Today we will learn how to construct different star pattern programs in java. So before start learning pattern program in java, we will make a quick overview of the star pattern definition.
Table of Contents
What are Star Patterns?
Basically the Star Patterns are the series of stars which are arranged in a particular order. These patterns are created by arranging the stars which are similar to the number patterns in java.
For Example:

Every interview starts with pattern programs. So it is mandatory to learn the logic behind these star patterns. These patterns are best suited to increase your logical thinking ability.
We have structured these pattern programs in such a way that the beginners and the professionals can easily understand and practice with their own.
So after learning these programs, your duty is to understand the logic behind each pattern. Don’t go fast spend time to understand each program which will be beneficial for you.
So without wasting time, we will start learning star patterns one by one. If you have any doubt then please feel free to express your doubt in the comment section below.
Different Star Pattern Programs in Java
1. Star Pattern 1:
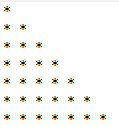
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=0; i< rows;i++ ) { for (int j=0; j<=i; j++) { System.out.print("*" + " "); } System.out.println(); } //Close the resources sc.close(); } }
2. Star Patter 2:
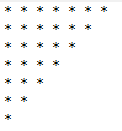
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= rows-1; i>=0 ; i--) { for (int j=0; j<=i; j++) { System.out.print("*" + " "); } System.out.println(); } //Close the resources sc.close(); } }
3. Star Pattern 3:
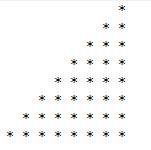
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows; i++) { for (int j=1; j<=rows-i; j++) { System.out.print(" "); } for (int k=0;k<=i;k++) { System.out.print("*"); } System.out.println(""); } //Close the resources sc.close(); } }
4. Star Pattern 4:
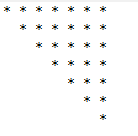
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= rows; i>= 1; i--) { for (int j=rows; j>i;j--) { System.out.print(" "); } for (int k=1;k<=i;k++) { System.out.print("*"); } System.out.println(""); } //Close the resources sc.close(); } }
5. Star Pattern 5:
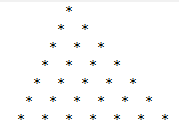
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=rows-1; j>i; j--) { System.out.print(" "); } for (int k=0; k<=i; k++) { System.out.print("*" + " "); } System.out.println(); } //Close the resources sc.close(); } }
6. Star Pattern 6:
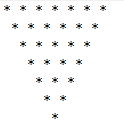
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j<=i; j++) { System.out.print(" "); } for (int k=0; k<=rows-1-i; k++) { System.out.print("*" + " "); } System.out.println(); } //Close the resources sc.close(); } }
7. Star Pattern 7:
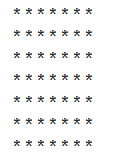
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=rows-1; j>i; j--) { System.out.printf("*"+" "); } for (int k=0; k<=i; k++) { System.out.print("*" + " "); } System.out.println(""); } //Close the resources sc.close(); } }
8. Star Pattern 8:
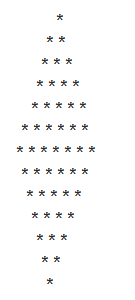
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=rows-1; j>=i; j--) { System.out.print(" "); } for (int k=0; k<=i; k++) { System.out.print("*" + " "); } System.out.println(""); } for (int i= 0; i<= rows-1 ; i++) { for (int j=-1; j<=i; j++) { System.out.print(" "); } for (int k=0; k<=rows-2-i; k++) { System.out.print("*" + " "); } System.out.println(""); } //Close the resources sc.close(); } }
9. Star Pattern 9:
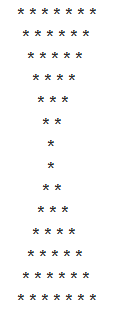
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j <i; j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*" + " "); } System.out.println(""); } for (int i= rows-1; i>= 0; i--) { for (int j=0; j < i ;j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*" + " "); } System.out.println(""); } //Close the resources sc.close(); } }
10. Star Pattern 10:
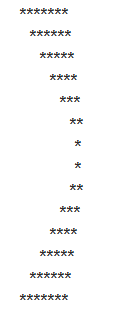
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j < i ;j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*"); } System.out.println(""); } for (int i= rows-1; i>= 0; i--) { for (int j=0; j<i ;j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*"); } System.out.println(""); } //Close the resources sc.close(); } }
11. Star Pattern 11:
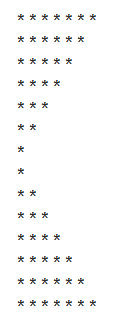
