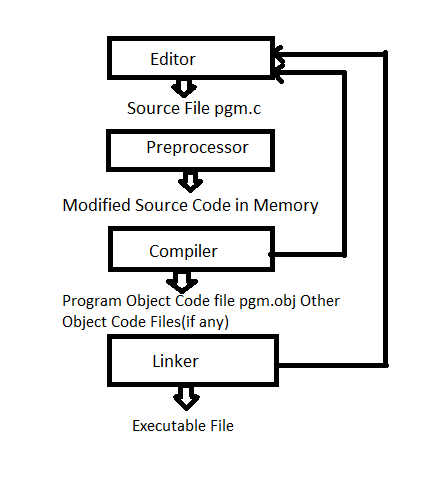
1. Editor:
A text editor is usually used to enter the C program into a file. For example, vi is a popular text editor used on Linux systems.
The program that is entered into the file is known as the source program because it represents the original form of the program expressed in the C language.
After the source program has been entered into a file you can then proceed to have it compiled.
The language translator converts the high-level language program into the low-level language program called object code.
2. Preprocessor:
The C Pre-processor, as its name implies, processes before the processing of C source program. It is a program that processes the source code before the compiler translates the source code into the object code.
During processing, these preprocessors perform some modifications on the source code based on the instruction provided by the preprocessors.
These instructions are known as preprocessor commands or directives. Each of the preprocessor directives begins with a # symbol and do not require semicolon(;) at the end.
The preprocessor is used to make the source code more readable, much easy to modify, portable and more efficient. Preprocessor directives are placed in the source program before calling the function main()
A preprocessor mainly performs the following tasks on the HLL code:
Removing Comments: It removes all the comments. A comment is written only for humans to understand the code.
File Inclusion: Including all the files from the library that our program needs. In HLL we write #include which is a directive for the preprocessor that tells it to include the contents of the library file specified. For example, #include will tell the preprocessor to include all the contents in the library file stdio.h.
Macro Expansion: Macros can be called as small functions that are not as overhead to the process. If we have to write a function (having a small definition) that needs to be called recursively (again and again), then we should prefer a macro over a function.
3. Compiler:
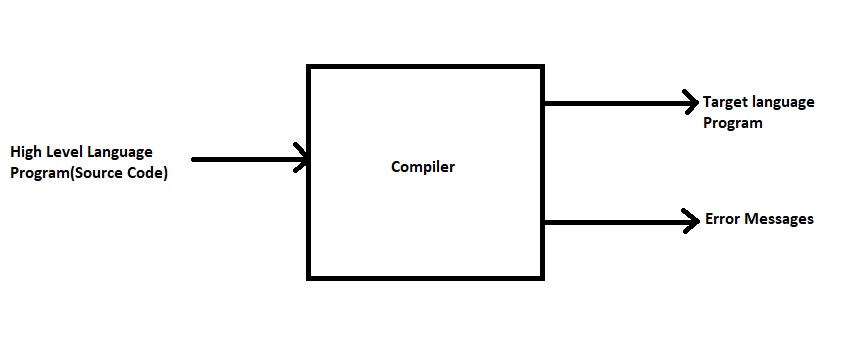
A compiler is a program that translates the instruction of a high-level language (source program) into machine language (object program).
A compiler is a program or set of programs that converts source code written in a high-level language to low-level language (assembly language or machine language).
A programming language can have many compilers. For example, GCC C, Turbo C, Quick C etc. are different compilers for C programming language.
Need Of Compiler for Compilation Process in C
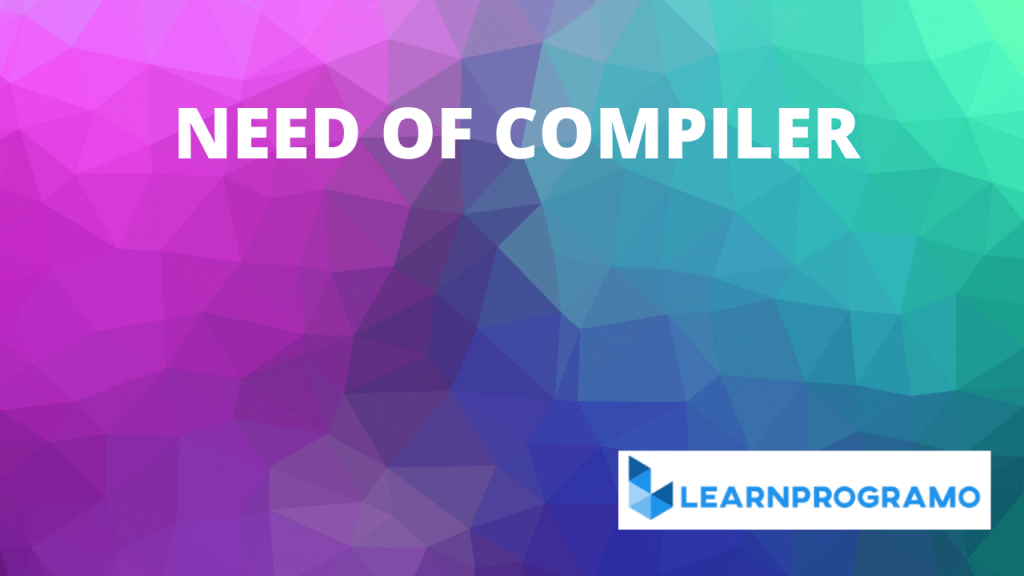
A computer understands only binary language and executes instructions coded in binary language. It cannot execute a single instruction given in any other form. Therefore, we must provide instructions to the computer in binary language.
