Hi Friends, today in this tutorial we’ll learn how to solve Dynamic Array in C Hackerrank Solution With Explanation. If you’re wondering how to solve the Dynamic Array Hackerrank problem in C, then you’re at the right place. Here you’ll get all hackerrank solutions with an explanation. But, before getting started we’ll first let you know about hacker rank.
Table of Contents
What is Hackerrank?
Hackerrank is a tech company that mainly focuses on competitive programming challenges for students, companies, and consumers. Hackerrank was founded by two founders Vivek Ravishankar and Harishankar Karunanidhi in the year 2012. Its headquarters are situated in California, United States.
With the help of this platform students as well as professionals prepare themselves for the placements by practicing the coding problems. The questions that hacker rank contains are asked in the interview rounds of many companies.
So let us code Dynamic Array in C Hackkerank Solution with Explanation.
Dynamic Array in C Hackerrank Solution
Generally, Dynamic Array in C is represented using pointers with the allocated memory on which they point on. So you can use malloc or calloc functions from the stdlib.h library.
Problem Statement:
Snow Howler is the librarian at the central library of the city of HuskyLand. He must handle requests which come in the following forms:
1 x y: Insert a book with y pages at the end of the xth shelf.
2 x y: Print the number of pages in the yth book on the xth shelf.
3 x: Print the number of books on the xth shelf.
Snow Howler has got an assistant, Oshie provided by the department of education. Although inexperienced, Oshie can handle all of the queries of types 2 and 3.
Help Snow Howler deal with all the queries of type 1.
Task:
Snow Howler is the librarian at the central library of the city of HuskyLand. He must handle requests which come in the following forms:
1 x y: Insert a book with y pages at the end of the xth shelf.
2 x y: Print the number of pages in the yth book on the xth shelf.
3 x: Print the number of books on the xth shelf.
Input Format:
In the first line integer total_number_of_shelves i.e the number of shelves in the library is given. Similarly in the second line integer total_number_of_queries i.e, the number of requests is given. Each total_number_of_queries contains a request in one of the three specified formats.
Constraints:
- 1 <= total_number_of_shelves <= 10^5.
- 1 <= total_number_of_queries <= 10^5.
- For the queries of the 2nd type, it is guaranteed that the book is present on the xth shelf at the yth index.
- 0 <= x <total_number_of_shelves.
- Numbers for both books and shelves are starting from 0.
- The maximum no of books per shelf <= 1100.
Output Format:
Write a logic for all the requests of type 1. And the logic of types 2 and 3 is already provided.
Sample Input 0:
5 5 1 0 15 1 0 20 1 2 78 2 2 0 3 0
Sample Output 0:
78 2
Explanation 0:
There is a total of 5 shelves and 5 requests. 1st place the 15-page book at the end of the shelf 0. 2nd Place the 20-page book at the end of the shelf 0. 3rd Place a 78-page book at the end of the shelf 2. 4th The no of pages in the 0th book on the 2nd shelf is 78. 5th the number of books on the 0th shelf is 2.
Dynamic Array in C Hackerrank Solution
//Learnprogramo - programming made simple /*Dynamic Array in C - Hacker Rank Solution*/ #include <stdio.h> #include <stdlib.h> /* * This stores the total number of books in each shelf. */ int* total_number_of_books; /* * This stores the total number of pages in each book of each shelf. * The rows represent the shelves and the columns represent the books. */ int** total_number_of_pages; int main() { int total_number_of_shelves; scanf("%d", &total_number_of_shelves); total_number_of_books = calloc(total_number_of_shelves, sizeof(int)); int total_number_of_queries; scanf("%d", &total_number_of_queries); total_number_of_pages = malloc(total_number_of_shelves * sizeof(int *)); for (int i = 0; i < total_number_of_shelves; i++) { total_number_of_pages[i] = calloc(1100, sizeof(int)); } while (total_number_of_queries--) { int type_of_query; scanf("%d", &type_of_query); if (type_of_query == 1) { /* * Process the query of first type here. */ int shelf, pages; scanf("%d %d", &shelf, &pages); total_number_of_books[shelf]++; int *book = total_number_of_pages[shelf]; while (*book != 0) book++; *book = pages; } else if (type_of_query == 2) { int x, y; scanf("%d %d", &x, &y); printf("%d\n", *(*(total_number_of_pages + x) + y)); } else { int x; scanf("%d", &x); printf("%d\n", *(total_number_of_books + x)); } } if (total_number_of_books) { free(total_number_of_books); } for (int i = 0; i < total_number_of_shelves; i++) { if (*(total_number_of_pages + i)) { free(*(total_number_of_pages + i)); } } if (total_number_of_pages) { free(total_number_of_pages); } return 0; }
Output:
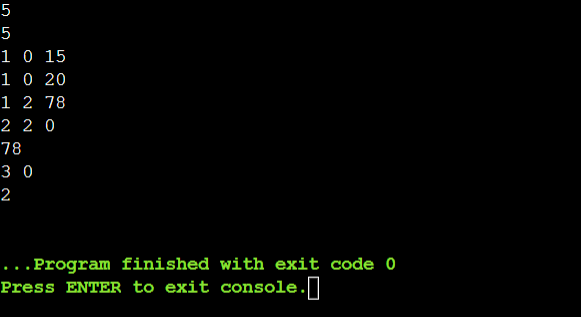
Conclusion
So, in this way we’ve learned how to code dynamic array in c hackerrank solution with explanation. If you’ve any doubts then please feel free to contact us. We’ll reach you as soon as possible.
Thanks and Happy Coding 🙂
Also Read:
- Adams Charity Adam Decides to be generous.
- A Rural Library Created Lending Facility for only 100 Books.