In this tutorial, we’ll see how to write Array Reversal in C Hackerrank Solution with an explanation. If you’re wondering how to solve array reversal in the C Hackerrank solution, then you’re at the right place. Here you’ll get all Hackerrank solutions with the explanation. But, before getting started we’ll first let you know about Hackerrank. So,
Table of Contents
What is Hackerrank?
Hackerrank is a tech company that mainly focuses on competitive programming challenges for students, companies, and consumers. Hackerrank was founded by two founders Vivek Ravishankar and Harishankar Karunanidhi in the year 2012. Its headquarters are situated in California, United States.
With the help of this platform students as well as professionals prepare themselves for the placements by practicing the coding problems. The questions that hacker rank contains are asked in the interview rounds of many companies.
So let us code the Array Reversal in C Hackerrank solution with an Explanation.
Array Reversal in C Hackerrank Problem
Problem Statement
Given an array of size n, reverse it.
Example: If an array, arr = [1, 2, 3, 4, 5], after reversing it the array should be arr = [5, 4, 3, 2, 1].
Input Format
The first line contains an integer n, denoting the size of the array. The next line contains n space-separated integers denoting the elements of the array.
Constraints
1 <= n <= 1000
1 <= arri <= 1000, where arri is the ith element of the array.
Output Format
The output is handled by the code given in the editor, which would print the array.
Sample Input 0
6
16 13 7 2 1 12
Sample Output 0
12 1 2 7 13 16
Explanation 0
Given array, arr = [16, 13, 7, 2, 1, 12]. After reversing the array, arr = [12, 1, 2, 7, 13, 16].
1st Sample Input
7
1 13 15 20 12 13 2
1st Sample Output
2 13 12 20 15 13 1
Sample Input 2
8
15 5 16 15 17 11 5 11
Sample Output 2
11 5 11 17 15 16 5 15
Array Reversal in C Hackerrank Solution 1
//Learnprogramo - programming made simple #include <stdio.h> #include <stdlib.h> int main() { int num, *arr, i; scanf("%d", &num); arr = (int*) malloc(num * sizeof(int)); for(i = 0; i < num; i++) { scanf("%d", arr + i); } int temp; for (i = 0; i < num / 2; i++) { temp = (int) *(arr + num - i - 1); *(arr + num - i - 1) = *(arr + i); *(arr + i) = temp; } for(i = 0; i < num; i++) printf("%d ", *(arr + i)); return 0; }
Output:
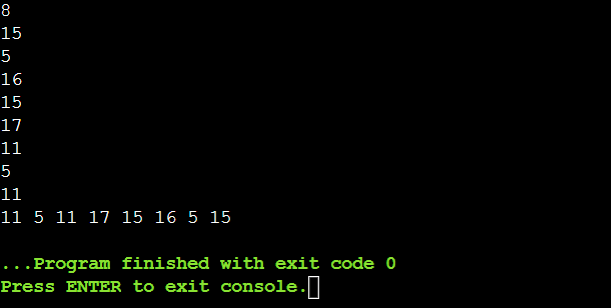
Explanation:
Reversing an array means changing the positions of the numbers in such a way that the last number comes in the first position, the second last in the second position, and so on.
So, in the above program, we’ve coded the program in c language. After compiling the code, the compiler will first ask you to enter the size of the array. Then you’ve to enter the numbers and then at last the program will reverse the array and print it on the desktop.
Array Reversal in C Hackerrank Solution 2
//Learnprogramo - programming made simple #include <stdio.h> #include <stdlib.h> int main() { int num, *arr, i; scanf("%d", &num); arr = (int*) malloc(num * sizeof(int)); for(i = 0; i < num; i++) { scanf("%d", arr + i); } /* Write the logic to reverse the array. */ int* left_ptr = arr; int* right_ptr; int temp; for(i = 0; i < num; i++) { if(i == num - 1) { right_ptr = (arr + i); } } while(left_ptr < right_ptr) { temp = *right_ptr; *right_ptr = *left_ptr; *left_ptr = temp; right_ptr--; left_ptr++; } for(i = 0; i < num; i++) { printf("%d ", *(arr + i)); } free(arr); return 0; }
Output:
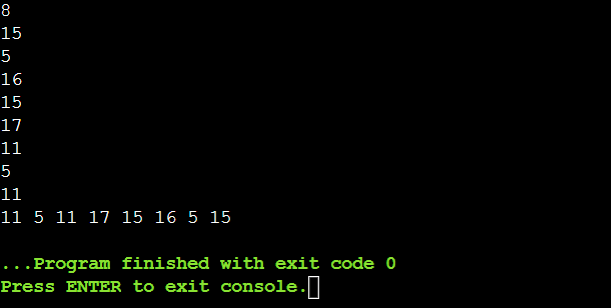
Conclusion
So, in this way we’ve learned how to code the array reversal program with an explanation. If you’ve any doubts then please feel free to contact us. We’ll reach you as soon as possible.
Thanks and Happy Coding :)
Also Read:
- Adams Charity Adam Decides to be generous.
- A Rural Library Created Lending Facility for only 100 Books.
- Dynamic Array in C Hackerrank Solution.
- Time Conversion Hackerrank Solution.
- Class Hackerrank Solution in C++.