Means we must write computer programs entirely in the binary language (sequence of 0’s and 1’s), and it is not possible to write the program in binary language.
So, there was a need of a translator that translates the computer instructions given in the English language to binary language. Hence, to accomplish the job of a translator compiler was invented.
The Compiler can also perform the following tasks:
Performs a pre-processing of source code. Gather all files required for the source code to compile.
Parses the entire source code. Checks for any syntax errors in the source code.
Performs a thorough syntax analysis of the source code. To understand the structure and semantic of the source code.
Optionally translates the source program (source code) code in an intermediate code known as object code to enhance Compiler Target language program (Machine object code) messages the performance.
Translates the object code to a binary language known as executable code.
Advantages of Compiler:
The object program can be used whenever required without the need for recompilation.
Source code is not included by the compiler, therefore compiled code is more secure than interpreted code.
The compiler produces an executable file, and therefore the program can be run without the need of the source code.
Disadvantages of Compiler:
Object code needs to be produced before a final executable file, this can be a low process.
When an error is found, the whole program has to be re-compiled again and again.
Interpreter:
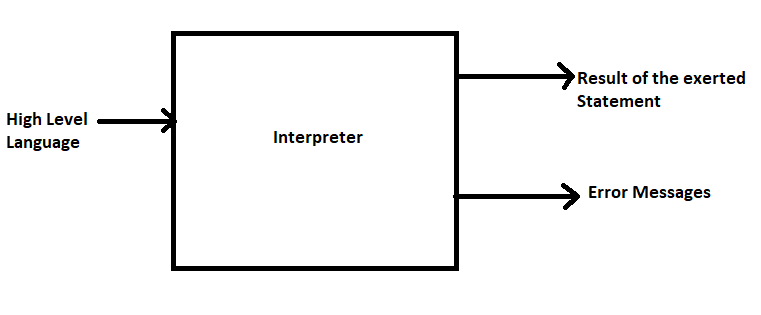
programming languages are implemented in two ways interpretation and compilation, An interpreter is a computer program that is used to directly execute program instructions written using one of the many high-level programming languages.
The interpreter transforms the high-level program into an intermediate language that it then executes, or it could parse the high-level source code and then performs the commands directly, which is done line by line Or statement by statement.
An interpreter also produces a low-level program from a high-level program, but the working of the interpreter is not High language similar to that of the compiler.
An interpreter processes the high-level program line-by-line and simultaneously, produces the low-level program.
Advantages of Interpreter:
Debugging (check errors) is easier since the interpreter stops when it finds an error.
Easier to create multi-platform (run on the different operating system) code. as each different platform would have an interpreter to run the same source code. Compilation Process in C.
If an error is found then there is no need to retranslate the whole program like a compiler.
Disadvantages of Interpreter:
Interpreters are generally slower than compiled programs because interpreter translates one line at a time.
Source code is required for the program to be executed, and this source code can be read by any other programmer so it is not a secured.
5. Loader or Linker:
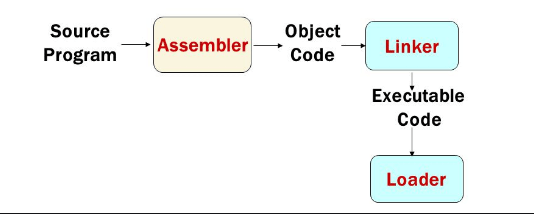
A loader loads the programs into the main memory from the storage device. The OS transparently calls the loader when needed.
A loader is a program used by an operating system to load programs from a secondary to main memory so as to be executed.
Linker links and combines objects generated by a compiler into a single executable. A linker is also responsible to link and combine all modules of a program if written separately.
Usually, large applications are written into small modules and are then compiled into object codes. A linker is a program that combines these object modules to form an executable.
The linker is a program that links together several object modules and libraries to form a single, coherent, executable program.
The loader is a program that brings an executable file residing on a disk into memory. Loading places data and machine instructions into the memory. Compilation Process in C.
Loader is a program that performs the functions of a linker and then immediately schedules the executable code for execution, Without necessarily creating an executable file as an output.