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= rows-1; i>= 0; i--) { for (int j=i; j>=0; j--) { System.out.print("*"+ " "); } System.out.println(""); } for (int i=0; i<= rows-1; i++) { for(int j=i; j >= 0;j--) { System.out.print("*"+ " "); } System.out.println(""); } //Close the resources sc.close(); } }
12. Star Pattern 12:
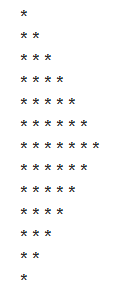
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j<=i; j++) { System.out.print("*"+ " "); } System.out.println(""); } for (int i=rows-1; i>=0; i--) { for(int j=0; j <= i-1;j++) { System.out.print("*"+ " "); } System.out.println(""); } //Close the resources sc.close(); } }
13. Star Pattern 13:
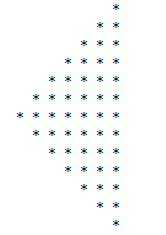
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 1; i<= rows ; i++) { for (int j=i; j < rows ;j++) { System.out.print(" "); } for (int k=1; k<=i;k++) { System.out.print("*"); } System.out.println(""); } for (int i=rows; i>=1; i--) { for(int j=i; j<=rows;j++) { System.out.print(" "); } for(int k=1; k<i ;k++) { System.out.print("*"); } System.out.println(""); } //Close the resources sc.close(); } }
14. Star Pattern 14:
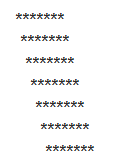
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i= 1; i<= rows ; i++) { for (int j=1; j< i ;j++){ System.out.print(" "); } for (int k=1; k<=rows ;k++) { System.out.print("*"); } System.out.println(""); } //Close the resources sc.close(); } }
15. Star Pattern 15:
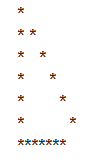
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=1; i<= rows ; i++) { for (int j=1; j<= i ;j++){ if(j==1 || j==i || i==rows) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(""); } //Close the resources sc.close(); } }
16. Star Pattern 16:
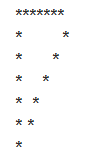
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=1; i<= rows ; i++) { for (int j = rows; j >= i; j--) { if (j == rows || j == i || i == 1) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(""); } //Close the resources sc.close(); } }
17. Star Pattern 17:
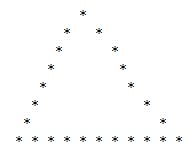
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=1; i<= rows ; i++) { for (int j = i; j < rows ; j++) { System.out.print(" "); } for (int k = 1; k <= (2*i -1) ;k++) { if( k==1 || i == rows || k==(2*i-1)) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(""); } //Close the resources sc.close(); } }
18. Star Pattern 18:
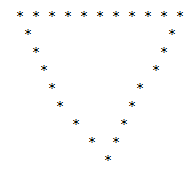
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=rows; i>= 1 ; i--) { for (int j = i; j < rows ; j++) { System.out.print(" "); } for (int k = 1; k <= (2*i -1) ;k++) { if( k==1 || i == rows || k==(2*i-1)) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(""); } //Close the resources sc.close(); } }
19. Star Pattern 19:
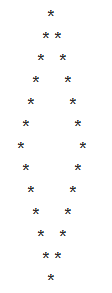
Program:
import java.util.Scanner; public class MainClass { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //Taking rows value from the user System.out.println("How many rows you want in this pattern?"); int rows = sc.nextInt(); System.out.println("Here is your pattern....!!!"); for (int i=1; i<= rows ; i++) { for (int j = rows; j > i ; j--) { System.out.print(" "); } System.out.print("*"); for (int k = 1; k < 2*(i -1) ;k++) { System.out.print(" "); } if( i==1) { System.out.println(""); } else { System.out.println("*"); } } for (int i=rows-1; i>= 1 ; i--) { for (int j = rows; j > i ; j--) { System.out.print(" "); } System.out.print("*"); for (int k = 1; k < 2*(i -1) ;k++) { System.out.print(" "); } if( i==1) System.out.println(""); else System.out.println("*"); } //Close the resources sc.close(); } }
20. Star Pattern 20:
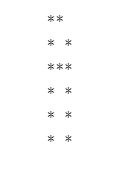
Program:
import java.util.Scanner; public class MainClass { // Java program to print alphabet A pattern void display(int n) { // Outer for loop for number of lines for (int i = 0; i<=n; i++) { // Inner for loop for logic execution for (int j = 0; j<= n / 2; j++) { // prints two column lines if ((j == 0 || j == n / 2) && i != 0 || // print first line of alphabet i == 0 && j != n / 2 || // prints middle line i == n / 2) System.out.print("*"); else System.out.print(" "); } System.out.println(); } } public static void main(String[] args) { Scanner sc = new Scanner(System.in); MainClass a = new MainClass(); a.display(7); } }
So this is the pattern program in java which are widely used in java and interviews. If you learn all the above pattern then your base of programming will become perfect. Hope you understand all the programs have a nice day…
If you have any problems or doubt then please mention in the below comment box.